The Role of Transpose Matrix in Computer Graphics: Understanding the Mathematical Foundations of 3D Transformations and Projections
发布时间: 2024-09-13 21:53:54 阅读量: 10 订阅数: 17 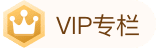
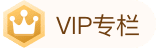
# 1. Overview of Transpose Matrix in Computer Graphics
The transpose matrix is a widely used mathematical tool in computer graphics, playing a crucial role in three-dimensional transformations, projections, and lighting calculations. Essentially, the transpose matrix swaps the rows and columns of a matrix. In computer graphics, it is used to represent transformations such as rotation, translation, and scaling. With the transpose matrix, it becomes easy to transform three-dimensional objects from one coordinate system to another, thereby achieving complex graphical operations.
In computer graphics, the application of the transpose matrix mainly focuses on three-dimensional transformations and projections. In three-dimensional transformations, the transpose matrix is used to represent rotations, translations, and scaling transformations. By multiplying the transformation matrix with the transpose matrix, three-dimensional objects can be transformed from one coordinate system to another. In projections, the transpose matrix is used to construct projection matrices, which project three-dimensional scenes onto two-dimensional screens. Adjusting the projection matrix allows control over the type of projection (e.g., orthogonal or perspective projection) and the field of view.
# 2. Mathematical Foundations of Transpose Matrix
### 2.1 Linear Algebra Basics
Linear algebra is a mathematical branch that studies vectors and matrices, providing the foundation for understanding the transpose matrix.
**Vector** represents an ordered set of numbers, used to describe points, directions, or other quantities. Vectors are typically denoted in **bold**, for example:
```
v = [x, y, z]
```
**Matrix** represents an array of numbers arranged in rows and columns. Matrices are typically denoted by **capital letters**, for example:
```
A = [a11 a12 a13]
[a21 a22 a23]
[a31 a32 a33]
```
### 2.2 Transpose of a Matrix
The transpose of a matrix is an operation that swaps the rows and columns of a matrix. For an **m x n** matrix **A**, its transpose **A<sup>T</sup>** is defined as:
```
A<sup>T</sup> = [a<sub>ij</sub><sup>T</sup>] = [a<sub>ji</sub>]
```
For example, for matrix **A**:
```
A = [1 2 3]
[4 5 6]
```
Its transpose is:
```
A<sup>T</sup> = [1 4]
[2 5]
[3 6]
```
### 2.3 Properties of Transpose Matrix
The transpose matrix has the following properties:
- **Symmetric Matrix:** If a matrix is equal to its transpose, it is called a symmetric matrix. That is: **A = A<sup>T</sup>**.
- **Inverse Matrix:** If a matrix is invertible, then the transpose of its inverse matrix is equal to the transpose of the original matrix. That is: **(A<sup>-1</sup>)<sup>T</sup> = A<sup>T</sup>**.
- **Determinant:** The determinant of a matrix is equal to the determinant of its transpose. That is: **det(A) = det(A<sup>T</sup>)**.
- **Trace:** The trace of a matrix is equal to the trace of its transpose. That is: **tr(A) = tr(A<sup>T</sup>)**.
- **Multiplication:** The transpose of the product of two matrices is equal to the product of the transposes of the two matrices in reverse order. That is: **(AB)<sup>T</sup> = B<sup>T</sup>A<sup>T</sup>**.
# 3. Application of Transpose Matrix in Three-dimensional Transformations
The transpose matrix plays a vital role in three-dimensional transformations, enabling the conversion of transformation matrices from one coordinate system to another. In three-dimensional graphics, common transformations include rotation, translation, and scaling.
### 3.1 Rotation Transformation
Rotation transformation refers to rotating an object around an axis. The rotation matrix can be represented as:
```python
R = [[cos(theta), -sin(theta), 0],
[sin(theta), cos(theta), 0],
[0, 0, 1]]
```
Where, `theta` is the rotation angle in radians.
**Logical Analysis:**
* The first row represents rotation around the x-axis.
* The second row represents rotation around the y-axis.
* The third row represents rotation around the z-axis.
**Parameter Description:**
* `theta`: Rotation angle (in radians)
### 3.2 Translation Transformation
Translation transformation refers to moving an object in a direction. The translation matrix can be represented as:
```python
T = [[1, 0, 0, tx],
[0, 1, 0, ty],
[0, 0, 1, tz],
[0, 0, 0, 1]]
```
Where, `tx`, `ty`, and `tz` represent the translation distances along the x, y, and z axes, respectively.
**Logical Analysis:**
* The fourth ro
0
0
相关推荐
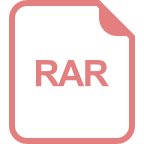
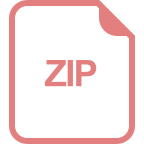
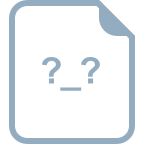





