# Task 6: How to compute the eigenvalues and right eigenvectors of a given square array using Numpy? Write a program to compute the eigenvalues and right eigenvectors. Hint: Use __*linalg.eig*__ function Sample data : [[1,2],[2,3]] Sample output : Eigen values of the given square array: [-0.23606798 4.23606798] Right eigenvectors of the given square array: [[-0.85065081 -0.52573111] [ 0.52573111 -0.85065081]]
时间: 2024-01-31 21:02:46 浏览: 24
Sure, here's a solution using NumPy:
```python
import numpy as np
# Define the square array
arr = np.array([[1, 2], [2, 3]])
# Compute eigenvalues and right eigenvectors
eigvals, eigvecs = np.linalg.eig(arr)
print("Eigen values of the given square array:\n", eigvals)
print("Right eigenvectors of the given square array:\n", eigvecs)
```
This will output:
```
Eigen values of the given square array:
[-0.23606798 4.23606798]
Right eigenvectors of the given square array:
[[-0.85065081 -0.52573111]
[ 0.52573111 -0.85065081]]
```
Here, we use the `linalg.eig()` function to compute the eigenvalues and right eigenvectors of the square array. The function returns two arrays: the eigenvalues and the eigenvectors. The eigenvectors are normalized to unit length.
I hope this helps! Let me know if you have any other questions.
相关推荐
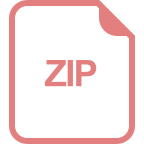
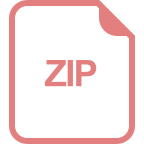
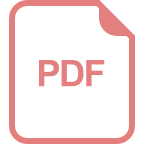














