Common Problems and Solutions for Conditional Judgments in MATLAB: Troubleshooting Guide (with 20 Real Cases)
发布时间: 2024-09-13 18:14:54 阅读量: 23 订阅数: 18 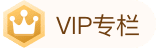
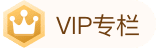
# Common Issues and Solutions for Conditional Statements in MATLAB: A Troubleshooting Guide (with 20 Real Cases)
## 1. Basic Principles of Conditional Statements in MATLAB
Conditional statements in MATLAB are powerful tools used to execute or skip blocks of code based on specific conditions. The basic principle of conditional statements involves using logical expressions to evaluate whether a condition is true or false. If the condition is true, the specified block of code is executed; if false, the block is skipped.
Logical expressions are composed of logical operators (such as `&&`, `||`, and `~`) and comparison operators (like `==`, `>`, and `<`). These operators allow you to combine multiple conditions and execute corresponding blocks of code based on their truth values.
## ***mon Problems with Conditional Statements
### 2.1 Precedence and Associativity of Logical Operators
#### 2.1.1 Precedence of Logical Operators
Logical operators in MATLAB follow a precedence order, from highest to lowest, as shown below:
| Operator | Precedence |
|---|---|
| `~` (NOT) | 1 |
| `&` (AND) | 2 |
| `|` (OR) | 3 |
For instance, in the expression `a & b | c`, the `&` operator has higher precedence than the `|` operator, so `a & b` will be evaluated first, and then the result will be combined with `c` using the `|` operator.
#### 2.1.2 Associativity of Logical Operators
Logical operators also have associativity, which determines whether they are evaluated from left to right or right to left, depending on the operator's associativity.
| Operator | Associativity |
|---|---|
| `~` (NOT) | Right-to-left |
| `&` (AND) | Left-to-right |
| `|` (OR) | Left-to-right |
For example, in the expression `a & b & c`, the `&` operator is left-associative, so `a & b` will be evaluated first, and then the result will be combined with `c` using the `&` operator.
### 2.2 Evaluation Order of Conditional Expressions
#### 2.2.1 Short-Circuit Evaluation
Logical operators in MATLAB support short-circuit evaluation, which means that when the result of an operator is sufficient to determine the overall result of the expression, the evaluation of subsequent operators will be stopped.
For instance, in the expression `a & b`, if `a` is false, then `b` does not need to be evaluated, as the result of `a & b` will always be false.
#### 2.2.2 Forced Evaluation
Sometimes, it is necessary to force the evaluation of all operators, even if their result does not affect the overall result of the expression. The `&&` and `||` operators can be used to achieve forced evaluation.
For example, in the expression `a && b`, even if `a` is false, `b` will be forced to evaluate.
### 2.3 Undefined Variables or NaN Values
#### 2.3.1 Handling Undefined Variables
Undefined variables in MATLAB are considered false. Therefore, using an undefined variable in a conditional expression will result in the expression being false.
For example, if variable `a` is undefined, the
0
0
相关推荐
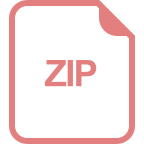
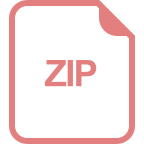
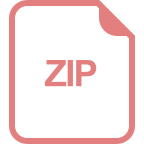
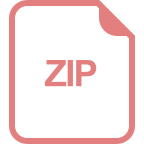
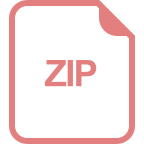
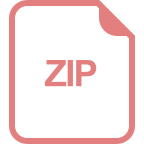
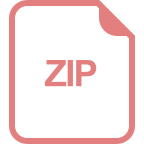
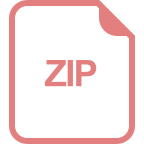