Python Operations on MySQL Data: Revealing Real-world CRUD Tips
发布时间: 2024-09-12 14:44:56 阅读量: 66 订阅数: 48 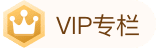
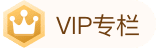
# Python Operations on MySQL Data: Advanced CRUD Techniques Revealed
In today's field of information technology, Python has become a popular programming language, offering great convenience and flexibility when interacting with MySQL databases. This chapter will serve as an introductory guide, leading readers through the basics of using Python to connect to MySQL databases and perform foundational database operations. This includes installing the necessary Python libraries, configuring database connections, and executing simple data queries and updates.
## 1.1 Installation and Configuration of Database Connections
Before you begin writing Python code to interact with MySQL, you must first ensure that MySQL is installed and that you have installed Python's database interface library. The most commonly used library is `mysql-connector-python`, which can be installed using pip:
```bash
pip install mysql-connector-python
```
Once installed, to configure your database connection, you will need to specify the database address, username, password, and the name of the database you wish to connect to. Below is a simple example of connection code:
```python
import mysql.connector
# Configure database connection parameters
db_config = {
'host': 'localhost',
'user': 'your_username',
'password': 'your_password',
'database': 'your_database'
}
# Create a database connection
cnx = mysql.connector.connect(**db_config)
# Create a cursor object
cursor = cnx.cursor()
```
## 1.2 Executing Basic Database Operations
With the cursor object created above, we can execute SQL statements to perform basic CRUD (Create, Read, Update, Delete) operations on a MySQL database. Below are some basic operation code examples:
```python
# Insert data
cursor.execute("INSERT INTO table_name (column1, column2) VALUES (%s, %s)", (value1, value2))
# Query data
cursor.execute("SELECT * FROM table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
# Update data
cursor.execute("UPDATE table_name SET column1 = %s WHERE column2 = %s", (value1, value2))
# Delete data
cursor.execute("DELETE FROM table_name WHERE column1 = %s", (value1,))
```
Beyond the basic content introduced above, operating MySQL databases in Python also includes more advanced topics such as exception handling, connection pooling, and performance optimization. These will be explained in detail in subsequent chapters. With the introduction of this chapter, you will lay a solid foundation for in-depth learning of advanced Python and MySQL interactions.
# 2. Detailed Explanation of CRUD Operations
## 2.1 Create Operation
### 2.1.1 Basic Method of Inserting Data
In database operations, creating (Create) is the first and crucial step. In Python, we typically use MySQLdb or PyMySQL libraries to create and manage databases. First, we need to establish a connection to the MySQL database, and then execute SQL statements to insert data.
```python
import MySQLdb
# Connect to the database
db = MySQLdb.connect("host", "user", "password", "database")
cursor = db.cursor()
# Prepare SQL statement for inserting data
sql = "INSERT INTO table_name (column1, column2) VALUES (%s, %s)"
val = ("value1", "value2")
try:
# Execute SQL statement
cursor.execute(sql, val)
# Commit transaction
***mit()
except MySQLdb.Error as e:
# Roll back transaction
db.rollback()
print(e)
finally:
# Close cursor and connection
cursor.close()
db.close()
```
This code demonstrates the basic method of inserting data using the MySQLdb library in Python. It shows the connection to the database, the creation of a cursor object, the execution of an insert statement, and the importance of committing the transaction and closing the cursor and connection to ensure proper resource release. Exception handling is also essential to properly manage any errors that may occur during the operation.
### 2.1.2 Batch Insertion and Performance Optimization
While inserting single pieces of data is simple, it can be highly inefficient when dealing with large amounts of data. Therefore, batch insertion is an effective method to improve insertion efficiency.
```python
import MySQLdb
# Connect to the database
db = MySQLdb.connect("host", "user", "password", "database")
cursor = db.cursor()
# Prepare batch insertion data
values = [
("value1", "value2"),
("value3", "value4"),
("value5", "value6"),
]
sql = "INSERT INTO table_name (column1, column2) VALUES (%s, %s)"
try:
# Execute batch insertion
cursor.executemany(sql, values)
***mit()
except MySQLdb.Error as e:
db.rollback()
print(e)
finally:
cursor.close()
db.close()
```
In the above code, the `cursor.executemany()` ***pared to inserting data row by row, `executemany()` can insert multiple pieces of data at once, significantly improving the efficiency of data insertion. Additionally, when processing a large amount of data, you can also consider turning on transactions, disabling auto-commit mode, to reduce database I/O operations, thus further optimizing performance.
## 2.2 Read Operation
### 2.2.1 Building Basic Query Statements
Query operations are the most frequent and important part of database operations. Building basic query statements usually involves the use of the SELECT statement, which can retrieve data from the database.
