MATLAB Crash Prevention Through Code Refactoring: Enhancing Maintainability and Stability
发布时间: 2024-09-13 14:32:05 阅读量: 30 订阅数: 40 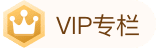
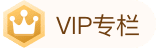
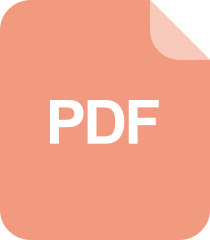
云计算之云计算安全技术:Intrusion Detection and Prevention Systems (IDPS):云计算安全概论.pdf
# Strategies for Refactoring MATLAB Code: Enhancing Maintainability and Stability to Prevent Crashes
## 1. Understanding the Roots and Analysis of MATLAB Crashes
Crashes in MATLAB are a common error that greatly inconvenience users. To solve the crash problem, it is first necessary to understand its roots.
MATLAB crashes are typically caused by the following reasons:
- **Memory Leaks:** When MATLAB programs fail to release memory that is no longer in use, memory leaks occur. This leads to a continuous increase in memory usage, ultimately resulting in a crash.
- **Stack Overflow:** When MATLAB programs call too many nested functions or use recursion, a stack overflow occurs. This results in the program being unable to allocate enough memory to store function call information, causing a crash.
## 2. Code Refactoring Strategies: Improving Maintainability and Stability
### 2.1 Optimization of Code Structure
#### 2.1.1 Modular Design
Modular design is a strategy that organizes code into independent, reusable modules. By breaking down the code into smaller, manageable units, maintainability is improved, and complexity is reduced.
**Code Block:**
```matlab
% Define a sum function
function sum = calculateSum(numbers)
sum = 0;
for i = 1:length(numbers)
sum = sum + numbers(i);
end
end
```
**Logical Analysis:**
This code implements the sum function, which iterates through the given list of numbers and accumulates their total.
**Parameter Description:**
* `numbers`: The list of numbers to be summed.
**Optimization Approach:**
By encapsulating the sum logic into an independent function, the code's reusability is enhanced. If the sum needs to be calculated in different contexts, this function can be easily called without having to rewrite the same code.
#### 2.1.2 Dependency Management
Dependency management refers to managing dependencies between code modules. Good dependency management can prevent circular dependencies and improve code maintainability.
**Code Block:**
```matlab
% Define a function that depends on the calculateSum function
function calculateAverage(numbers)
sum = calculateSum(numbers);
average = sum / length(numbers);
end
```
**Logical Analysis:**
The `calculateAverage` function depends on the `calculateSum` function to calculate the sum of the number list.
**Parameter Description:**
* `numbers`: The list of numbers for which the average is to be calculated.
**Optimization Approach:**
By clearly defining dependencies, circular dependencies can be avoided. For example, if the `calculateSum` function also depended on the `calculateAverage` function, it would result in a circular dependency, leading to unstable code.
### 2.2 Improving Error Handling Mechanisms
#### 2.2.1 Exception Handling
Exception handling is a mechanism for dealing with errors or exceptional situations that occur during code execution. By capturing and handling exceptions, code stability is improved, and crashes are prevented.
**Code Block:**
```matlab
try
% Execute code that may cause an exception
catch exception
% Handle the exception
end
```
**Logical Analysis:**
The `try-catch` statement is used to capture and handle exceptions. If the code in the `try` block causes an exception, control is transfe
0
0
相关推荐







