Dependency Analysis for NumPy Installation: Unveiling the Principles Behind Installing NumPy
发布时间: 2024-09-15 15:04:41 阅读量: 23 订阅数: 33 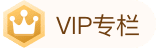
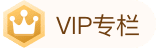
# NumPy Installation Dependency Analysis: Unveiling the Principles Behind NumPy Setup
## 1. Introduction to NumPy
NumPy, short for Numerical Python, is a library for the Python programming language, which is used for scientific computing. It offers support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays. NumPy is widely utilized in the fields of data analysis, machine learning, and image processing.
The main features of NumPy include:
- **Multi-dimensional array objects**: NumPy provides an array object called `ndarray` that can store n-dimensional arrays of data of different types.
- **Matrix operations**: NumPy comes with an extensive set of matrix operation functions, including addition, subtraction, multiplication, transposition, and inversion.
- **Linear algebra**: NumPy provides functions for solving systems of linear equations, calculating eigenvalues and eigenvectors.
- **Fourier transform**: NumPy offers functions for performing Fast Fourier Transform (FFT).
## 2. NumPy Installation Dependency Analysis
### 2.1 Python Environment Dependencies
NumPy installation relies on a Python environment and must meet the following conditions:
#### 2.1.1 Python Version Requirements
NumPy supports Python versions 3.6 and above. It is recommended to use the latest version of Python for the best compatibility and performance.
#### 2.1.2 Python Package Management Tools
Python package managers are used to install and manage Python packages, ***monly used package managers are:
- **pip**: Python's official package manager that installs and manages packages via the command line.
- **conda**: A package manager included with the Anaconda distribution that provides more comprehensive package management features and environment management.
### 2.2 NumPy Dependent Packages
NumPy relies on several core packages and optional packages:
#### 2.2.1 NumPy Core Dependent Packages
The core dependent packages for NumPy include:
- **numpy-base**: The foundational package of NumPy, providing basic data types and operations.
- **numpy-core**: Core algorithms and functions of NumPy.
- **numpy-f2py**: A tool for compiling Fortran code into Python extensions.
#### 2.2.2 Optional Dependent Packages
NumPy also supports optional dependent packages to extend its functionality:
- **scipy**: A library for scientific and technical computing, offering advanced mathematical functions and statistical analysis tools.
- **pandas**: A data analysis and manipulation library that provides data structures such as data frames and time series.
- **matplotlib**: A data visualization library that offers various plotting and charting functionalities.
**Code Block:**
```python
import numpy as np
# Creating a NumPy array
arr = np.array([1, 2, 3, 4, 5])
# Printing the array
print(arr)
```
**Logical Analysis:**
This code block demonstrates how to create and print an array using NumPy. The statement `import numpy as np` imports the NumPy library and assigns it the alias `np`. The statement `np.array([1, 2, 3, 4, 5])` creates a one-dimensional NumPy array containing the elements [1, 2, 3, 4, 5]. The statement `print(arr)` prints the array.
**Parameter Explanation:**
- `np.array()`: Creates a NumPy array.
- `[1, 2, 3, 4, 5]`: The list of elements for the array to be created.
- `print()`: Prints the array.
## 3. NumPy Installation in Practice
### 3.1 Installation via Pip
Pip is a Python package manager that is used to install and manage Python packages, including NumPy. There are two ways to install NumPy using Pip: command line installation and IDE installation.
#### 3.1.1 Command Line Installation
Enter the following command in the command line to install:
```
pip install numpy
```
After executing this command, Pip will automatically download and install NumPy along with its dependencies.
#### 3.1.2 IDE Installation
In an IDE like PyCharm, you can install NumPy by following these steps:
1. Open the IDE, click the "File" menu, and select "Settings".
2. In the "Settings" window, choose the "Project" tab, and then click "Python Interpreter".
3. In the "Python Interpreter" window, click the "+" button, select "Install Packages".
4. In the "Install Packages" window, search for "NumPy", and then click "Install Package".
The IDE will automatically download and install NumPy and its dependencies.
### 3.2 Installation via Conda
Conda is part of the Anaconda distribution, and it is a package and environment manager for Python packages and environments. There are two ways to install NumPy using Conda: command line installation and Anaconda Navigator installation.
#### 3.2.1 Command Line Installation
Enter the following command in the command line to install:
```
conda install numpy
```
After executing this command, Conda will automatically download and install NumPy along with its dependencies.
#### 3.2.2 Anaconda Navigator Installation
In Anaconda Navigator, you can install NumPy by following these steps:
1. Open Anaconda Navigator.
2. In the "Environments" tab, select the environment where you want to install NumPy.
3. Click on the "Packages" tab, search for "NumPy", and then click "Install".
Anaconda Navigator will automatically download and install NumPy and its dependencies.
### Code Example: Pip Command Line Installation
```
# Using Pip command line to install NumPy
pip install numpy
# Checking if NumPy is installed
import numpy
print(numpy.__version__)
```
**Logical Analysis:**
1. The `pip install numpy` command installs NumPy.
2. `import numpy` imports the NumPy module.
