The Truth Behind MATLAB Crashes: The Game of Memory Management and Resource Allocation, Revealing Optimization Strategies
发布时间: 2024-09-13 14:15:32 阅读量: 40 订阅数: 40 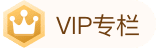
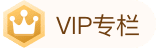
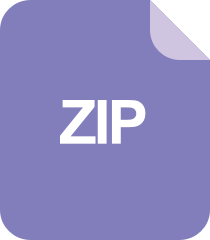
Chicago_Car_Crashes:芝加哥车祸
# 1. Overview of MATLAB Crashes
A MATLAB crash refers to the sudden termination of the MATLAB application during operation, usually manifested by the closing of the program window or the appearance of error messages. The causes of crashes can be multifaceted, including insufficient memory, improper resource allocation, and coding errors.
This chapter will outline the common causes of MATLAB crashes, laying the foundation for in-depth analysis and optimization strategies in subsequent chapters.
# 2. The Game of Memory Management and Resource Allocation
One of the primary reasons for MATLAB crashes is improper memory management and resource allocation. This section will delve into MATLAB's memory management mechanisms and resource allocation strategies, analyzing how insufficient memory and improper resource allocation lead to crashes and providing optimization strategies to avoid these issues.
### 2.1 MATLAB Memory Management Mechanisms
MATLAB employs a dynamic memory management mechanism, meaning that memory is automatically allocated and released as needed during program execution. The MATLAB memory space is primarily divided into the following areas:
- **Heap Space:** Used for storing dynamically allocated data, such as variables, arrays, and objects.
- **Stack Space:** Used for storing function call information, local variables, and temporary data.
- **Global Space:** Used for storing global variables and constants.
#### 2.1.1 Memory Space Allocation
MATLAB allocates heap space using the `malloc` function and releases heap space using the `free` function. When creating variables or arrays, MATLAB automatically allocates memory from the heap space. The size of the allocated memory depends on the data type and size of the variable or array.
```matlab
% Allocate a 1000x1000 double precision floating-point array
A = rand(1000, 1000);
% View the size of the allocated memory
memory
```
#### 2.1.2 Memory Recycling Mechanism
MATLAB uses a reference counting mechanism to manage memory recycling. When a variable or array is no longer referenced by any other variables or arrays, its reference count becomes 0, and MATLAB automatically releases the memory it occupies.
```matlab
% Create a variable and increase its reference count to 2
a = 1;
b = a;
% View the reference count of a
whos a
% Delete the reference to a, decreasing its reference count to 1
clear a
% View the reference count of a
whos a
% Delete the reference to b, decreasing the reference count of a to 0, MATLAB releases the memory occupied by a
clear b
% View the reference count of a
whos a
```
### 2.2 Resource Allocation Strategies
In addition to memory management, MATLAB also offers resource allocation strategies to manage the creation and destruction of variables, arrays, and objects. These strategies help optimize memory usage and prevent resource leaks.
#### 2.2.1 Variable Allocation and Release
Variables are allocated memory when created and released when destroyed. MATLAB uses the `assignin` function to allocate variables and the `clear` function to release variables.
```matlab
% Allocate a variable and view its memory usage
assignin('base', 'x', 1);
memory
% Release the variable and view its memory usage
clear x
memory
```
#### 2.2.2 Array Allocation and Release
Arrays are allocated memory when created and released when destroyed. MATLAB uses functions such as `zeros`, `ones`, `rand` to allocate arrays and the `clear` function to release arrays.
```matlab
% Allocate a 1000x1000 double precision floating-point array and view its memory usage
A = zeros(1000, 1000);
memory
% Release the array and view its memory usage
clear A
memory
```
#### 2.2.3 Object Allocation and Release
Objects are allocated memory when created and released when destroyed. MATLAB uses the `class` function to allocate objects and the `delete` function to release objects.
```matlab
% Create an object and view its memory usage
obj = class('MyClass');
memory
% Release the object and view its memory usage
delete(obj)
memory
```
# 3. Crash Cause Analysis and Optimization Strategies
### 3.1 Crashes Caused by Insufficient Memory
#### 3.1.1 Variables or Arrays Too Large
When the memory space allocated for variables or arrays exceeds the available memory in MATLAB, crashes due to insufficient memory occur. This is usually caused by the following reasons:
- **Variables Too Large:** Variables may become very large when storing large data structures or images.
- **Arrays Too Large:** Arrays may become very large when creating arrays with a large number of elements.
#### 3.1.2 Memory Leakage
Memory leakage refers to MATLAB's inability to release memory that is no longer in use. This can cause memory usage to continuously increase and eventually lead to crashes. Memory leakage is usually caused by the following reasons:
- **Variables Not Released:** Variables are not released after use, preventing MATLAB from reclaiming their memory.
- **Arrays Not Released:** Arrays are not released after use, preventing MATLAB from reclaiming their memory.
- **Objects Not Released:** Objects are not released after use, preventing MATLAB from reclaiming their memory.
### 3.2 Crashes Caused by Improper Resource Allocation
#### 3.2.1 Variables Not Released
When variables are no longer needed, not releasing them can waste memory and potentially lead to crashes. This is usually caused by the following reasons:
- **Forget to Release Variables:** Forget to use the `clear` or `delete` command to release variables after they are no longer needed.
