【The Python and MySQL Interaction Guide】: The Ultimate Guide to Unlocking Database Operations
发布时间: 2024-09-12 14:36:02 阅读量: 28 订阅数: 30 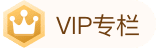
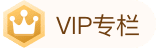
# [Python and MySQL Interaction Secrets]: Ultimate Guide to Database Operations
## 1.1 Introduction to Python Database Interaction
Python is a programming language widely used in Web development, data analysis, artificial intelligence, and more. MySQL is a popular open-source relational database management system. The interaction between Python and MySQL allows developers to leverage Python's capabilities to manage databases, execute SQL queries, and fulfill complex application requirements.
## 1.2 Basic Interaction Principles
The basic interaction between Python and MySQL involves three main steps: connection, query, and connection closure. Python establishes a connection to the MySQL server using specific libraries (such as `mysql-connector-python` or `pymysql`). Once connected, SQL statements can be sent to query or modify the database content. After execution, to release resources, the connection should be closed in a timely manner.
## 1.3 Python Database Connection and Query Technical Framework
To deeply understand the interaction between Python and MySQL, it is necessary to master some basic database connection techniques, such as installing connection drivers and understanding SQL syntax. This chapter will gradually analyze how Python interacts with MySQL databases from theory to practice, providing a solid theoretical foundation for subsequent application practices and optimizations.
```python
# Example code: Using the pymysql library to connect to a MySQL database
import pymysql
# Creating a connection
connection = pymysql.connect(host='localhost', user='user', password='password', db='db')
try:
with connection.cursor() as cursor:
# Executing an SQL query
sql = "SELECT `id`, `name` FROM `users`"
cursor.execute(sql)
results = cursor.fetchall()
for row in results:
print(row)
finally:
connection.close()
```
From the above code example, we can see that building interactions between Python and MySQL databases is achieved through establishing connections, executing SQL statements, and closing connections. In the following chapters, we will discuss in detail topics such as connection library selection, secure construction of SQL statements, and query result processing.
# 2. Building Python Database Connections and Queries
### 2.1 Python Database Connection Basics
#### 2.1.1 Selection of Tools and Libraries for Connecting to MySQL Databases
When choosing tools and libraries for connecting to MySQL databases, Python developers have several options. One of the most commonly used libraries is `mysql-connector-python`, an official driver provided by MySQL that supports Python 3.X versions. Another popular choice is `PyMySQL`, a pure Python library designed for interaction with MySQL servers with good community support and documentation. Finally, you can also choose to use `SQLAlchemy`, a powerful ORM (Object-Relational Mapping) tool that supports multiple databases, including MySQL.
When choosing a library suitable for the project, consider the following factors:
- **Project requirements**: Whether ORM support is needed or only the execution of native SQL statements.
- **Performance**: Different libraries may have varying performance levels, especially under high concurrency and large data volumes.
- **Community and support**: Choosing an active community and well-supported library can reduce potential issues.
- **Compatibility**: Ensure that the selected library is compatible with your Python version.
#### 2.1.2 Establishing and Closing Database Connections
Establishing a database connection is the first step in database operations. Here is an example using `mysql-connector-python` to show how to establish and close connections.
```python
import mysql.connector
# Establishing a connection
try:
connection = mysql.connector.connect(
host='localhost', # Database host address
database='test_db', # Database name
user='root', # Username
password='password' # Password
)
print('MySQL Database connection successful')
except mysql.connector.Error as err:
print(f'Error: {err}')
finally:
if connection.is_connected():
connection.close() # Closing the connection
print('MySQL connection is closed')
```
In the above code, we first import the `mysql.connector` module and use the `connect` method to establish a connection. After the connection is established, we use the `is_connected` method to check if the connection was successful and close the connection after operations are completed.
### 2.2 Combining SQL Queries with Python Applications
#### 2.2.1 Constructing Secure SQL Query Statements
When interacting with MySQL using Python, constructing secure SQL query statements is particularly important to avoid security issues such as SQL injection. It is recommended to use parameterized queries, passing parameters through placeholders, allowing the database driver to automatically handle parameter conversion and escaping.