3. `print(numpy.__version__)` prints the NumPy version.
**Parameter Explanation:**
* `pip install numpy`: Installs the NumPy package.
* `import numpy`: Imports the NumPy module.
* `print(numpy.__version__)`: Prints the NumPy version.
## ***mon Issues During NumPy Installation
### 4.1 Failed Installation of Dependent Packages
#### 4.1.1 Incompatible Versions of Dependent Packages
While installing NumPy, you may encounter issues with incompatible versions of dependent packages. For instance, NumPy might require a specific version of a package, but an incompatible version is already installed on the system.
**Solution:**
* Check the version requirements for the dependent package.
* Uninstall the old version of the dependent package.
* Install a compatible version of the dependent package.
#### 4.1.2 Incorrect System Environment Variable Configuration
If the system environment variables are not configured correctly, it may also lead to a failed installation of dependent packages. For example, the PATH environment variable might not be set correctly, causing the inability to find the installation path of the dependent packages.
**Solution:**
* Check if the PATH environment variable contains the installation path of the dependent package.
* If not, add the installation path of the dependent package to the PATH environment variable.
### 4.2 Failed Installation of NumPy
#### 4.2.1 Compilation Environment Issues
NumPy installation requires a C compiler. If a C compiler is not installed on the system or the installed version is too old, it may result in a failed installation of NumPy.
**Solution:**
* Install a compatible C compiler.
* Update the C compiler to a newer version.
#### 4.2.2 Permission Issues
If the user does not have sufficient permissions, it may also lead to a failed installation of NumPy. For example, the user may not have the permission to write to the installation directory.
**Solution:**
* Use a user with sufficient permissions to install NumPy.
* Change the permissions of the installation directory.
## 5. Optimization of NumPy Installation
After completing the basic installation of NumPy, we can optimize the installation process to enhance efficiency and performance. This chapter will introduce two optimization methods: managing dependent packages and optimizing NumPy installation.
### 5.1 Dependent Package Management
#### 5.1.1 Using Dependent Package Managers
Dependent package managers can automatically manage the installation and update of dependent packages, ***monly used dependent package managers include:
- pip: Python's official package manager
- conda: A package manager included with the Anaconda distribution
**Using pip to install dependent packages:**
```bash
pip install numpy
```
**Using conda to install dependent packages:**
```bash
conda install numpy
```
#### 5.1.2 Periodically Updating Dependent Packages
Regular updates of dependent packages can ensure that NumPy is using the latest versions, thereby benefiting from the latest features and fixes. You can use the following commands to update dependent packages:
**Using pip to update dependent packages:**
```bash
pip install --upgrade numpy
```
**Using conda to update dependent packages:**
```bash
conda update numpy
```
### 5.2 NumPy Installation Optimization
#### 5.2.1 Using Binary Installation Packages
Binary installation packages contain pre-compiled code and can avoid the compilation process during installation. This can significantly reduce installation time, especially on low-performance computers.
**Using pip to install binary installation packages:**
```bash
pip install numpy --prefer-binary
```
**Using conda to install binary installation packages:**
```bash
conda install numpy -c conda-forge
```
#### 5.2.2 Compilation Optimization
If binary installation packages are not an option, ***pilation optimization options include:
- **-march=native**: Uses the instruction set compatible with the current CPU architecture
- **-O3**: Enables the highest level of optimization
- **-j**: Specifies the number of parallel compilation threads
**Using compilation optimization to install NumPy:**
```bash
pip install numpy --install-option="--march=native --O3 --j=4"
```
## 6. Advanced NumPy Installation
### 6.1 Installation in a Virtual Environment
A virtual environment is a self-contained Python environment that allows users to install and manage different versions of Python packages without affecting the system-wide Python installation. Virtual environments are particularly useful for projects that require a specific version of NumPy or its dependencies.
#### 6.1.1 Creating a Virtual Environment
Use the following command to create a virtual environment:
```bash
python -m venv venv_name
```
Here, `venv_name` is the name of the virtual environment.
#### 6.1.2 Installing NumPy in a Virtual Environment
After activating the virtual environment, you can install NumPy using the following command:
```bash
pip install numpy
```
### 6.2 Installation via Docker
Docker is a containerization platform that enables users to run applications in isolated environments. Docker installation of NumPy is ideal for projects that need consistent execution of NumPy across different environments.
#### 6.2.1 Creating a Docker Image
Use the following command to create a Docker image:
```bash
docker build -t numpy_image .
```
Here, the dot (`.`) indicates that the current directory contains the `Dockerfile`.
#### 6.2.2 Running a Docker Container
Use the following command to run a Docker container:
```bash
docker run -it --rm numpy_image python
```
This will启动一个交互式的Python会话在容器中,用户可以在其中使用NumPy。
0
0
相关推荐