```python
import MySQLdb
# Connect to the database
db = MySQLdb.connect("host", "user", "password", "database")
cursor = db.cursor()
# Build basic query statement
sql = "SELECT column1, column2 FROM table_name WHERE condition"
try:
# Execute query statement
cursor.execute(sql)
# Get all query results
results = cursor.fetchall()
for row in results:
print(row)
except MySQLdb.Error as e:
print(e)
finally:
cursor.close()
db.close()
```
Executing the above Python script can retrieve the required data from the specified table based on conditions and return it as tuples. Here, the `fetchall()` method is used to obtain all results. If pagination is required, it can be combined with LIMIT and OFFSET.
### 2.2.2 Implementation Tips for Complex Queries
In real-world application scenarios, we often need to perform complex query operations, such as multi-table join queries, subqueries, and aggregate queries. This requires the use of SQL statements' powerful features to achieve complex data retrieval.
```python
import MySQLdb
# Connect to the database
db = MySQLdb.connect("host", "user", "password", "database")
cursor = db.cursor()
# Build complex query statement
sql = """
SELECT table1.column1, ***
***mon_field = ***mon_field
WHERE table1.column3 > %s
GROUP BY table1.column1
HAVING COUNT(*) > %s
ORDER BY table1.column1
LIMIT %s, %s
"""
try:
# Execute complex query statement
cursor.execute(sql, (value1, value2, offset, limit))
# Get paginated query results
results = cursor.fetchall()
for row in results:
print(row)
except MySQLdb.Error as e:
print(e)
finally:
cursor.close()
db.close()
```
In this example, we implemented an inner join query, grouped and aggregated results by a column, and then sorted and paginated the results. This is a relatively complex query operation, and through the understanding and application of SQL statements, we can effectively implement various data retrieval needs.
## 2.3 Update Operation
### 2.3.1 Strategies for Updating a Single Table
Data update operations are typically performed using the `UPDATE` statement, which allows us to modify existing records in a table. The correct update strategy is crucial for maintaining data integrity and accuracy.
```python
import MySQLdb
# Connect to the database
db = MySQLdb.connect("host", "user", "password", "database")
cursor = db.cursor()
# Build update statement
sql = "UPDATE table_name SET column1 = %s, column2 = %s WHERE condition"
try:
# Execute update operation
cursor.execute(sql, (value1, value2))
***mit()
except MySQLdb.Error as e:
db.rollback()
print(e)
finally:
cursor.close()
db.close()
```
In this example, we updated the `column1` and `column2` fields in the `table_name` table with new values and only modified records that met the `condition`. ***revent data conflicts and inconsistencies, it is common practice to lock the relevant records before updating.
### 2.3.2 Multi-table Joint Updates in Real-world Scenarios
In some complex business scenarios, it is necessary to update data in a table based on data from other tables, and this is where multi-table joint updates come into play.
```python
import MySQLdb
# Connect to the database
db = MySQLdb.connect("host", "user", "password", "database")
cursor = db.cursor()
# Build multi-table joint update statement
sql = """
UPDATE table1
SET table1.column = (SELECT column FROM table2 WHERE condition)
WHERE exists (***mon_field = ***mon_field AND condition)
"""
try:
# Execute multi-table joint update operation
cursor.execute(sql)
***mit()
except MySQLdb.Error as e:
db.rollback()
print(e)
finally:
cursor.close()
db.close()
```
This example demonstrates the application of updates across multiple tables. With subqueries, we can update corresponding data in `table1` based on data from `table2`. It is important to ensure that subqueries return the expected results and to pay attention to the performance impact of SQL statements, especially when dealing with large amounts of data.
## 2.4 Delete Operation
### 2.4.1 Principles of Safe Data Deletion
Data deletion should follow the principle of minimizing operations to ensure it does not affect the integrity of other related data. Before performing deletion operations, data should be fully backed up to prevent any mishaps.
```python
import MySQLdb
# Connect to the database
db = MySQLdb.connect("host", "user", "password", "database")
cursor = db.cursor()
# Build delete statement
sql = "DELETE FROM table_name WHERE condition"
try:
# Execute delete operation
cursor.execute(sql)
***mit()
except MySQLdb.Error as e:
db.rollback()
print(e)
finally:
cursor.close()
db.close()
```
In the above Python code, we used the `DELETE FROM` statement to delete records that met specific conditions. When executing delete operations, make sure the conditions are accurate to avoid accidentally deleting important data. Before deleting a large amount of data, consider using the `TRUNCATE` statement, which can more efficiently clear all data from a table.
### 2.4.2 The Difference Between Batch Deletion and L
0
0
相关推荐









