[In-depth Exploration]: Implementing Advanced Query Techniques in MySQL with Python
发布时间: 2024-09-12 15:05:18 阅读量: 38 订阅数: 38 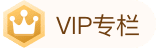
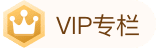
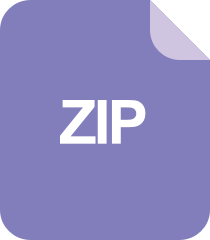
Golang---Exploration:Eksplorasi di GO
# In-depth Exploration: Implementing Advanced MySQL Query Techniques in Python
As data volumes surge, effectively retrieving information from databases has become increasingly critical. MySQL, as a widely-used open-source database management system, its advanced query techniques are indispensable skills for data analysts and database administrators. This chapter will explore the basics of some advanced query techniques in MySQL, including JOINs, subqueries, and index optimization, which not only help us quickly and accurately retrieve data from complex database structures but also greatly enhance query efficiency and response speed. The following chapters will delve into how to apply these techniques in Python and share some practical examples to deepen understanding and optimize query performance.
# 2. Python Database Programming Basics
### 2.1 Introduction to Python Database Programming
As a high-level programming language, Python has demonstrated its elegance and efficiency in handling database-related tasks. Python achieves interaction with databases through various means, including but not limited to direct use of APIs provided by databases and utilizing database abstraction layers (such as DB-API).
#### 2.1.1 Database Connection and Interaction
A database connection is the first step in performing database operations. Python communicates with different types of databases through database drivers, such as MySQL, PostgreSQL, ***mon connection methods include using native database drivers or indirectly using ORM frameworks like SQLAlchemy.
#### 2.1.2 Database Abstraction Layer in Python
The database abstraction layer is a very important concept in Python. DB-API is the Python Database Interface Specification, which defines a series of functions and variables, providing a general database operation interface. Using DB-API not only ensures compatibility between different databases but also reduces the complexity of direct database API operations. Through the database abstraction layer in Python, developers can write clearer and more maintainable code.
### 2.2 MySQL Database Connection
#### 2.2.1 Using MySQL Connector/Python
MySQL Connector/Python is an official MySQL database connector that allows Python programs to connect directly to MySQL databases. To use it, you first need to install the module, then create a connection, execute statements, and process results.
```python
import mysql.connector
from mysql.connector import Error
def create_server_connection(host_name, user_name, user_password):
connection = None
try:
connection = mysql.connector.connect(
host=host_name,
user=user_name,
passwd=user_password
)
print("MySQL Database connection successful")
except Error as err:
print(f"Error: '{err}'")
return connection
connection = create_server_connection('localhost', 'username', 'password')
```
The above code shows how to connect to a MySQL server using MySQL Connector/Python. Note that connection parameters (such as hostname, username, and password) need to be replaced according to actual circumstances.
#### 2.2.2 Using and Managing Connection Pools
A connection pool is a technique to improve the efficiency of database connections. It pre-creates a certain number of database connections and keeps them in a pool for reuse. Python uses connection pools to reduce the overhead of frequently opening and closing database connections, improving program performance.
### 2.3 SQL Injection Protection in Python
#### 2.3.1 Concept and Dangers of SQL Injection
SQL injection is a technique where attackers insert malicious SQL code snippets into input fields, thereby bypassing security measures to access or manipulate databases. Without proper protection measures, SQL injection can lead to data leaks, data corruption or deletion, and unauthorized data access, posing significant security risks.
#### 2.3.2 Use and Benefits of Prepared Statements
Prepared statements are an effective means to prevent SQL injection. Prepared statements are typically used with parameterized queries, allowing SQL statement templates to be compiled in advance and then filled with specific parameter values for execution. This method not only effectively prevents SQL injection but also improves query performance.
```python
import mysql.connector
from mysql.connector import Error
def execute_prepared_statement(connection, query, params):
try:
cursor = connection.cursor()
cursor.execute(query, params)
result = cursor.fetchall()
return result
except Error as err:
print(f"Error: '{err}'")
finally:
cursor.close()
connection = create_server_connection('localhost', 'username', 'password')
if connection.is_connected():
query = "SELECT * FROM users WHERE username = %s AND password = %s"
params = ('username', 'password')
results = execute_prepared_statement(connection, query, params)
print(results)
```
In this code snippet, we used prepared statements to query user information, where `%s` is a parameter placeholder, and `params` is used to pass parameter values, thus avoiding direct concatenation of parameters in the SQL statement, effectively preventing the risk of SQL injection.
### 2.4 Summary
This chapter first gave a brief introduction to Python database programming, including methods of database connection and interaction, and the application of the database abstraction layer in Python. Then, it focused on how to use MySQL Connector/Python to connect to MySQL databases and discussed the use and management of connection pools in detail. Next, we discussed the concept, dangers, and protection strategies of SQL injection, emphasizing the role of prepared statements in preventing SQL injection. Through the above content, this chapter provided the foundational knowledge of Python database programming and highlighted the importance of security. In subsequent chapters, we will delve into the technical details and best practices for implementing advanced MySQL queries using Python.
# 3. Implementing Advanced MySQL Queries in Python
## 3.1 Joint Query (JOIN)
### 3.1.1 Basic Syntax of Joint Query
Joint queries are one of the core skills in database operations, especially when it is necessary to extract related data from multiple tables. In Python, we usually use ORM tools like SQLAlchemy or write native SQL statements to implement joint queries.
The basic syntax for JOIN queries is as follows:
```sql
SELECT *
FROM table1
JOIN table2
ON table1.column_name = table2.column_name;
```
In th
0
0
相关推荐
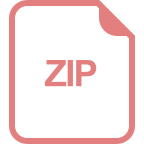
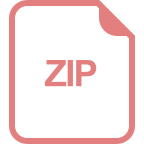
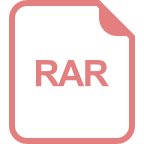
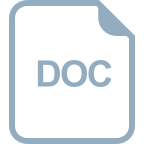
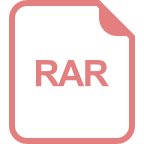
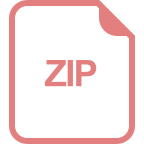
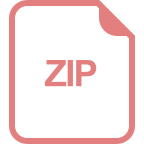
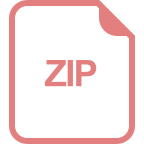