Mastering Logical Operators in MATLAB: The Foundation of Conditional Judgments (with 10 Application Scenarios)
发布时间: 2024-09-13 18:06:48 阅读量: 26 订阅数: 23 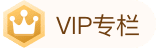
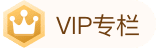
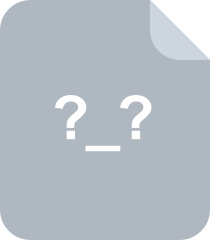
Mastering the C++17 STL Make full use of the standard library components in epub

# Logical Operators in MATLAB: The Foundation of Conditional Judgments (with 10 Application Scenarios)
Logical operators are fundamental tools in MATLAB for performing logical operations. They enable you to compare values, combine conditions, ***monly used logical operators in MATLAB include:
***& (AND)**: Returns true when both conditions are true.
***| (OR)**: Returns true when at least one condition is true.
***~ (NOT)**: Reverses the truth value of a condition.
These operators can be combined to create complex conditional expressions. For example, the following expression will return true if `x` is greater than 5 and `y` is less than 10:
```
x > 5 & y < 10
```
# 2.1 The Basics of Conditional Judgments
### 2.1.1 Relational Operators
Relational operators are used to compare two values and return a boolean value (true or false). Commonly used relational operators in MATLAB are:
| Operator | Description |
|---|---|
| == | Equal to |
| ~= | Not equal to |
| < | Less than |
| <= | Less than or equal to |
| > | Greater than |
| >= | Greater than or equal to |
**Example:**
```matlab
a = 5;
b = 3;
a == b % Returns false
a ~= b % Returns true
a < b % Returns false
a <= b % Returns false
a > b % Returns true
a >= b % Returns true
```
### ***
***monly used logical operators in MATLAB are:
| Operator | Description |
|---|---|
| & | AND |
| \| | OR |
| ~ | NOT |
**Example:**
```matlab
a = true;
b = false;
a & b % Returns false
a | b % Returns true
~a % Returns false
```
**Logical Operator Precedence:**
The precedence of logical operators from highest to lowest is:
1. ~
2. &
3. |
**Code Logic Analysis:**
In the above examples, `a & b` calculates the AND value of `a` and `b`, and since `b` is false, the result is false. `a | b` calculates the OR value of `a` and `b`, and since `a` is true, the result is true. `~a` calculates the NOT value of `a`, and since `a` is true, the result is false.
# 3. Practical Application of Logical Operators in MATLAB
### 3.1 Data Filtering and Extraction
Logical operators are widely used in MATLAB for data filtering and extraction tasks. By using relational operators and logical operators, we can search for and extract the required elements from a dataset based on specific conditions.
#### 3.1.1 Finding Specific Elements
To find elements in a dataset that meet specific conditions, we can use relational operators (such as `==`, `~=`, `>`, `<`) and logical operators (such as `&`, `|`). For example, the following code finds all elements greater than 5 in an array:
```
% Create an array
data = [1, 3, 5, 7, 9, 11, 13, 15];
% Find elements greater than 5
greater_than_five = data > 5;
% Display elements greater than 5
disp(greater_than_five)
```
Output:
```
[***]
```
#### 3.1.2 Extracting a Subset That Meets Conditions
To extract a subset of data that meets specific conditions, we can use logical indexing. Logical indexing is a boolean array where `true` elements correspond to elements that meet the condition. For example, the following code extracts all even elements from an array:
```
% Create an array
data = [1, 3, 5, 7, 9, 11, 13, 15];
% Extract even elements
even_indices = mod(data, 2) == 0;
even_subset = data(even_indices);
% Display even subset
disp(even_subset)
```
Output:
```
[***]
```
### 3.2 Decision Control
Logical operators in MATLAB are also used for decision control, such as branch statements and loop statements. By using logical conditions, we can control the flow of the program and execute different blocks of code based on various conditions.
#### 3.2.1 Branch Statements
Branch statements (such as `if-else` and `switch-case`) allow us to execute different blocks of code based on logical conditions. For example, the following code uses an `if-else` statement to print different messages based on the input value:
```
% Get user input
input_value = input('Enter a number: ');
% Print message based on input value
if input_value > 0
disp('The number is positive.')
elseif input_value < 0
disp('The number is negative.')
else
disp('The number is zero.')
end
```
#### 3.2.2 Loop Statements
Loop statements (such as `for` and `while`) allow us to repeatedly execute a block of code until a specific condition is met. For example, the following code uses a `while` loop to print numbers from 1 to 10:
```
% Initialize counter
i = 1;
% Loop to print numbers
while i <= 10
disp(i)
i = i + 1;
end
```
# 4.1 Logical Functions
### 4.1.1 any() and all()
MATLAB provides two logical functions, `any()` and `all()`, which are used to perform logical operations on elements in an array or matrix.
