Conditional Data Processing in MATLAB: Filtering and Manipulating Data Based on Conditions (with 10 Real-World Examples)
发布时间: 2024-09-13 18:18:41 阅读量: 28 订阅数: 27 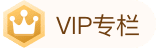
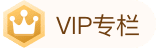
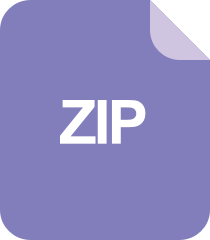
Conditional Nonparametric Kernel Density:Condtional Kernel Density Estimate with normal kernel and LSCV带宽选择-matlab开发
# Overview of Conditional Data Processing in MATLAB
Conditional data processing is a powerful feature in MATLAB that enables you to filter, manipulate, and analyze data based on specified conditions. By employing logical operators and conditional statements, you can create complex conditions to handle data that meets specific criteria.
Conditional data processing is beneficial in a wide array of applications, such as:
* Filtering specific values from large datasets
* Finding maximum and minimum values
* Conditional summation and averaging
* Extracting particular rows or columns
* Replacing or removing elements
* Creating conditional masks
* Visualizing and analyzing conditional data
# 2. Conditional Filtering
Conditional filtering is the process of extracting specific elements or subsets from data based on given conditions. MATLAB provides a wealth of conditional filtering tools, including logical operators and conditional statements.
### 2.1 Logical Operators
Logical operators are used to combine boolean values (true or false) to create more complex conditions.
#### 2.1.1 AND, OR, NOT
***AND (&&)**: The result is true only if all operands are true.
***OR (||)**: The result is true if at least one operand is true.
***NOT (~)**: Inverts the truth value; true becomes false, and false becomes true.
**Example:**
```matlab
a = [1 0 1 0];
b = [1 1 0 1];
% AND operation
c = a && b; % [1 0 0 0]
% OR operation
d = a || b; % [1 1 1 1]
% NOT operation
e = ~a; % [0 1 0 1]
```
#### 2.1.2 Logical Comparison Operators
Logical comparison operators are used to compare two values and return a boolean value.
| Operator | Description |
|---|---|
| == | Equal to |
| ~= | Not equal to |
| < | Less than |
| <= | Less than or equal to |
| > | Greater than |
| >= | Greater than or equal to |
**Example:**
```matlab
a = [1 2 3 4];
b = [2 3 4 5];
% Equal to operation
c = a == b; % [0 1 1 0]
% Not equal to operation
d = a ~= b; % [1 0 0 1]
% Greater than operation
e = a > b; % [0 0 0 0]
```
### 2.2 Conditional Statements
Conditional statements allow the execution of different code blocks based on conditions.
#### 2.2.1 if-else Statements
if-else statements execute different code blocks based on conditions.
**Syntax:**
```matlab
if condition
% Code to execute if condition is true
else
% Code to execute if condition is false
end
```
**Example:**
```matlab
a = 5;
if a > 10
disp('a is greater than 10')
else
disp('a is not greater than 10')
end
```
#### 2.2.2 switch-case Statements
switch-case statements execute different code blocks based on the value of a variable.
**Syntax:**
```matlab
switch variable
case value1
% Code to execute if variable equals value1
case value2
% Code to execute if variable equals value2
...
otherwise
% Code to execute if variable does not equal any specified values
end
```
**Example:**
```matlab
a = 'apple';
switch a
case 'apple'
disp('a is an apple')
case 'banana'
disp('a is a banana')
otherwise
disp('a is not an apple or a banana')
end
```
# 3. Conditional Operations
### 3.1 Element-wise Operations
#### 3.1.1 Indexing and Boolean Indexing
Indexing and boolean indexing allow you to select specific elements based on conditions.
**Indexing:**
```
result = data(logical_index)
```
***data:** Original data matrix
***logical_index:** Boolean index vector where True indicates the elements to be selected
**Boolean Indexing:**
```
result = data(data > threshold)
```
***data:** Original data matrix
***threshold:** Threshold value
**Code Block Logic Analysis:**
This code block uses boolean indexing to select all elements greater than the threshold value.
**Parameter Description:**
***data:** Input data matrix
***threshold:** Comparison threshold
***result:** New matrix containing the elements that meet the condition
#### 3.1.2 Element-wise Functions
Element-wise functions apply to each element of a data matrix, allowing you to perform operations based on conditions.
**Example:**
```
result = abs(data)
```
***abs:** Absolute value function
***data:** Original data matrix
**Code Bl
0
0