The Role of MATLAB Genetic Algorithms in Complex System Modeling: Strategies and Case Studies
发布时间: 2024-09-15 04:25:20 阅读量: 54 订阅数: 22 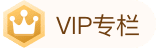
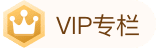
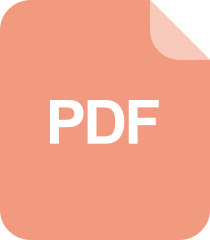
Hands-On Genetic Algorithms with Python: Applying genetic algori
# Step-by-Step MATLAB Genetic Algorithm Implementation
Genetic Algorithms (GA) are heuristic search algorithms for solving optimization and search problems, inspired by the theory of natural selection and biological evolution. MATLAB, as a widely-used mathematical computing and programming environment, provides a Genetic Algorithm Toolbox for more efficient optimization tasks in engineering and scientific computations.
This chapter aims to introduce the basics of using Genetic Algorithms in MATLAB to readers, including its working principles, toolbox applications, and some simple case studies, laying the foundation for further in-depth learning in subsequent chapters.
```matlab
% Example: Using MATLAB's built-in ga function for simple optimization
% Define a simple fitness function, such as maximizing the objective function f(x) = x^2
fitnessFcn = @(x) -x.^2;
% Range of optimization variables
lb = -50;
ub = 50;
% Perform optimization
[x,fval] = ga(fitnessFcn,1,[],[],[],[],lb,ub);
```
In the above MATLAB code example, we define a simple fitness function and set the variable range, then call the `ga` function for optimization calculation. The result `x` is the variable value that maximizes the function value within the given range, and `fval` is the corresponding fitness function value.
From this example, we can see that the MATLAB Genetic Algorithm Toolbox is very intuitive and convenient to use, making MATLAB an important tool for research and application of genetic algorithms.
# 2. Theoretical Basis of Genetic Algorithms
### 2.1 Basic Principles of Genetic Algorithms
#### 2.1.1 Natural Selection and Genetic Mechanisms
The inspiration for Genetic Algorithms comes from Darwin's theory of natural selection. This theory suggests that individuals better adapted to their environment have higher chances of survival and reproduction, and through generations of inheritance and variation, populations gradually adapt to their environment. In Genetic Algorithms, this process is abstracted into "populations," "individuals," and "fitness functions" within a computer program.
In the algorithm, each solution is called an "individual," represented by a set of parameters (called "chromosomes"). An individual's "fitness" is evaluated by a fitness function, which is designed based on the optimization problem and can quantify an individual's ability to solve the problem. In each generation, individuals are selected to participate in the production of the next generation based on fitness, and new individuals, i.e., new solutions, are generated through genetic operations such as "crossover" (similar to biological hybridization) and "mutation" (similar to gene mutation). This process iterates until a preset termination condition is reached.
#### 2.1.2 Main Components of the Algorithm
The main components of Genetic Algorithms include:
- **Population**: A set of individuals, each representing a potential solution to the problem.
- **Individual**: A set of parameters representing a single potential solution.
- **Chromosome**: The parameter encoding within an individual, usually in binary code.
- **Fitness Function**: A function that evaluates an individual's ability to adapt to the environment.
- **Selection**: The process of selecting individuals based on the fitness function to participate in reproduction in the next generation.
- **Crossover**: The process of combining the chromosomes of two individuals to produce offspring.
- **Mutation**: The process of randomly changing individual characteristics on the chromosome.
### 2.2 Mathematical Model of Genetic Algorithms
#### 2.2.1 Encoding and Fitness Function
**Encoding** is a key step in Genetic Algorithms, converting solution domains within a problem into a form that the algorithm can process. Binary strings, real-valued strings, or other encoding methods are commonly used to represent the chromosomes of individuals. Appropriate encoding methods can greatly affect the algorithm's search efficiency and the quality of solutions.
**Fitness Function** is the standard for evaluating the chromosome's ability to adapt to the environment and must be designed based on the specific problem. For optimization problems, the fitness function is usually the opposite of the objective function's performance. For example, the smaller the objective function value of a minimization problem, the larger the fitness function value.
The code block can demonstrate the implementation of a simple Genetic Algorithm fitness function, such as:
```matlab
% MATLAB code block: Fitness function example
function fitness = fitness_function(x)
% Assuming we have a simple objective function f(x) = -x^2 + 4x
% The fitness function is the opposite of this objective function, since MATLAB's optimization toolbox
% by default looks for the minimum value, we need to convert it to a high fitness value
target_function = -x^2 + 4*x;
fitness = -target_function; % Take the opposite as the fitness value
end
```
#### 2.2.2 Selection, Crossover, and Mutation Operations
**Selection***mon selection methods include roulette wheel selection, tournament selection, etc. Roulette wheel selection decides the probability of an individual being selected based on its fitness proportion to the total fitness, so individuals with higher fitness have a greater chance of being selected.
