The Application of Transposing Matrices in Machine Learning: From Theory to Practice, Unveiling 5 Key Scenarios
发布时间: 2024-09-13 21:46:02 阅读量: 31 订阅数: 26 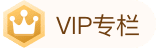
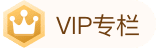
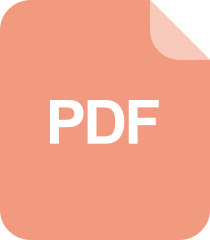
Software Architecture for Big Data and the Cloud
# 1. Theoretical Foundation of Transposed Matrices**
A transposed matrix is one that switches its rows and columns. For an m×n matrix A, its transposed matrix AT is an n×m matrix, where the element in the i-th row and j-th column of AT equals the element in the j-th row and i-th column of A.
Transposed matrices have the following properties:
- (AB)T = BTAT
- (AT)T = A
- (A+B)T = AT+BT
- (kA)T = kAT, where k is a scalar
# 2.1 Optimization of Matrix Operations
### 2.1.1 Application of Transposed Matrices in Matrix Multiplication
In machine learning, matrix multiplication is a common operation used for calculating model weights, feature transformations, and prediction results. Transposed matrices can optimize the computational efficiency of matrix multiplication.
Consider two matrices A and B, where A has dimensions m x n and B has dimensions n x p. The computational complexity of standard matrix multiplication is O(mnp).
By transposing matrix B and changing its dimensions to p x n, matrix multiplication can be optimized to A * B^T. In this case, the computational complexity becomes O(mn + np), which is significantly more efficient when m and n are much larger than p.
**Code Block:**
```python
import numpy as np
# Original matrices A and B
A = np.array([[1, 2, 3], [4, 5, 6]])
B = np.array([[7, 8], [9, 10], [11, 12]])
# Transposed matrix B
B_T = np.transpose(B)
# Matrix multiplication
C = A @ B_T
print(C)
```
**Logical Analysis:**
* `np.transpose(B)`: Transposes matrix B, changing its dimensions from n x p to p x n.
* `A @ B_T`: Performs matrix multiplication, optimizing the computational complexity to O(mn + np).
### 2.1.2 Application of Transposed Matrices in Feature Engineering
Feature engineering is a crucial step in machine learning, used for extracting and transforming useful features from raw data. Transposed matrices can simplify certain operations in feature engineering.
For instance, in one-hot encoding, categorical features are converted into binary vectors. The conventional method requires逐行转换, with a computational complexity of O(mn), where m is the number of samples and n is the number of categories.
By transposing the raw data and changing its dimensions to n x m, and then performing one-hot encoding, the computational complexity is optimized to O(nm).
**Code Block:**
```python
import pandas as pd
# Original data
data = pd.DataFrame({
'category': ['A', 'B', 'C', 'A', 'B'],
'value': [1, 2, 3, 4, 5]
})
# Transposed data
data_T = data.T
# One-hot encoding
data_onehot = pd.get_dummies(data_T)
print(data_onehot)
```
**Logical Analysis:**
* `data.T`: Transposes the original data, changing its dimensions from m x n to n x m.
* `pd.get_dummies(data_T)`: Performs one-hot encoding, optimizing the computational complexity to O(nm).
# 3. Practical Cases of Transposed Matrices in Machine Learning
### 3.1 Natural Language Processing
#### 3.1.1 Application of Transposed Matrices in Text Classification
In text classification tasks, transposed matrices can be used to convert text data into a format suitable for classification models. Specifically, transposed matrices can arrange words in rows and documents in columns. Through this transformation, each document can be represented as a word vector, where each element represents the frequency of that word in the document.
```python
import numpy as np
from sklearn.feature_extraction.text import CountVectorizer
# Text data
texts = ["This is a sample text.", "This is another sample text."]
# Create a word vectorizer
vectorizer = CountVectorizer()
# Convert text data into word vectors
X = vectorizer.fit_transform(texts)
# Get word vectors
word_vectors = X.toarray()
# Transpose the word vectors
transposed_word_vectors = word_vectors.T
# Print the transposed word vectors
print(transposed_word_vectors)
```
**Code Logical Analysis:**
* Use `CountVectorizer` to convert text data into word vectors.
* Convert word vectors into a NumPy array.
* Use the `T` attribute to transpose word vectors.
* Print the transposed word vectors.
#### 3.1.2 Application of Transposed Matrices in Text Mining
In text mining tasks, transposed matrices can be used to discover patterns and relationships in text data. For example, by transposing text data, we can identify frequently occurring word pairs or groups.
```python
import numpy as np
from sklearn.feature_extraction.text import TfidfVectorizer
# Text data
texts = ["This is a sample text.", "This is another sample text."]
# Create a TF-IDF vectorizer
vectorizer = TfidfVectorizer()
# Convert text data into TF-IDF vectors
X = vectorizer.fit_transform(texts)
# Get TF-IDF vectors
tfidf_vectors = X.toarray()
# Transpose the TF-IDF vectors
transposed_tfidf_vectors = tfidf_vectors.T
# Print the transposed TF-IDF vectors
print(transposed_tfidf_vectors)
```
**Code Logical Analysis:**
* Use `TfidfVectorizer` to convert text data into TF-IDF vectors.
