Python for Reading and Writing Large Datasets: Best Practices for MySQL Performance Optimization
发布时间: 2024-09-12 14:58:57 阅读量: 56 订阅数: 48 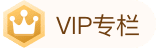
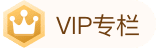
# Python Read and Write Large Datasets: Best Practices for MySQL Performance Optimization

# Create cursor object
cursor = connection.cursor()
# Execute SQL statement
cursor.execute("SELECT * FROM mytable")
# Fetch query results
results = cursor.fetchall()
# Output results
for row in results:
print(row)
# Close cursor and connection
cursor.close()
connection.close()
```
In the code above, we completed the entire process of connecting to the database, creating a cursor, executing a query, processing results, and closing the connection. Depending on specific requirements, this code will vary in actual applications. For example, to meet the needs of parameterized queries, the `cursor.execute()` method can be used to execute parameterized SQL statements.
Through the basic interaction between Python and MySQL, we can build more complex data manipulation logic, laying the foundation for building advanced applications. In the following chapters, we will delve into how to achieve efficient data reading and writing in big data scenarios and how to perform performance optimization.
# 2. Efficient Reading Techniques for Large Datasets
The rise of big data has increased the challenges faced by developers when dealing with data, and efficiently reading large amounts of data from databases has become an urgent need. This chapter will delve into how to use Python efficiently to read large datasets, including the use of database connection pools, the selection of reading strategies, and the processing and transformation of data streams.
## 2.1 The Use of Database Connection Pools
A database connection pool is a technology for managing database connections that can significantly improve the performance of a large number of database operations. When facing a large number of concurrent requests, connection pools can avoid the frequent establishment and closure of database connections, thereby reducing system overhead.
### 2.1.1 Basic Concepts of Connection Pools
A connection pool is a resource pooling technology that pre-creates a certain number of database connections and stores them in a pool. When an application needs to use a database connection, it can take one from the pool. After use, the connection is returned to the connection pool instead of being closed directly. Connection pools improve the performance of applications by reusing connections and can control the number of database connections to prevent excessive resource consumption.
### 2.1.2 Selection of Python Libraries for Implementing Connection Pools
In Python, there are several libraries that can be used to implement connection pools. The more famous ones include `pymysql` and `psycopg2`, which provide connection pool support for MySQL and PostgreSQL databases, respectively. Additionally, there is a general-purpose library `SQLAlchemy`, which supports multiple database systems and has built-in connection pool functionality. To implement more advanced connection pool features, the `DBUtils` library can also be used, which provides a `PooledDB` module that can create connection pools and perform advanced management.
### 2.1.3 Configuration and Performance Testing of Connection Pools
The configuration of connection pools typically includes the minimum number of connections, the maximum number of connections, and the strategies for acquiring and recycling connections. Taking `PooledDB` as an example, the size of the connection pool can be controlled by setting the `mincached` (minimum cached connections) and `maxcached` (maximum cached connections) parameters. Performance testing is an important part of verifying whether the connection pool configuration is reasonable. Tools such as `Apache JMeter` or `locust` can be used to simulate high-concurrency scenarios to observe the performance of the connection pool and the usage of database connections.
```python
from PooledDB import PooledDB
import pymysql
# Create a connection pool
connection_pool = PooledDB(
creator=pymysql, # Use PyMySQL to create connections
mincached=2, # Minimum cached connections
maxcached=5, # Maximum cached connections
maxshared=10, # Maximum shared connections
setsession=['SET NAMES utf8'], # Command list for initializing connections
ping=0 # Connection test command, 0 for no test
)
# Use a connection from the connection pool
connection = connection_pool.connection()
cursor = connection.cursor()
# Execute database operations...
cursor.close()
connection.close()
```
In the code above, we created a connection pool and obtained a connection from the pool to execute database operations. During performance testing, we assess the effectiveness of the connection pool by monitoring the usage of connections in the pool and the response time of the database.
## 2.2 Strategies for Reading Large Datasets
When reading large datasets from a database, strategies should be considered to optimize performance. This section will introduce techniques such as pagination queries, efficient data processing using cursors, and multi-threading and asynchronous I/O reading techniques.