Here is an example of using `mysql-connector-python` for parameterized queries:
```python
try:
connection = mysql.connector.connect(
host='localhost',
database='test_db',
user='root',
password='password'
)
cursor = connection.cursor() # Creating a cursor
insert_query = "INSERT INTO users (username, password) VALUES (%s, %s)"
data = ('john_doe', 'johndoe123')
cursor.execute(insert_query, data) # Executing a parameterized query
connection.***mit() # Committing the transaction
except mysql.connector.Error as err:
print(f'Error: {err}')
finally:
if connection.is_connected():
cursor.close() # Closing the cursor
connection.close() # Closing the connection
```
In this example, the `cursor.execute()` method is used to execute an SQL statement with placeholders, and `data` is a tuple containing the parameters to be inserted.
#### 2.2.2 Using Python to Execute SQL Statements
To execute SQL statements, apart from using the `cursor.execute()` method, queries like `SELECT` can be executed, and the returned result set can be processed.
```python
try:
cursor.execute("SELECT username FROM users WHERE username=%s", ('john_doe',))
result = cursor.fetchone() # Fetching one record of the result set
print("Username:", result)
except mysql.connector.Error as err:
print(f'Error: {err}')
finally:
if connection.is_connected():
cursor.close()
connection.close()
```
In the above code, the `fetchone()` method is used to retrieve the next record in the query result set. If there are multiple records in the query result, the `fetchone()` method can be called in a loop to obtain them.
### 2.3 Advanced Database Operation Practices
#### 2.3.1 Handling Complex SQL Query Result Sets
When dealing with complex query result sets, the entire result can be fetched by iterating over the `cursor` object.
```python
try:
cursor.execute("SELECT * FROM users")
for result in cursor:
print("ID:", result[0], "Username:", result[1])
except mysql.connector.Error as err:
print(f'Error: {err}')
finally:
if connection.is_connected():
cursor.close()
connection.close()
```
In this code block, the `cursor` object acts like an iterator, returning the next record in the query result set with each loop.
#### 2.3.2 Using Python for Transaction Control and Stored Procedure Calls
In database operations, transaction control is an important concept that ensures a series of operations either fully succeed or fully roll back. Transaction control in MySQL using Python can be implemented through the `***mit()` and `connection.rollback()` methods.
Here is an example of transaction control:
```python
try:
connection = mysql.connector.connect(
host='localhost',
database='test_db',
user='root',
password='password'
)
cursor = connection.cursor() # Creating a cursor
connection.start_transaction() # Starting a transaction
cursor.execute("INSERT INTO users (username, password) VALUES (%s, %s)", ('jane_doe', 'janedoe123'))
# Execute more operations...
connection.***mit() # Committing the transaction
except mysql.connector.Error as err:
print(f'Error: {err}')
connection.rollback() # Rolling back the transaction
finally:
if connection.is_connected():
cursor.close()
connection.close()
```
The above code demonstrates how to start a transaction, execute operations, and commit the transaction in the absence of errors or roll back the transaction in case of errors.
In MySQL, stored procedures are sets of SQL statements designed for specific functions. Python can call stored procedures using the `CALL` statement.
```python
try:
cursor.callproc('proc_name', [param1, param2]) # Calling a stored procedure
except mysql.connector.Error as err:
print(f'Error: {err}')
finally:
if connection.is_connected():
cursor.close()
connection.close()
```
In this code block, the `callproc` method is used to call a stored procedure named `proc_name` and pass in relevant parameters. Python supports the calling of stored procedures through the `callproc` method of the cursor object.
In-depth study and practice of this chapter will lay a solid foundation for further exploring interactions between Python and MySQL, providing a wealth of tools and methods for solving more complex database interaction requirements. With the accumulation of practical experience, developers will be able to utilize Python for efficient and secure database operations more effectively.
# 3. Advanced Techniques in Python Database Programming
#### 3.1 Application of ORM Frameworks in Python
##### 3.1.1 Overview and Selection of ORM Frameworks
Object-Relational Mapping (ORM) is a very popular pattern in database programming that provides a method to convert between programming language objects and database tables, reducing the complexity of database operations. With ORM frameworks, developers can manipulate databases in an object-oriented manner without writing complex SQL statements.
In the Python world, there are several popular ORM frameworks to choose from, including but not limited to:
- **SQLAlchemy**: SQLAlchemy is a powerful ORM and database toolkit for Python that supports a wide range of database systems.
- **Django ORM**: Django is a full-stack framework with an integrated ORM that is closely integrated with Django and is ideal for rapid web application development.