* Convert TF-IDF vectors into a NumPy array.
* Use the `T` attribute to transpose TF-IDF vectors.
* Print the transposed TF-IDF vectors.
### 3.2 Image Processing
#### 3.2.1 Application of Transposed Matrices in Image Enhancement
In image enhancement tasks, transposed matrices can be used to perform operations such as rotation and flipping on images. By transposing the image, we can change the dimensions of the image, thereby achieving image enhancement.
```python
import numpy as np
import cv2
# Read image
image = cv2.imread("image.jpg")
# Transpose image
transposed_image = np.transpose(image)
# Display the transposed image
cv2.imshow("Transposed Image", transposed_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**Code Logical Analysis:**
* Use `cv2.imread()` to read the image.
* Use `np.transpose()` to transpose the image.
* Use `cv2.imshow()` to display the transposed image.
* Use `cv2.waitKey(0)` to wait for user input.
* Use `cv2.destroyAllWindows()` to close all windows.
#### 3.2.2 Application of Transposed Matrices in Image Segmentation
In image segmentation tasks, transposed matrices can be used to segment the image into different regions. By transposing the image, we can change its dimensions, making it easier to identify different regions in the image.
```python
import numpy as np
import cv2
# Read image
image = cv2.imread("image.jpg")
# Transpose image
transposed_image = np.transpose(image)
# Use K-Means clustering to segment image
kmeans = cv2.kmeans(transposed_image.reshape(-1, 3), 3)
# Segment image into different regions
segmented_image = kmeans[1].reshape(image.shape)
# Display the segmented image
cv2.imshow("Segmented Image", segmented_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**Code Logical Analysis:**
* Use `cv2.imread()` to read the image.
* Use `np.transpose()` to transpose the image.
* Convert the transposed image into a one-dimensional array.
* Use `cv2.kmeans()` to perform K-Means clustering on the image.
* Convert the clustering results into a two-dimensional array.
* Use `cv2.imshow()` to display the segmented image.
* Use `cv2.waitKey(0)` to wait for user input.
* Use `cv2.destroyAllWindows()` to close all windows.
# 4.1 Deep Learning
### 4.1.1 Application of Transposed Matrices in Convolutional Neural Networks
In Convolutional Neural Networks (CNNs), transposed matrices are used to perform deconvolution operations, also known as transposed convolutions. Transposed convolutions are an upsampling operation for feature maps, which can increase the resolution of feature maps.
**Code Block:**
```python
import tensorflow as tf
# Define input feature maps
input_features = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Define a transposed convolution kernel
transpose_kernel = tf.constant([[0.5, 0.5], [0.5, 0.5]])
# Perform transposed convolution operation
output_features = tf.nn.conv2d_transpose(input_features, transpose_kernel, strides=[1, 1, 1, 1], padding='SAME')
# Print output feature maps
print(output_features)
```
**Logical Analysis:**
* `input_features` are the input feature maps, with a shape of `[3, 3, 1]`, where `3` represents the height and width of the feature maps, and `1` represents the number of channels.
* `transpose_kernel` is the transposed convolution kernel, with a shape of `[2, 2, 1, 1]`, where `2` represents the height and width of the kernel, and `1` represents the number of input and output channels.
* The `strides` parameter specifies the stride of the convolution operation, set to `[1, 1, 1, 1]`, indicating a stride of 1 in each dimension.
* The `padding` parameter specifies the padding mode of the convolution operation, set to `'SAME'`, indicating that the size of the output feature maps will be the same as the input feature maps.
* `output_features` is the output of the transposed convolution operation, with a shape of `[3, 3, 1]`, and the resolution is the same as the input feature maps.
### 4.1.2 Application of Transposed Matrices in Recurrent Neural Networks
In Recurrent Neural Networks (RNNs), transposed matrices are used to calculate gradients for updating model parameters during backpropagation.
**Code Block:**
```python
import tensorflow as tf
# Define a recurrent neural network cell
rnn_cell = tf.nn.rnn_cell.BasicRNNCell(num_units=10)
# Define an input sequence
input_sequence = tf.constant([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Define an output sequence
output_sequence, _ = tf.nn.dynamic_rnn(rnn_cell, input_sequence, dtype=tf.float32)
# Calculate gradients
gradients = tf.gradients(output_sequence, input_sequence)
# Print gradients
print(gradients)
```
**Logical Analysis:**
* `rnn_cell` is the recurrent neural network cell, and the `num_units` parameter specifies the dimension of the hidden state.
* `input_sequence` is the input sequence, with a shape of `[3, 3]`, where `3` represents the length of the sequence, and `3` represents the input dimension at each time step.
* `output_sequence` is the output sequence of the recurrent neural network, with a shape of `[3, 10]`, where `3` represents the length of the sequence, and `10` represents the output dimension at each time step.
* `gradients` are the gradients of the output sequence with respect to the input sequence, with a shape of `[3, 3]`, where `3` represents the length of the sequence, and `3` represents the gradient dimension at each time step.
