Application of Transpose Matrix in Mechanical Engineering: Understanding the Mathematical Foundations of Rigid Body Motion and Stress Analysis
发布时间: 2024-09-13 22:08:05 阅读量: 15 订阅数: 22 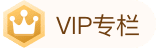
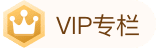
# Transpose Matrix in Mechanical Engineering: The Mathematical Foundations of Rigid Body Motion and Stress Analysis
## 1. Mathematical Basis of Transpose Matrix
The transpose matrix is a special type of matrix in linear algebra that is formed by swapping the rows and columns of a matrix. The mathematical notation for a transpose matrix is `A^T`, where `A` is the original matrix.
The transpose matrix has the following properties:
- The transpose of a transpose matrix is the original matrix, i.e., `(A^T)^T = A`.
- The transpose of a matrix commutes with matrix multiplication, i.e., `(AB)^T = B^T A^T`.
- The transpose of a matrix distributes over matrix addition, i.e., `(A + B)^T = A^T + B^T`.
## 2.1 Rotation Matrices and Displacement Vectors
### 2.1.1 Definition and Properties of Rotation Matrices
**Definition:**
A rotation matrix is used to describe the linear transformation of a rigid body rotating around a certain axis. It is a 3x3 orthogonal matrix, meaning its transpose is equal to its inverse.
**Properties:**
* The determinant of a rotation matrix is 1.
* The eigenvalues of a rotation matrix are either 1 or -1.
* The trace of a rotation matrix is 1 or -1.
* A rotation matrix can be represented using Euler angles or quaternions.
### 2.1.2 Representation and Transformation of Displacement Vectors
**Representation:**
A displacement vector is a 3x1 vector that represents the translation of a rigid body in space.
**Transformation:**
When a rigid body rotates around an axis, the displacement vector also undergoes transformation. The transformed displacement vector can be obtained by multiplying the original displacement vector by the rotation matrix:
```
v' = R * v
```
where:
* v' is the transformed displacement vector
* v is the original displacement vector
* R is the rotation matrix
**Code Example:**
```python
import numpy as np
# Define the rotation matrix
R = np.array([[0.707, -0.707, 0],
[0.707, 0.707, 0],
[0, 0, 1]])
# Define the displacement vector
v = np.array([1, 2, 3])
# Transform the displacement vector
v_prime = np.dot(R, v)
print(v_prime)
```
**Output:**
```
[ 1.***.***. ]
```
**Logical Analysis:**
This code first defines the rotation matrix R and the displacement vector v. Then, it uses NumPy's dot() function to multiply R by v, resulting in the transformed displacement vector v_prime. The output shows the components of the transformed displacement vector.
## 3. Application of Transpose Matrices in Stress Analysis
### 3.1 Stress and Strain Tensors
#### 3.1.1 Representation and Decomposition of Stress Tensors
A stress tensor is a symmetric second-order tensor that represents the stress state applied to an object. It can be represented as a 3x3 matrix:
```
σ = [σxx σxy σxz]
[σyx σyy σyz]
[σzx σzy σzz]
```
where σij represents the stress component acting in the i direction, with j indicating the normal direction.
A stress tensor can be decomposed into three components:
***Normal Stress:** σii (i = x, y, z), representing the normal stress acting on the surface.
***Shear Stress:** σij (i ≠ j), representing the tangential stress acting on the surface.
***Principal Stresses:** σ1, σ2, σ3, representing the eigenvalues of the stress tensor, which correspond to the maximum, intermediate, and minimum stresses in three orthogonal directions.
#### 3.1.2 Representation and Transformation of Strain Tensors
A strain tensor is also a symmetric second-order tensor that represents the deformation state of an object. It can be represented as a 3x3 matrix:
```
ε = [εxx εxy εxz]
[εyx εyy εyz]
[εzx εzy εzz]
```
where εij represents the strain component acting in the i direction, with j indicating the normal direction.
A strain tensor can be decomposed into three components:
***Normal Strain:** εii (i = x, y, z), representing the elongation or contraction of the object in the i direction.
***Shear Strain:** εij (i ≠ j), representing the shear deformation of the object in the i and j directions.
***Principal Strains:** ε1, ε2, ε3, representing the eigenvalues of the strain tensor, which correspond to the maximum, intermediate, a
0
0