- **Peewee**: Peewee is a small, easy-to-use ORM framework suitable for rapid iteration and small projects.
Choosing an appropriate ORM framework usually depends on the project's scale, requirements, and team familiarity. For beginners, Peewee may be easier to learn; for large enterprise applications, SQLAlchemy offers more flexibility and scalability.
##### 3.1.2 Implementing Database Model Definitions Using ORM Frameworks
Defining database models using ORM frameworks is accomplished by defining Python classes whose attributes and methods map to columns and operations in the database. Taking SQLAlchemy as an example, we can define models as follows:
```python
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Defining a base class
Base = declarative_base()
# Defining a model class mapped to a table in the database
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
fullname = Column(String)
nickname = Column(String)
# Creating a database engine
engine = create_engine('sqlite:///example.db')
# Creating all tables
Base.metadata.create_all(engine)
# Creating a session
Session = sessionmaker(bind=engine)
session = Session()
```
In this example, we define a `User` class with four fields corresponding to the `users` table in the database. With the tools provided by SQLAlchemy, we create a database engine, define table structures, and create a database session, which can be used to perform database operations.
The advantage of using ORM frameworks is that they make database operations more intuitive and object-oriented, while also providing some abstraction layers, such as automatically handling table creation and relationship definitions.
#### 3.2 Application of Database Connection Pools
##### 3.2.1 Concept and Advantages of Connection Pools
A database connection pool is a technology used to manage and reuse database connections. When an application needs to interact with a database, the connection pool provides a pre-opened connection instead of creating a new one each time. The main advantages of connection pools include:
- **Reducing resource consumption**: Reducing the time and resources spent connecting to the database.
- **Improving performance**: Since establishing new connections takes less time, the database's response speed will be faster.
- **Increasing concurrency performance**: The connection pool allows limited database connections to be used more efficiently, supporting more concurrent operations.
In Python, libraries such as `db_pool` and `SQLAlchemy` can be used to implement connection pools.
##### 3.2.2 Implementing Connection Pool Technology in Python
Implementing connection pools in Python usually involves choosing mature libraries to avoid potential errors that may arise from custom connection pools. SQLAlchemy, as an ORM framework, also supports the use of connection pools.
```python
from sqlalchemy import create_engine
# Creating an engine with a connection pool
engine = create_engine('sqlite:///example.db', pool_size=5, max_overflow=10)
# Using the engine for database operations
```
In this example, the `pool_size` parameter defines the size of the connection pool, and the `max_overflow` parameter defines the number of connections that can exceed the pool size. These parameters ensure that the connection pool can provide sufficient connections in high-concurrency scenarios.
#### 3.3 Big Data Volume Processing and Performance Optimization
##### 3.3.1 Techniques for Pagination Queries and Batch Inserts
When dealing with large amounts of data, pagination queries and batch inserts are very important techniques:
- **Pagination queries**: Pagination can prevent memory overflow and performance degradation caused by loading too much data at once. In SQLAlchemy, pagination can be implemented as follows:
```python
from sqlalchemy.sql import text
# Building a pagination query
stmt = text("SELECT * FROM users")
stmt = stmt.limit(10).offset(20)
results = session.execute(stmt).fetchall()
```
- **Batch inserts**: When inserting large amounts of data, using batch inserts can significantly improve performance. In SQLAlchemy, batch inserts can be performed using the `execute` method.
```python
# Building a batch insert statement
stmt = text("INSERT INTO users (name, fullname, nickname) VALUES (:name, :fullname, :nickname)")
# Preparing the data to be inserted
users_to_insert = [
{'name': 'name1', 'fullname': 'Full Name 1', 'nickname': 'nick1'},
{'name': 'name2', 'fullname': 'Full Name 2', 'nickname': 'nick2'},
# More data...
]
# Executing the batch insert
session.execute(stmt, users_to_insert)
***mit()
```
##### 3.3.2 Python Code P***
***mon performance analysis tools in Python include `cProfile` and `line_profiler`. `cProfile` provides function-level call statistics, while `line_profiler` provides the execution time for each line of code.
Once performance bottlenecks are identified, optimization can be achieved through the following strategies:
- **Using built-in functions and libraries**: Python's built-in functions and standard libraries are usually optimized, and using them is more efficient than custom functions.
- **Avoiding unnecessary data copying**: For example, using generator expressions instead of list comprehensions.
