Optimizing Conditional Code in MATLAB: Enhancing Performance of Conditional Statements (with 15 Practical Examples)
发布时间: 2024-09-13 18:28:37 阅读量: 27 订阅数: 29 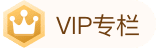
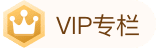
# 1. Overview of MATLAB Conditional Code Optimization
MATLAB conditional code optimization refers to the process of enhancing the efficiency and performance of conditional code by applying various techniques. Conditional code is used to execute different blocks of code based on specific conditions, and it is widely utilized in many MATLAB applications. Optimizing conditional code can significantly improve the speed of programs, especially when dealing with large datasets.
Conditional code optimization involves a variety of techniques, including vectorization, parallelization, Boolean indexing, logical indexing, short-circuit evaluation, and early termination. By applying these techniques, the number of loops, branches, and condition checks can be reduced, thereby enhancing the efficiency of the code.
# 2. Fundamentals of MATLAB Conditional Code Optimization
### 2.1 Basic syntax and usage of conditional statements in MATLAB
MATLAB's conditional statements are used to execute different blocks of code based on specific conditions. The basic syntax is as follows:
```matlab
if condition
execute code block 1
else
execute code block 2
end
```
Here, `condition` is a Boolean expression. If it is true, `code block 1` is executed; otherwise, `code block 2` is executed.
### 2.2 Common conditional operators in MATLAB
MATLAB provides a variety of conditional operators for comparing numerical values, strings, and logical values. The commonly used operators include:
| Operator | Description |
|---|---|
| == | Equal to |
| ~= | Not equal to |
| < | Less than |
| <= | Less than or equal to |
| > | Greater than |
| >= | Greater than or equal to |
| & | Logical AND |
| \| | Logical OR |
| ~ | Logical NOT |
### 2.3 Best practices for MATLAB conditional code optimization
To optimize MATLAB conditional code, the following best practices can be followed:
- **Use vectorized operations:** MATLAB supports vectorized operations, allowing the execution of operations on multiple elements within an array simultaneously. This can significantly improve the efficiency of conditional code.
- **Use parallelization:** MATLAB supports parallelization, allowing tasks to be assigned to multiple processors for simultaneous execution. This can further enhance the performance of conditional code.
- **Use Boolean indexing and logical indexing:** Boolean indexing and logical indexing enable the selection of elements within an array using a Boolean vector. This can simplify conditional code and improve efficiency.
- **Use short-circuit evaluation and early termination:** MATLAB supports short-circuit evaluation and early termination, preventing unnecessary calculations. This can improve the performance of conditional code, especially in complex conditional expressions.
**Code Examples:**
```matlab
% Using vectorized operations
x = rand(1000000, 1);
y = x > 0.5;
% Using parallelization
parfor i = 1:length(x)
if x(i) > 0.5
y(i) = true;
else
y(i) = false;
end
end
% Using Boolean indexing
x = rand(1000000, 1);
y = x > 0.5;
z = x(y);
% Using short-circuit evaluation and early termination
x = rand(1000000, 1);
y = x > 0.5 & x < 0.7;
```
**Logical Analysis:**
* The first code block uses vectorized operations to compare each element in `x` with 0.5 and store the results in `y`.
* The second code block utilizes parallelization to distribute the comparison tasks among multiple processors for parallel execution.
* The third code block uses Boolean indexing to select elements in `x` that are greater than 0.5 and store them in `z`.
* The fourth code block employs short-circuit evaluation and early termination, where the expression `x < 0.7` will not be evaluated if `x` is greater than 0.5.
# 3.1 Vectorization and Parallelization of Conditional Code in MATLAB
**Vectorization**
Vectorization is a technique that converts loop operations into vector or matrix operations, significantly enhancing the execut
0
0
相关推荐