* During backpropagation, transposed matrices are used to calculate gradients for updating model parameters.
# 5.1 Parallel Computing
### 5.1.1 Application of Transposed Matrices in Distributed Computing
In distributed computing, transposed matrices can be used to optimize data parallel processing. By transposing a matrix, data blocks can be allocated to different computing nodes for parallel computing, thereby improving computational efficiency.
**Code Block:**
```python
import numpy as np
from dask.distributed import Client
# Create a distributed client
client = Client()
# Create a large matrix
matrix = np.random.rand(10000, 10000)
# Transpose matrix
transposed_matrix = client.submit(np.transpose, matrix)
# Parallel compute matrix multiplication
result = client.submit(np.matmul, transposed_matrix, matrix)
# Get the computation result
result.result()
```
**Logical Analysis:**
* Use the `dask.distributed` library to create a distributed client.
* Create a large matrix `matrix`.
* Use `client.submit` to submit the matrix transpose task to the distributed client.
* Use `client.submit` to submit the matrix multiplication task to the distributed client.
* Use `result.result()` to get the computation result.
**Parameter Explanation:**
* `matrix`: The matrix to be transposed.
* `transposed_matrix`: The transposed matrix.
* `result`: The result of the matrix multiplication computation.
### 5.1.2 Application of Transposed Matrices in GPU Acceleration
In GPU acceleration, transposed matrices can be used to optimize data layout for improved performance of GPU kernels. By transposing the matrix, data can be organized into a form that is more suitable for GPU kernel parallel computing.
**Code Block:**
```python
import numpy as np
import cupy as cp
# Create a large matrix
matrix = np.random.rand(10000, 10000)
# Copy the matrix to the GPU
gpu_matrix = cp.asarray(matrix)
# Transpose matrix
transposed_gpu_matrix = cp.transpose(gpu_matrix)
# Use GPU kernel to compute matrix multiplication
result = cp.matmul(transposed_gpu_matrix, gpu_matrix)
# Copy the result back to the CPU
result = result.get()
```
**Logical Analysis:**
* Use the `cupy` library to copy the matrix to the GPU.
* Use `cp.transpose` to transpose the GPU matrix.
* Use GPU kernel to compute matrix multiplication.
* Copy the result back to the CPU.
**Parameter Explanation:**
* `matrix`: The matrix to be transposed.
* `gpu_matrix`: The matrix on the GPU.
* `transposed_gpu_matrix`: The transposed matrix on the GPU.
* `result`: The result of the matrix multiplication computation.
# 6. Future Prospects of Transposed Matrices in Machine Learning**
**6.1 Emerging Technologies**
**6.1.1 Application of Transposed Matrices in Quantum Machine Learning**
Quantum machine learning is an emerging field in machine learning that leverages the principles of quantum mechanics to solve problems that are difficult for traditional machine learning methods. Transposed matrices play a significant role in quantum machine learning because they can be used for:
- **Representation of quantum states:** Transposed matrices can be used to represent quantum states, which is crucial for the development and implementation of quantum algorithms.
- **Optimization of quantum gates:** Transposed matrices can be used to optimize the performance of quantum gates, thereby increasing the efficiency of quantum algorithms.
- **Analysis of quantum entanglement:** Transposed matrices can be used to analyze quantum entanglement, which is vital for understanding the complexity of quantum machine learning.
**6.1.2 Application of Transposed Matrices in Edge Computing**
Edge computing is a distributed computing paradigm that brings computation tasks closer to the data source. Transposed matrices play a significant role in edge computing because they can be used for:
- **Data preprocessing:** Transposed matrices can be used to preprocess data on edge devices, thereby reducing the overhead of data transmission.
- **Model compression:** Transposed matrices can be used to compress machine learning models, allowing them to be deployed on edge devices.
- **Inference acceleration:** Transposed matrices can be used to accelerate the inference process on edge devices, thereby improving real-time response capabilities.
**6.2 Expansion of Application Domains**
**6.2.1 Application of Transposed Matrices in Healthcare**
Transposed matrices have a wide range of applications in the healthcare sector, including:
- **Medical image analysis:** Transposed matrices can be used to analyze medical images, such as X-rays and MRI scans, to detect diseases and abnormalities.
- **Drug discovery:** Transposed matrices can be used to simulate the interaction between drugs and proteins, thereby accelerating the drug discovery process.
- **Personalized medicine:** Transposed matrices can be used to analyze patient data to develop personalized treatment plans.
**6.2.2 Application of Transposed Matrices in Financial Technology**
Transposed matrices have significant applications in the financial technology sector, including:
- **Risk management:** Transposed matrices can be used to analyze financial data to identify and manage risks.
- **Fraud detection:** Transposed matrices can be used to detect financial fraud, such as credit card fraud and money laundering.
- **Portfolio optimization:** Transposed matrices can be used to optimize portfolios to maximize returns and minimize risks.
0
0
相关推荐
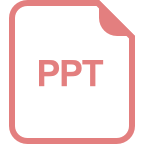
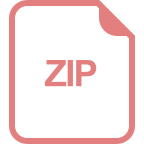