**Crossover** operation simulates the hybridization process in biological genetics. It combines two chromosomes according to certain rules to produce two new chromosomes. A commonly used crossover operation is single-point crossover, which randomly selects a crossover point and then exchanges the parts of the chromosomes after this point between two individuals.
**Mutation** operation simulates the process of gene mutation. At certain positions on some chromosomes, gene values are randomly changed (e.g., binary encoding 0 becomes 1) to maintain the diversity of the population.
The next code block can demonstrate simple crossover and mutation operations:
```matlab
% MATLAB code block: Crossover operation example
function [child1, child2] = crossover(parent1, parent2)
crossover_point = randi([1, length(parent1)-1]); % Random crossover point
child1 = [parent1(1:crossover_point), parent2(crossover_point+1:end)];
child2 = [parent2(1:crossover_point), parent1(crossover_point+1:end)];
end
% MATLAB code block: Mutation operation example
function mutated_child = mutation(child, mutation_rate)
mutated_child = child;
for i = 1:length(child)
if rand < mutation_rate
mutated_child(i) = 1 - mutated_child(i); % Binary mutation
end
end
end
```
### 2.3 Convergence Analysis of Genetic Algorithms
#### 2.3.1 Theoretical Convergence Conditions
As a probabilistic search algorithm, the convergence of Genetic Algorithms is one of the key focuses of theoretical research. Whether a Genetic Algorithm can converge to the global optimum solution depends on the design parameters and operational strategies of the algorithm. Theoretically, if the selection pressure in the algorithm is sufficiently large and the crossover and mutation operations can explore enough of the search space, then the algorithm has a probability of converging to the optimal solution.
#### 2.3.2 Performance Evaluation Metrics of the Algorithm
The performance evaluation of Genetic Algorithms usually depends on the following metrics:
- **Convergence Speed**: The number of iterations required for the algorithm to reach a certain level of fitness.
- **Convergence Quality**: The probability that the algorithm finds the optimal solution or an approximate optimal solution.
- **Stability**: The consistency of the algorithm's results when run repeatedly under the same conditions.
- **Robustness**: The algorithm's ability to adapt to changes in the problem.
By analyzing these metrics, we can help improve the algorithm design and enhance the practicality and efficiency of Genetic Algorithms. The following table shows a comparison of Genetic Algorithm performance under different parameter settings:
| Parameter Setting | Convergence Speed | Convergence Quality | Stability | Robustness |
|-------------------|------------------|--------------------|-----------|------------|
| Parameter Combination A | Faster | Higher | Good | Strong |
| Parameter Combination B | Slower | Lower | Poor | Weak |
| Parameter Combination C | Medium | Medium | Medium | Medium |
From the table, we can clearly see the impact of different parameter settings on the performance of Genetic Algorithms, guiding us in optimizing the algorithm.
# 3. Using MATLAB Genetic Algorithm Toolbox
In the practice of optimizing complex problems, the MATLAB Genetic Algorithm Toolbox provides users with a comprehensive set of tools and functions to achieve efficient design and development of Genetic Algorithms. This chapter will delve into the specific methods of using the MATLAB Genetic Algorithm Toolbox, including toolbox installation and configuration, basic operations and function interpretation, as well as advanced applications and customization strategies.
## 3.1 Toolbox Installation and Configuration
### 3.1.1 Installation Steps and Environment Setup
Installing the MATLAB Genetic Algorithm Toolbox usually involves a few simple steps. First, ensure that your system has MATLAB installed. Open MATLAB and use the toolbox manager to install the GA Toolbox. In the command window, enter:
```matlab
>> toolbox install -setup
```
After executing, follow the prompts of the installation wizard to install. After installation, you need to set up the path in the MATLAB environment so that the toolbox can be correctly recognized and used. Through the command:
```matlab
>> addpath('path/to/GA Toolbox')
```
Replace "path/to/GA Toolbox" with the actual file path. After setting the path, restart MATLAB to ensure that the settings take effect.
### 3.1.2 Toolbox Function Overview
After installing and configuring the MATLAB Genetic Algorithm Toolbox, users can access a complete set of functions and commands related to Genetic Algorithms. These functions include but are not limited to:
- `ga`: The basic Genetic Algorithm function.
- `gamultiobj`: A Genetic Algorithm function for multi-objective optimization problems.
- `gacompany`: A fu
0
0
相关推荐







