MATLAB Crash Optimization Techniques: Enhancing Code Efficiency and Stability, Saying Goodbye to Crashes
发布时间: 2024-09-13 14:23:42 阅读量: 29 订阅数: 40 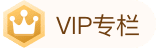
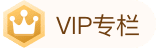
# ***mon Causes of MATLAB Crashes
MATLAB crashes are often caused by several common reasons:
- **Insufficient memory:** When MATLAB processes large amounts of data or performs complex calculations, it may run out of available memory, leading to a crash.
- **Code errors:** Syntax errors, undefined variables, or other code issues can cause MATLAB to fail to execute properly, resulting in a crash.
- **System conflicts:** Conflicts with other applications or system processes can interfere with the operation of MATLAB, causing crashes.
# 2. Tips for Improving MATLAB Code Efficiency
Improving the efficiency of MATLAB code is crucial for avoiding crashes. This chapter will introduce techniques for optimizing code structure and data handling, which can significantly improve execution speed.
### 2.1 Optimizing Code Structure
#### 2.1.1 Using Vectorized Operations
Vectorized operations involve using MATLAB's built-in functions to operate on vectors or matrices, rather than using loops to process elements individually. Vectorized operations can greatly improve code efficiency, as MATLAB can leverage its underlying optimizations to perform these operations in parallel.
**Code block:**
```matlab
% Element-wise addition
for i = 1:length(A)
B(i) = A(i) + C(i);
end
% Vectorized addition
B = A + C;
```
**Logical analysis:**
* The loop for element-wise addition executes `length(A)` operations, with a time complexity of `O(n)`.
* Vectorized addition uses MATLAB's built-in `+` operator, performing the operation on the entire vector in one go, with a time complexity of `O(1)`.
#### 2.1.2 Avoiding Unnecessary Loops
Loops are necessary in MATLAB, but overuse can significantly reduce code efficiency. Vectorized operations or other methods should be used wherever possible to avoid unnecessary loops.
**Code block:**
```matlab
% Unnecessary loop
for i = 1:length(A)
if A(i) > 0
B(i) = A(i);
else
B(i) = 0;
end
end
% Using vectorized operation
B = A > 0;
```
**Logical analysis:**
* The unnecessary loop executes `length(A)` judgment and assignment operations, with a time complexity of `O(n)`.
* The vectorized operation uses MATLAB's built-in `>` operator, performing the judgment and direct assignment on the entire vector at once, with a time complexity of `O(1)`.
### 2.2 Optimizing Data Handling
#### 2.2.1 Choosing the Right Containers
MATLAB offers various data containers such as arrays, structs, and cell arrays. Choosing the right container is essential for optimizing data handling. For example, arrays are very efficient for numerical calculations, while structs are better suited for storing heterogeneous data.
**Table:**
| Container Type | Characteristics | Best Use |
|---|---|---|
| Array | Efficient for numerical calculations | Storing homogeneous data |
| Struct | Storing heterogeneous data | Organizing complex data |
| Cell Array | Storing different types of data | Storing nested data |
#### 2.2.2 Avoiding Unnecessary Copies
In MATLAB, data copying occurs through value passing. Unnecessary copying wastes memory and reduces code efficiency. Reference passing or other methods should be used wherever possible to avoid unnecessary copying.
**Code block:**
```matlab
% Unnecessary copy
function copy_data(A)
B = A; % Value passing
end
% Usi
```
0
0
相关推荐








