MATLAB Genetic Algorithm Coding and Operations: In-depth Analysis of Crossover and Mutation
发布时间: 2024-09-15 04:22:30 阅读量: 40 订阅数: 38 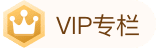
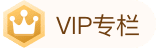
# Fundamental Concepts and Principles of Genetic Algorithms
Genetic algorithms are a class of search heuristic algorithms that simulate the principles of natural selection and genetics. They belong to the category of evolutionary algorithms and are widely applied in various optimization and search problems. Genetic algorithms typically consist of concepts such as populations, individuals, genes, and fitness, with the core idea being the iterative improvement of a set of candidate solutions through the "survival of the fittest" mechanism of natural selection. Each individual represents a potential solution in the problem space, while a population is a collection of these individuals.
## 1.1 Basic Principles of Genetic Algorithms
The basic principles of genetic algorithms can be understood through the following steps:
- Initialization: Randomly generate a set of individuals to form an initial population.
- Fitness Evaluation: Calculate the fitness of each individual in the population based on a predefined fitness function.
- Selection: Select individuals based on their fitness, with higher fitness individuals having a greater chance of being chosen for reproduction.
- Crossover: Combine two parent individuals through crossover (also known as hybridization or recombination) to produce offspring.
- Mutation: Randomly alter the genes of individuals with a certain probability to increase the diversity of the population.
- Replacement: Replace some individuals in the current population with the offspring, completing a generation of evolution.
- Termination Condition: Repeat the above steps until a termination condition is met, such as reaching a predetermined number of generations or a fitness standard.
## 1.2 Key Characteristics of Genetic Algorithms
- Parallelism: Genetic algorithms search the solution space in parallel through populations, effectively avoiding local optima and possessing the ability for global search.
- Guidance: Through the fitness function, the algorithm can guide the search towards better solutions.
- Adaptability: The iterative process of the algorithm can adaptively adjust parameters, such as selection, crossover, and mutation probabilities, to meet the needs of different problems.
With continuous exploration and practice, the theory and applications of genetic algorithms have formed a relatively comprehensive theoretical system and operational framework, showing great potential and wide applicability in solving practical problems.
# 2. Genetic Algorithm Framework and Components in MATLAB
MATLAB provides a powerful genetic algorithm toolbox, allowing users to easily build and execute genetic algorithms. This chapter will delve into the genetic algorithm framework and components in MATLAB, including data structures, function libraries, parameter settings, and algorithm configurations.
## 2.1 Data Structures in Genetic Algorithms
In genetic algorithms, a population is a collection of solutions, and each generation of the population is updated through selection, crossover, and mutation operations. The design of data structures significantly affects algorithm performance.
### 2.1.1 Population Representation
In MATLAB, populations are typically represented by matrices, where each row represents an individual, and each column represents a variable. This representation method is convenient for implementing genetic operations and can easily adapt to different problem scales.
```matlab
% Example: Initialize population
popSize = 100; % Population size
nVars = 5; % Number of variables
varMin = -10; % Minimum value of variables
varMax = 10; % Maximum value of variables
% Use MATLAB built-in function for population initialization
population = varMin + (varMax - varMin) * rand(popSize, nVars);
```
### 2.1.2 Design of Fitness Functions
The fitness function is the standard for evaluating the performance of individuals in genetic algorithms. Designing a good fitness function is crucial to the success of the algorithm. It should accurately reflect the objectives and constraints of the problem.
```matlab
% Example: Define a simple fitness function
function fitness = simpleFitnessFunction(x)
% Assuming our objective function to minimize is f(x) = sum(x.^2)
fitness = sum(x.^2);
end
% Call the fitness function to evaluate the population in MATLAB
fitnessValues = arrayfun(@(x) simpleFitnessFunction(x), population);
```
## 2.2 MATLAB Genetic Algorithm Function Library
MATLAB offers a rich library of genetic algorithm functions, which mainly include selection functions, crossover functions, and mutation functions. These are the basis for implementing genetic algorithms.
### 2.2.1 Selection Functions
Selection functions aim to choose individuals for the next generation based on their fitness. In MATLAB, various strategies such as roulette wheel selection and tournament selection can be chosen.
```matlab
% Example: Use roulette wheel selection to select a population
% Assuming fitnessValues are already sorted in ascending order
sumFit = sum(fitnessValues);
probs = fitnessValues / sumFit;
cumProbs = cumsum(probs);
selectedIndices = find(rand(popSize, 1) <= cumProbs);
selectedIndividuals = population(selectedIndices, :);
```
### 2.2.2 Crossover Functions
Crossover functions are responsible for generating a new population. MATLAB's crossover functions can implement various crossover strategies, including single-point crossover and multi-point crossover.