- **Using local variables**: Accessing local variables is usually faster than accessing global variables.
- **Using caching**: Caching frequently used calculation results can significantly improve performance.
By employing these strategies, we can effectively improve the execution efficiency of Python code, especially when interacting with databases. Performance optimization is a continuous process that requires constant monitoring, analysis, and adjustments.
This concludes the third chapter; we will continue to delve into advanced interaction techniques between Python and MySQL in the following sections.
# 4. Advanced Interaction Techniques Between Python and MySQL
## 4.1 Advanced Query Techniques
### 4.1.1 Example of Using Python for Complex Queries
When dealing with complex business logic, we need to perform complex queries such as multi-table joins and grouping aggregations. In Python, using the pandas library in conjunction with SQLAlchemy can achieve the construction of complex queries and powerful data processing on the results. Below is an example code block of using Python for complex queries:
```python
import pandas as pd
from sqlalchemy import create_engine
# Connecting to the database
engine = create_engine('mysql+pymysql://username:password@host/dbname')
# Building a complex query statement
query = """
SELECT t1.*, t2.*
FROM table1 t1
JOIN table2 t2 ON t1.id = t2.fk_id
WHERE t1.column1 = 'condition'
GROUP BY t1.id
HAVING COUNT(t1.id) > 10
ORDER BY t1.created_at DESC
LIMIT 10;
"""
# Executing the query and loading into a DataFrame
df = pd.read_sql_query(query, engine)
# Processing the query result set
# Example: Displaying the first few rows of the DataFrame
print(df.head())
```
In the above code, we first imported the necessary libraries: pandas for data processing and SQLAlchemy for building database engines. Then, we constructed a complex SQL query statement that includes multi-table joins, conditional filtering, grouping aggregation, sorting, limiting the number of results, and more. With the `pd.read_sql_query` function, we can execute the SQL statement and load the results into a pandas DataFrame object, facilitating further data analysis and processing.
### 4.1.2 SQL Joins and Python Data Processing
In multi-table queries, the join operation is one of the key steps, allowing us to merge data from multiple related tables based on common fields. In Python, we can utilize the DataFrame object from the pandas library to process join data. Here is an example of using a DataFrame to perform a join operation:
```python
import pandas as pd
# Assuming we already have two tables loaded into DataFrames
df_table1 = pd.DataFrame({'id': [1, 2, 3], 'value': ['A', 'B', 'C']})
df_table2 = pd.DataFrame({'id': [1, 2, 3], 'score': [90, 85, 80]})
# Using the merge function for an inner join
df_inner_join = pd.merge(df_table1, df_table2, on='id', how='inner')
# Using the merge function for an outer join
df_outer_join = pd.merge(df_table1, df_table2, on='id', how='outer')
# Outputting the join results
print("Inner join result:")
print(df_inner_join)
print("\nOuter join result:")
print(df_outer_join)
```
In this example, we first created two DataFrame objects simulating two tables. Then we used the `pd.merge` function to implement an inner join (`how='inner'`) and an outer join (`how='outer'`). The `merge` function's `on` parameter specifies the key used for joining (the common field), and the `how` parameter defines the type of join. This way, we can easily handle complex join operations in Python and further process the resulting dataset.
## 4.2 Database Security and Protection
### 4.2.1 SQL Injection Protection and Code Auditing
SQL injection is a common form of database attack where attackers inject malicious SQL code that can lead to unauthorized data access or database damage. The most effective method to prevent SQL injection in Python code is to use parameterized queries. Here are some best practices for preventing SQL injection:
- Use ORM frameworks (such as SQLAlchemy) that inherently prevent SQL injection.
- When executing native SQL, use prepared statements and parameterized queries.
- Never concatenate user input directly into SQL query statements.
Here is an example of safely executing SQL queries:
```python
from sqlalchemy import create_engine, text
# Building a secure SQL query statement
def safe_query():
engine = create_engine('mysql+pymysql://username:password@host/dbname')
with engine.connect() as conn:
# Using parameterized queries to prevent SQL injection
query = text("SELECT * FROM users WHERE username = :username AND password = :password")
result = conn.execute(query, {'username': 'admin', 'password': 'securepassword'})
return result.fetchall()
# Calling the function to execute a safe query
data = safe_query()
print(data)
```
In this example, we used the `text` object provided by SQLAlchemy to create a query statement with parameter placeholders. By passing parameters through the `execute` method, queries can be safely executed without the threat of SQL injection. This practice is essential when performing any database operations involving user input, ensuring the security of database operations.