- **Variables Not Released in Loops:** Variables are created in loops without being released at the end of the loop.
#### 3.2.2 Arrays Not Released
When arrays are no longer needed, not releasing them can waste memory and potentially lead to crashes. This is usually caused by the following reasons:
- **Forget to Release Arrays:** Forget to use the `clear` or `delete` command to release arrays after they are no longer needed.
- **Arrays Not Released in Loops:** Arrays are created in loops without being released at the end of the loop.
#### 3.2.3 Objects Not Released
When objects are no longer needed, not releasing them can waste memory and potentially lead to crashes. This is usually caused by the following reasons:
- **Forget to Release Objects:** Forget to use the `delete` command to release objects after they are no longer needed.
- **Objects Not Released in Loops:** Objects are created in loops without being released at the end of the loop.
### 3.3 Optimization Strategies
#### 3.3.1 Optimize Memory Usage
- **Use Appropriate Data Types:** Choose the smallest data type that is most suitable for storing data, such as using `int8` instead of `double`.
- **Avoid Creating Unnecessary Variables:** Only create variables that are absolutely necessary and ensure they are released when no longer in use.
- **Use Sparse Matrices:** For matrices with a large number of zero elements, using sparse matrices can save memory.
- **Use Memory-Mapped Files:** For very large datasets, using memory-mapped files can avoid loading the entire dataset into memory.
#### 3.3.2 Optimize Resource Allocation
- **Use `clear` and `delete` Commands:** Release variables, arrays, or objects when no longer needed using `clear` and `delete` commands.
- **Use `try-catch` Blocks:** Use `try-catch` blocks when allocating resources to release them in case of errors.
- **Use Object Pools:** For objects that are frequently created and destroyed, using object pools can reduce the overhead of creating and destroying objects.
# 4. Practices in MATLAB Memory Management and Resource Allocation
This section will introduce practical tools and best practices for memory management and resource allocation in MATLAB, helping you effectively manage memory and resources in MATLAB and avoid crash issues.
### 4.1 Memory Management Tools
MATLAB provides various tools to help you analyze and optimize memory usage, including:
- **Memory Analyzer:** Allows you to view the allocation of memory in the MATLAB workspace and identify potential memory leaks or other memory issues.
- **Memory Optimization Tools:** Offers a set of tools to optimize MATLAB memory usage, such as clearing the workspace, compressing variables, and releasing objects.
### 4.2 Best Practices for Resource Allocation
#### 4.2.1 Variable Allocation and Release
- Create variables only when needed and release variables that are no longer in use promptly.
- Use the `clear` command to release variables, or use the `delete` command to release objects.
- Avoid using global variables, as they will remain in memory.
#### 4.2.2 Array Allocation and Release
- Allocate only the necessary array size and release arrays that are no longer in use promptly.
- Use `zeros` or `ones` functions to create arrays rather than using `[]`.
- Use the `clear` command to release arrays, or use the `delete` command to release object arrays.
#### 4.2.3 Object Allocation and Release
- Create objects only when needed and release objects that are no longer in use promptly.
- Use the `delete` command to release objects.
- Use object pools to manage objects to improve performance and reduce memory usage.
#### Code Example:
```
% Create a large array
largeArray = randn(10000, 10000);
% Use the memory analyzer to view memory usage
memory
% Release the array
clear largeArray
% View memory usage again
memory
```
#### Code Logic Analysis:
- The first line creates a 10000x10000 random array, which allocates a significant amount of memory.
- The second line uses the memory analyzer to view the current memory usage.
- The third line releases the array, freeing the memory allocated to it.
- The fourth line uses the memory analyzer again to view memory usage, showing a significant reduction in memory usage after releasing the array.
#### Parameter Description:
- `randn(m, n)`: Creates an m-by-n random matrix with elements drawn from a normal distribution.
- `memory`: Displays information about memory allocation and usage in the MATLAB workspace.
- `clear`: Releases variables or arrays.
# 5. Prevention and Debugging of MATLAB Crashes
### 5.1 Preventive Measures
#### 5.1.1 Monitor Memory Usage
* Use the MATLAB Memory Analyzer (MAT) to monitor memory usage.
* MAT provides real-time memory usage information, including variable size, type, and allocation location.
* Regularly check MAT to identify potential memory issues.
#### 5.1.2 Optimize Resource Allocation
* Follow best practices for resource allocation (see Section 4.2).
* Avoid allocating overly large variables or arrays.
* Release variables, arrays, and objects that are no longer in use promptly.
### 5.2 Debugging Techniques
#### 5.2.1 Using a Debugger
* Use the MATLAB Debugger (dbstop) to set breakpoints.
* Set breakpoints in the code to pause execution when specific conditions are met.
* Check variable values and memory usage to identify problems.
#### 5.2.2 Analyzing Memory Usage
* Use the MATLAB Memory Analyzer (MAT) to analyze memory usage.
* Identify variables or arrays that occupy a significant amount of memory.
* Check the type and allocation location of variables to understand memory usage patterns.
#### 5.2.3 Analyzing Resource Allocation
* Use the MATLAB Memory Analyzer (MAT) to analyze resource allocation.
* Identify variables, arrays, or objects that have not been released.
* Check if the allocated resources are still in use and release those that are no longer needed promptly.
0
0
相关推荐