**any() Function**
The `any()` function returns a boolean value indicating whether there is at least one non-zero element in the array or matrix. If such an element exists, it returns `true`; otherwise, it returns `false`.
**Syntax:**
```
result = any(array)
```
**Parameters:**
* `array`: The array or matrix to be checked.
**Example:**
```
>> a = [1, 0, 3, 0, 5];
>> any(a)
ans = true
```
**all() Function**
The `all()` function returns a boolean value indicating whether all elements in the array or matrix are non-zero. If all elements are non-zero, it returns `true`; otherwise, it returns `false`.
**Syntax:**
```
result = all(array)
```
**Parameters:**
* `array`: The array or matrix to be checked.
**Example:**
```
>> b = [1, 2, 3, 4, 5];
>> all(b)
ans = true
```
### 4.1.2 find() and logical()
**find() Function**
The `find()` function returns a vector containing the indices of elements in the array or matrix that satisfy the specified condition.
**Syntax:**
```
indices = find(array, condition)
```
**Parameters:**
* `array`: The array or matrix to search.
* `condition`: The condition to be specified. Can be a relational operator, logical operator, or boolean expression.
**Example:**
```
>> c = [1, 3, 5, 7, 9];
>> indices = find(c > 5)
indices = [4, 5]
```
**logical() Function**
The `logical()` function converts the input to logical values. If the input is non-zero, it returns `true`; otherwise, it returns `false`.
**Syntax:**
```
result = logical(array)
```
**Parameters:**
* `array`: The array or matrix to be converted.
**Example:**
```
>> d = [0, 1, 2, 3, 4];
>> logical(d)
ans = [false, true, true, true, true]
```
# 5. 10 Application Scenarios of Logical Operators in MATLAB
Logical operators have a wide range of applications in MATLAB, from data validation to financial modeling, providing flexible and powerful tools for a variety of tasks. Here are 10 application scenarios of logical operators in MATLAB:
### 5.1 Data Validation
Logical operators can be used to verify if data meets specific conditions. For example, the following code checks if a number is positive:
```matlab
isPositive = x > 0;
```
### 5.2 Image Processing
Logical operators are very useful in image processing. For example, the following code uses logical operators to create a binary mask where white pixels indicate regions in the image with brightness above a threshold:
```matlab
mask = image > threshold;
```
### 5.3 Signal Processing
Logical operators can be used to manipulate signals. For example, the following code uses logical operators to find peaks in a signal:
```matlab
peaks = find(signal > mean(signal));
```
### 5.4 Numerical Computation
Logical operators can be used to perform numerical computations. For example, the following code uses logical operators to calculate the dot product of two vectors:
```matlab
dotProduct = sum(x .* y);
```
### 5.5 Machine Learning
Logical operators are crucial in machine learning. For example, the following code uses logical operators to build a simple binary classifier:
```matlab
predictions = (x > threshold) & (y < threshold);
```
### 5.6 Database Queries
Logical operators can be used to construct complex database queries. For example, the following code uses logical operators to retrieve records that meet specific conditions from a database:
```matlab
results = select * from table where (column1 > value1) & (column2 < value2);
```
### 5.7 Web Development
Logical operators can be used to control the flow in web applications. For example, the following code uses logical operators to check if a user is logged in:
```matlab
if (isLoggedIn)
// Display user content
else
// Redirect to login page
end
```
### 5.8 System Management
Logical operators can be used to automate system management tasks. For example, the following code uses logical operators to check if a server is running:
```matlab
if (isServerRunning)
// Perform maintenance tasks
else
// Send alerts
end
```
### 5.9 Game Development
Logical operators can be used to control the logic in games. For example, the following code uses logical operators to check if a player has collided with an enemy:
```matlab
if (player.position == enemy.position)
// Player dies
end
```
### 5.10 Financial Modeling
Logical operators can be used to build complex financial models. For example, the following code uses logical operators to calculate the risk of a portfolio:
```matlab
risk = (expectedReturn > threshold) & (standardDeviation < threshold);
```
0
0
相关推荐
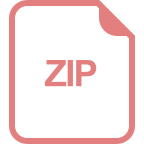
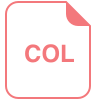
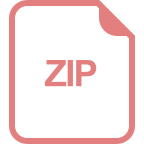
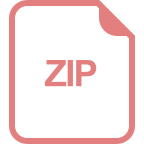
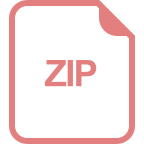
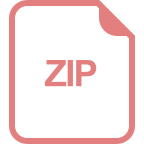
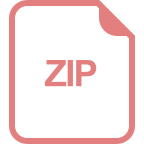
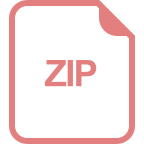