### 4.2.2 MySQL Permission Management and Python Applications
Database permission management is an important part of database security, and proper permission settings can effectively prevent unauthorized operations. In Python applications, we can manage MySQL user permissions to restrict database access. Here is an example of how to manage MySQL user permissions using a Python script:
```python
from sqlalchemy import create_engine
from sqlalchemy.engine import reflection
# Creating a database engine
engine = create_engine('mysql+pymysql://root:password@localhost/dbname')
# Checking for a specific user
with engine.connect() as conn:
inspector = reflection.Inspector.from_engine(conn)
users = inspector.get_users()
if 'new_user' not in users:
# Creating a new user
conn.execute("CREATE USER 'new_user' IDENTIFIED BY 'secure_password';")
# Granting specific permissions
conn.execute("GRANT SELECT, INSERT ON dbname.* TO 'new_user';")
print("New user created and permissions assigned successfully.")
else:
print("The user already exists.")
```
In this example, we first established a connection to the MySQL database using an engine and used the `reflection.Inspector.from_engine` method to check for existing users. If the desired user does not exist, we execute the creation of a new user and the assignment of permissions. This process utilizes SQLAlchemy's reflection mechanism to inspect and modify the database's metadata information, which is a powerful database management approach.
## 4.3 Data Backup and Recovery
### 4.3.1 Data Backup Strategies and Python Script Implementation
Data backup is an important task in database management, which helps to quickly restore data in case of data loss or damage. Python can be used to automate the backup process by executing SQL statements or using database management tools for backup. Here is an example of using Python to back up a MySQL database:
```python
import subprocess
import os
def backup_database():
# Database connection information
username = 'root'
password = 'password'
database = 'dbname'
backup_file = 'backup.sql'
# Building the mysqldump command
command = f"mysqldump -u {username} -p{password} {database} > {backup_file}"
# Executing the command for backup
process = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = ***municate()
if process.returncode == 0:
print(f"Backup successful, file saved at: {backup_file}")
else:
print(f"Backup failed, error message: {stderr.decode()}")
# Executing the backup function
backup_database()
```
In this example, we used the `subprocess.Popen` method to execute the `mysqldump` command for database backup, which is an official MySQL backup tool. We constructed a command string, including the database username, password, database name, and backup file name. We executed the command and captured the output using the `communicate()` method to determine if the backup was successful.
### 4.3.2 Data Recovery Methods and Automated Operation Examples
Data recovery is the reverse process of backup, which means restoring data from the backup file to the database. In Python, we can use similar logic to implement automated data recovery. Here is an example of using Python to perform data recovery:
```python
import subprocess
def restore_database(backup_file):
# Database connection information
username = 'root'
password = 'password'
# Building the mysql command
command = f"mysql -u {username} -p{password} < {backup_file}"
# Executing the command for recovery
process = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = ***municate()
if process.returncode == 0:
print("Data recovery successful.")
else:
print(f"Data recovery failed, error message: {stderr.decode()}")
# Assuming the backup file path is 'path/to/backup.sql'
backup_file = 'path/to/backup.sql'
restore_database(backup_file)
```
This example is similar to the backup example, but here we used the `mysql` command instead of `mysqldump`. We constructed a command string for executing data recovery and then executed the command using the `subprocess.Popen` method. If the command executes successfully, data recovery is complete; if it fails, the error message can be used to determine the cause of failure.
By writing Python scripts to automate these operations, management tasks can be simplified, and data can be quickly recovered in the event of an意外. In actual applications, backup and recovery scripts are usually integrated into scheduled tasks, such as using Linux's `cron` or Windows Task Scheduler.
# 5. Case Studies of Python and MySQL Interaction
## 5.1 Introduction to Real-World Application Scenarios
### 5.1.1 Database Interaction Requirements in Common Business Scenarios
In real-world application development, the diversity and complexity of business scenarios require developers to have a profound understanding of database interactions. For example, in an e-commerce platform, inventory management, order processing, user behavior tracking, and more all require frequent database interactions. In social media applications, storing user information, managing friend relationships, and publishing dynamic information all rely on the efficient processing of databases. In addition, content management systems, ERP software, Internet of Things data storage, and other scenarios also involve complex database operation requirements.