```matlab
% Example: Single-point crossover function implementation
function [child1, child2] = singlePointCrossover(parent1, parent2, crossoverRate)
if rand() > crossoverRate
child1 = parent1;
child2 = parent2;
else
% Randomly select a crossover point
crossoverPoint = randi(length(parent1) - 1);
child1 = [parent1(1:crossoverPoint), parent2(crossoverPoint+1:end)];
child2 = [parent2(1:crossoverPoint), parent1(crossoverPoint+1:end)];
end
end
```
### 2.2.3 Mutation Functions
Mutation functions are the primary means of introducing new genetic information in genetic algorithms. MATLAB supports various mutation strategies, including bit mutation and uniform mutation.
```matlab
% Example: Uniform mutation function implementation
function mutatedIndividual = uniformMutation(individual, mutationRate, varMin, varMax)
mutatedIndividual = individual;
for i = 1:length(individual)
if rand() < mutationRate
mutatedIndividual(i) = varMin + (varMax - varMin) * rand();
end
end
end
```
## 2.3 Parameter Settings and Algorithm Configuration
Properly setting parameters for genetic algorithms is key to obtaining excellent solutions. Parameters include population size, generations of evolution, crossover rate, mutation rate, and selection strategies.
### 2.3.1 Population Size and Generations of Evolution
Population size and generations of evolution are two basic parameters of genetic algorithms. They affect the algorithm's search ability and computational cost.
### 2.3.2 Adjustment of Crossover and Mutation Rates
Crossover and mutation rates are two important parameters controlling the algorithm's search direction. Typically, the crossover rate should be set higher, while the mutation rate should be set lower.
### 2.3.3 Customizing Selection Strategies
Selection strategies determine which individuals have the chance to participate in the reproduction of the next generation. MATLAB allows users to customize selection strategies based on actual problems to optimize algorithm performance.
The MATLAB genetic algorithm toolbox offers extensive parameter adjustment options. Users can configure settings appropriately based on specific problems and experience. In the implementation process of genetic algorithms, choosing suitable parameters and strategies significantly affects algorithm performance.
In the next chapter, we will explore the implementation and optimization of crossover operations in genetic algorithms, delving into the different classifications of crossover operations, the code implementation of crossover operations in MATLAB, and case studies of crossover operations.
# 3. Implementation and Optimization of Crossover Operations
## 3.1 Basic Principles and Classifications of Crossover Operations
### 3.1.1 Single-point Crossover and Multi-point Crossover
Crossover operations simulate the hybridization phenomenon in biological evolution within genetic algorithms. It allows for the production of new offspring chromosomes from two parent chromosomes, providing the genetic algorithm with the ability to explore the solution space. In single-point crossover, a random crossover point is selected, and the parent chromosomes exchange their chromosomal segments at this point, resulting in offspring. This crossover method is simple and efficient but may lead to a decrease in population diversity.
In contrast, multi-point crossover allows for more than one crossover point, making the exchange of chromosomal segments between parents more frequent and thus increasing the genetic diversity of the offspring. Multi-point crossover may perform better than single-point crossover in solving certain problems, but it also has a higher computational complexity.
### 3.1.2 Uniform Crossover and Order Crossover
Uniform crossover does not rely on specific crossover points but independently selects genes from each gene locus of the two parents with a certain probability. This ensures diversity at the gene level and avoids certain gene loci from becoming fixed. Uniform crossover is particularly suitable for problems where genes are mutually independent.
Order crossover is based on the relative order of genes in the parent chromosomes for exchange. This method preserves the relative position information of the genes in the parent chromosomes, avoiding unreasonable gene combinations. It is suitable for problems where genes have order-relatedness.
## 3.2 Code Implementation of Crossover Operations in MATLAB
### 3.2.1 Steps to Write Crossover Functions
To implement crossover operations in MATLAB, a crossover function must first be defined. This function takes the parent chromosomes as input and generates offspring. Here is a simple implementation of a single-point crossover function:
```matlab
function [child1, child2] = singlePointCrossover(parent1, parent2)
% Ensure both parents have the same length
if length(parent1) ~= length(parent2)
error('Parent chromosome lengths must be the same');
end
% Randomly determine the crossover point
crossoverPoint = randi(length(parent1) - 1);
% Produce offspring
child1 = [parent1
```
0
0
相关推荐
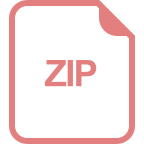
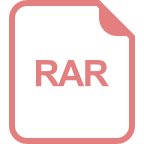
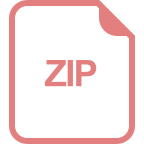
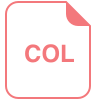
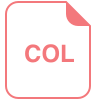
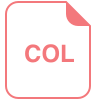
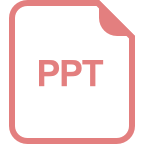
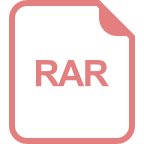
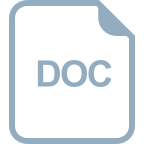