Image Processing and Computer Vision Techniques in Jupyter Notebook
发布时间: 2024-09-15 17:50:32 阅读量: 94 订阅数: 43 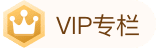
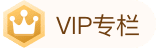
# Image Processing and Computer Vision Techniques in Jupyter Notebook
## Chapter 1: Introduction to Jupyter Notebook
### 2.1 What is Jupyter Notebook
Jupyter Notebook is an interactive computing environment that supports code execution, text writing, and image display. Its main features include:
- Supports multiple programming languages, such as Python, R, Julia, etc.;
- Can run in a browser without needing to install additional development environments;
- Allows mixing code and text, making experimentation and result presentation more convenient;
- Supports plugin extensions for easy integration with various tools.
### 2.2 Advantages and Characteristics of Jupyter Notebook
In the field of image processing and computer vision, Jupyter Notebook has many advantages:
- It provides a visual presentation of the image processing process, facilitating debugging and demonstration;
- The interactive notebook makes experimental data visualization more intuitive;
- Code and explanatory text are presented synchronously, making sharing and communication easier;
- Supports data visualization libraries, such as Matplotlib, Seaborn, etc., enriching the presentation forms.
This is an introduction to Jupyter Notebook along with its advantages and characteristics. Next, we will delve into the basics of image processing.
# 2. Basics of Image Processing
#### 2.1 Overview of Image Processing
Image processing refers to the techniques used to manipulate digital images to obtain desired information or improve image quality. Image processing generally includes the following:
- Image enhancement: Improving image quality and visualization through various techniques.
- Image restoration: Restoring images by removing noise or repairing damaged parts.
- Image compression: Reducing the storage space required for images through various encoding algorithms.
- Feature extraction: Extracting key features from images for subsequent image recognition and analysis.
#### 2.2 Introduction to Common Image Processing Libraries
In image processing, commonly used libraries include:
| Library Name | Language | Main Functions |
| ------------ | -------- | -------------- |
| OpenCV | C++/Python | Provides a wide range of image processing and computer vision functionalities |
| Pillow | Python | Supports image opening, manipulation, saving, etc. |
| scikit-image | Python | Offers various image processing algorithms and tools |
Code example: Using OpenCV to read an image and display it
```python
import cv2
import matplotlib.pyplot as plt
# Read the image
image = cv2.imread('image.jpg')
# Convert color space
image_rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# Display the image
plt.imshow(image_rgb)
plt.axis('off')
plt.show()
```
mermaid formatted flowchart example:
```mermaid
graph LR
A[Start] --> B(Image Enhancement)
B --> C(Image Restoration)
C --> D(Image Compression)
D --> E(Feature Extraction)
E --> F[End]
```
With the above content, we have a deeper understanding of the basics of image processing. The next section will continue to introduce image reading and display in Jupyter Notebook.
# 3. Image Reading and Display in Jupyter Notebook
## 3.1 Image Reading
In Jupyter Notebook, we can use common image processing libraries to read image files, such as OpenCV and PIL. Here is an example code using OpenCV to read an image:
```python
import cv2
# Read image file
img = cv2.imread('image.jpg')
# Display image size
height, width, channels = img.shape
print('Image size:', height, 'x', width)
# Display a message indicating successful reading
print('Image read successfully!')
```
## 3.2 Image Display
In Jupyter Notebook, we can use the Matplotlib library to display images. Here is an example code using Matplotlib to display an image:
```python
import matplotlib.pyplot as plt
import cv2
# Read image file
img = cv2.imread('image.jpg')
# Convert BGR format image to RGB format
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# Display image
plt.imshow(img)
plt.axis('off') # Hide the axes
plt.show()
```
Next, we will use a table to compare the pros and cons of the two methods:
| Method | Pros | Cons |
| ----------------- | ------------------------------- | ----------------------------- |
| OpenCV | Fast image reading | Supports fewer formats |
| Matplotlib | Supports a wide range of formats | Slower than OpenCV |
Below is a Mermaid formatted flowchart showing the process of image reading and display:
```mermaid
graph LR
A[Start] --> B[Read Image File]
B --> C[Display Image Size]
C --> D[Display Successful Reading Message]
D --> E[Convert BGR to RGB Format]
E --> F[Display Image]
F --> G[End]
```
This concludes the specific content of Chapter 3, wh
0
0
相关推荐