### 5.1.2 Business Logic and Database Design in Project Case Examples
Taking an online retail platform as an example, its business logic may include user registration, login, product browsing, shopping cart management, order generation, payment processing, and inventory updates. Database design needs to consider data integrity, consistency, and security. Therefore, several key tables may be needed:
- User table (User)
- Product table (Product)
- Order table (Order)
- Order Detail table (Order Detail)
- Inventory table (Inventory)
The design of each table needs to consider its correspondence with the business process, such as how the inventory table should reflect the product inventory in real-time and interact with the order table to ensure the correct update of the inventory count.
## 5.2 Case Practice: Building a Complete Web Application Database Interaction
### 5.2.1 Using Django or Flask Frameworks to Interact with MySQL
Using Python's Django or Flask framework can quickly build Web applications and implement interactions with MySQL databases. Taking Django as an example, developers can leverage its built-in ORM system to simplify database operations. First, define the data model (Models) mapping to the database table structure, and Django will automatically generate the corresponding table structure based on the model.
```python
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=100)
description = models.TextField()
price = models.DecimalField(max_digits=10, decimal_places=2)
stock = models.IntegerField()
class Order(models.Model):
customer = models.ForeignKey('auth.User', on_delete=models.CASCADE)
date_ordered = models.DateTimeField(auto_now_add=True)
complete = models.BooleanField(default=False)
# Omitting the definition of other models...
```
After defining the models, by running `python manage.py makemigrations` and `python manage.py migrate` commands, Django will automatically handle database migrations and create the corresponding table structure.
### 5.2.2 Implementing CRUD Operations and Data Processing for Complex Business Logic
In Django, CRUD operations (Create, Read, Update, Delete) on the database can be easily completed through the ORM (Object-Relational Mapping) interface. Here are some operation examples:
```python
# Creating a product record
new_product = Product(name="Python Programming", price=35.00)
new_product.save()
# Querying product records
products = Product.objects.filter(price__gte=10)
# Updating the product price
product = Product.objects.get(id=1)
product.price = 30.00
product.save()
# Deleting a product record
product_to_delete = Product.objects.get(id=2)
product_to_delete.delete()
```
For complex business logic, such as automatic deduction of inventory and updating order status, you can handle this within the model by writing custom methods or using Django's signals mechanism to trigger specific events.
## 5.3 Case Analysis and Conclusion
### 5.3.1 Analyzing Problem-Solving Strategies in the Case
In the online retail platform case mentioned above, some problems may be encountered, such as inventory overselling and payment security. To solve these problems, a series of strategies are needed:
- Use transaction control to ensure the atomicity of operations, for example, wrapping the inventory deduction and order generation in transactions.
- Utilize database constraints, such as foreign keys and uniqueness constraints, to ensure data integrity.
- Use prepared statements during data interaction to prevent SQL injection attacks.
### 5.3.2 Summarizing Best Practices and Lessons Learned
Through the analysis of actual cases, we can summarize the following best practices:
- Database design should closely follow business requirements, avoiding overdesign or underdesign.
- Leverage ORM tools provided by Python frameworks to simplify database operations and improve development efficiency.
- Reasonably use transactions in business logic to ensure data consistency.
- Prevent security vulnerabilities, especially in data processing and user input.
By continuously applying and optimizing these practices, you can build Web applications with efficient and secure database interactions.
0
0
相关推荐
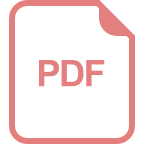
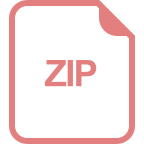
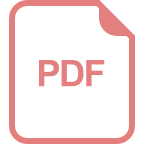
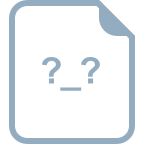
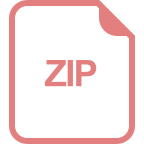
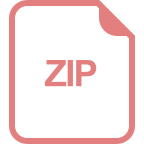
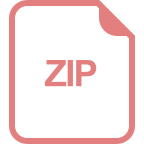
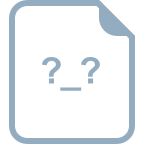
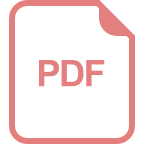