Clever Use of Boolean Variables in MATLAB: Flexible Tools for Conditional Judgment with 15 Application Scenarios
发布时间: 2024-09-13 18:14:06 阅读量: 19 订阅数: 23 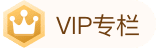
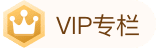
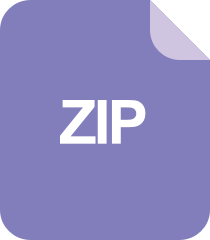
sghmc-matlab:随机梯度哈密顿蒙特卡罗的 Matlab 实现
# Clever Application of Boolean Variables in MATLAB: A Flexible Tool for Conditional Judgement (with 15 Scenarios)
## 1. The Basics of Boolean Variables in MATLAB
Boolean variables are a data type in MATLAB used to represent truth values, possessing only two possible values: `true` (true) and `false` (false). They are commonly employed to control program flow, perform conditional judgements, and handle logical operations.
The syntax for creating a boolean variable is:
```matlab
variable_name = logical(value);
```
Here, `variable_name` is the name of the boolean variable you wish to create, and `value` is the truth value to be assigned to that variable. For example:
```matlab
is_valid = true;
```
## 2. Application of Boolean Variables in Conditional Judgement
### 2.1 Logical Operations of Boolean Variables
Boolean variables support logical operations, including AND, OR, and NOT. These operators can combine two or more boolean variables to produce a new boolean value.
- **AND operator (&&):** The result is true if both boolean variables are true; otherwise, it is false.
- **OR operator (||):** The result is true if at least one of the boolean variables is true; otherwise, it is false.
- **NOT operator (~):** Inverts the boolean value, turning true to false and false to true.
**Code Block:**
```matlab
% Define boolean variables
x = true;
y = false;
% AND operation
z = x && y; % z = false
% OR operation
w = x || y; % w = true
% NOT operation
v = ~x; % v = false
```
**Logical Analysis:**
* `x && y`: Since `x` is true and `y` is false, the result `z` is false.
* `x || y`: Since `x` is true, the result `w` is true.
* `~x`: Inverts `x`, so the result `v` is false.
### 2.2 Conditional Expressions with Boolean Variables
Conditional expressions are a syntactic structure that uses boolean variables for conditional judgement. They allow different code blocks to be executed based on the value of a boolean variable.
**Syntax:**
```
if conditional expression
execute code block 1
else
execute code block 2
end
```
**Code Block:**
```matlab
% Define boolean variable
is_valid = true;
% Conditional expression
if is_valid
% Execute code block 1
disp('Input is valid')
else
% Execute code block 2
disp('Input is invalid')
end
```
**Logical Analysis:**
* Since `is_valid` is true, code block 1 is executed, displaying "Input is valid".
* If `is_valid` were false, code block 2 would be executed, displaying "Input is invalid".
**Nested Conditional Expressions:**
Conditional expressions can be nested to handle more complex conditions.
**Code Block:**
```matlab
% Define boolean variables
is_valid = true;
is_positive = true;
% Nested conditional expression
if is_valid
if is_positive
% Execute code block 1
disp('Input is valid and positive')
else
% Execute code block 2
disp('Input is valid but negative')
end
else
% Execute code block 3
disp('Input is invalid')
end
```
**Logical Analysis:**
* Since `is_valid` is true, the outer conditional expression is executed.
* Since `is_positive` is also true, the inner conditional expression is executed, displaying "Input is valid and positive".
* If `is_positive` were false, the else code block of the inner conditional expression would be executed, displaying "Input is valid but negative".
* If `is_valid` were false, the else code block of the outer conditional expression would be executed, displaying "Input is invalid".
## 3. Application of Boolean Variables in Array and Matrix Operations
Boolean variables in MATLAB are not limited to conditional judgement; they are also widely used in array and matrix operations, providing powerful tools for data processing and analysis. This chapter will delve into the application of boolean variables in array and matrix operations, including boolean indexing, logical operations, and matrix operations.
### 3.1 Boolean Indexing and Logical Operations
Boolean indexing is a technique that uses boolean variables to select specific elements from an array or matrix. The syntax for boolean indexing is as follows:
```matlab
array_or_matrix(logical_vector)
```
Where:
* `array_or_matrix` is the array or matrix to be indexed.
* `logical_vector` is a boolean vector with a length equal to the number of rows or columns of `array_or_matrix`.
The principle of boolean indexing is that each element of the boolean vector corresponds to an element of `array_or_matrix`. If the element of the boolean vector is true, the corresponding element is selected.
**Example:**
```matlab
A = [1 2 3; 4 5 6; 7 8 9];
logical_vector = [true
```
0
0
相关推荐
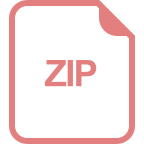
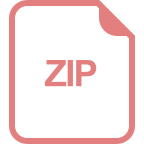
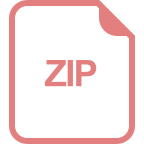
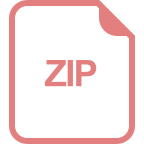
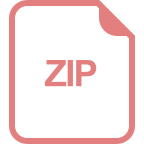
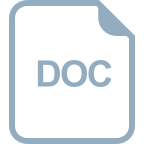
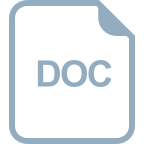
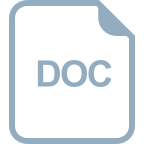