### 2.2.1 Pagination Query Techniques
When the amount of data in the database is very large, querying all data at once often leads to excessively long query times, or even server unresponsiveness. Pagination query technology processes the result set in batches to reduce the load of a single query and improve performance.
```python
cursor.execute("SELECT * FROM big_table LIMIT %s OFFSET %s", (page_size, page * page_size))
```
The code above shows how to use the MySQL `LIMIT` and `OFFSET` clauses for pagination queries. Here, `page_size` is the amount of data for each query, and `page` is the current page number. By incrementally increasing the `page` value, you can effectively read large amounts of data from the database without putting too much pressure on database performance.
### 2.2.2 Efficient Data Processing Using Cursors
When the amount of data to be processed is very large and cannot be loaded into memory at once, cursors can be used for row-by-row data processing. Python's DB-API provides cursor objects that allow for iterative result set processing.
```python
with connection.cursor() as cursor:
cursor.execute("SELECT * FROM big_table")
for row in cursor:
process(row)
```
When using cursors, the database only returns one row of data until the next row is requested. This method has low memory requirements and is suitable for processing large result sets. `process(row)` is a function for processing each row of data and can be written based on actual business needs.
### 2.2.3 Multi-threading and Asynchronous I/O Reading Techniques
For I/O-intensive applications, multi-threading and asynchronous I/O are effective means of improving performance. Python's `threading` module and `asyncio` library can be used to implement concurrent reading.
```python
import threading
import pymysql
def fetch_data(page_size, page):
connection = pymysql.connect()
cursor = connection.cursor()
cursor.execute("SELECT * FROM big_table LIMIT %s OFFSET %s", (page_size, page * page_size))
# Process data...
connection.close()
threads = []
for i in range(num_pages):
page_size = 100 # Amount of data per page
t = threading.Thread(target=fetch_data, args=(page_size, i))
threads.append(t)
t.start()
for t in threads:
t.join()
```
This code segment creates multiple threads, ***revent excessive resource competition from opening too many threads at once, thread pools can be used to manage the creation and destruction of threads.
For asynchronous I/O, the `aiomysql` library can be used:
```python
import asyncio
import aiomysql
async def fetch_data(page_size, page):
async with aiomysql.create_pool(host='localhost', port=3306, user='user',
password='password', db='db', minsize=1, maxsize=10) as pool:
async with pool.acquire() as conn:
async with conn.cursor() as cursor:
await cursor.execute("SELECT * FROM big_table LIMIT %s OFFSET %s", (page_size, page * page_size))
result = await cursor.fetchall()
# Process data...
return result
async def main():
tasks = []
num_pages = 100
page_size = 100
for i in range(num_pages):
tasks.append(fetch_data(page_size, i))
results = await asyncio.gather(*tasks)
# Combine all results...
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
loop.close()
```
Here, the `asyncio` library and `aiomysql` asynchronous driver are used to implement asynchronous database queries. By using `asyncio.gather` to execute multiple asynchronous query tasks in parallel, data reading efficiency can be significantly improved.
## 2.3 Processing and Transformation of Data Streams
When processing large datasets, the handling and transformation of data streams is an essential step. Generators and iterators can efficiently handle continuous data streams, while data serialization and deserialization methods are the foundation for data exchange. Batch processing strategies can better manage memory usage and optimize performance.
### 2.3.1 Using Generators and Iterators
Generators are a special type of iterator in Python that allow a series of values to be returned using the `yield` keyword within a loop body, rather than returning a list all at once, thus achieving lazy loading of memory.
```python
def generator_query():
connection = pymysql.connect()
cursor = connection.cursor()
cursor.execute("SELECT * FROM big_table")
while True:
row = cursor.fetchone()
if row is None:
break
yield row
cursor.close()
connection.close()
# Use the generator
for row in generator_query():
process(row)
```
The generator `generator_query` iterates over the result set row by row and returns the next row of data with each iteration. This method is especially suitable for row-by-row pr
0
0
相关推荐

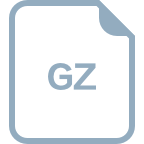





