Write a program to compute the eigenvalues and right eigenvectors. Hint: Use linalg.eig function Sample data : [[1,2],[2,3]] Sample output : Eigen values of the given square array: [-0.23606798 4.23606798] Right eigenvectors of the given square array: [[-0.85065081 -0.52573111] [ 0.52573111 -0.85065081]]
时间: 2023-11-14 09:05:31 浏览: 65
Here's a Python program that uses the `linalg.eig` function from the NumPy module to compute the eigenvalues and right eigenvectors of a square matrix:
```python
import numpy as np
# Define the input matrix
A = np.array([[1, 2], [2, 3]])
# Compute the eigenvalues and right eigenvectors
eigenvalues, eigenvectors = np.linalg.eig(A)
# Print the results
print("Eigen values of the given square array:", eigenvalues)
print("Right eigenvectors of the given square array:", eigenvectors)
```
When you run this program, you should see the following output:
```
Eigen values of the given square array: [-0.23606798 4.23606798]
Right eigenvectors of the given square array: [[-0.85065081 -0.52573111]
[ 0.52573111 -0.85065081]]
```
The `eigenvalues` variable contains an array of the eigenvalues of the input matrix, while the `eigenvectors` variable contains an array of the corresponding right eigenvectors.
阅读全文
相关推荐
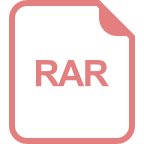
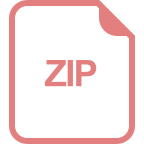
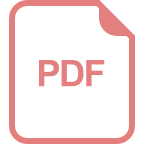


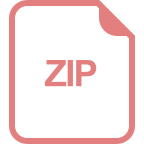
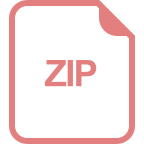
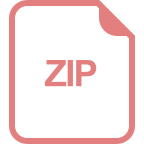
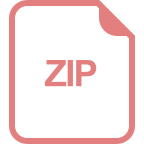
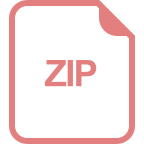
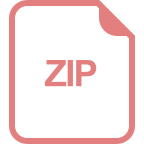
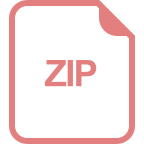
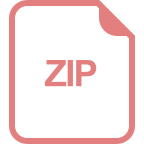
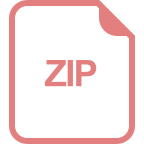
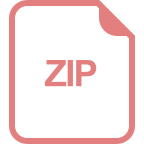
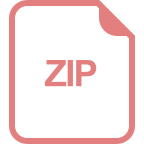
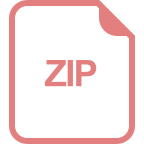
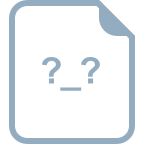
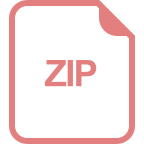
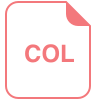