The Ultimate Solution to MATLAB Crashes: Optimize Code and Environment Settings for a Stable Operating Environment
发布时间: 2024-09-13 14:16:56 阅读量: 42 订阅数: 40 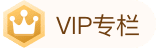
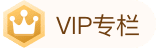
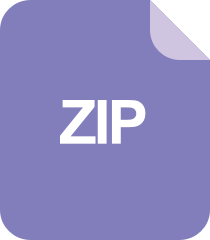
Chicago_Car_Crashes:芝加哥车祸
# Ultimate Solutions for MATLAB Crashes: Optimizing Code and Environment Configuration for Stable Operation
## 1. Analysis of Causes for MATLAB Crashes
MATLAB crashes are a common issue that can be caused by a variety of factors. Understanding these causes is crucial for solving and preventing crashes.
**1. Insufficient Memory**
MATLAB is a memory-intensive application that requires a large amount of memory to process data and perform computations. When available memory is insufficient, MATLAB may crash.
**2. Code Errors**
Syntax errors, logical errors, and runtime errors can all lead to MATLAB crashes. These errors can interrupt code execution and cause the application to crash.
**3. Hardware Issues**
Hardware problems, such as memory faults or graphics card issues, can also cause MATLAB crashes. These issues can affect MATLAB's interaction with system resources, resulting in instability.
## 2. Optimizing MATLAB Code
Optimizing MATLAB code is essential for improving the performance of MATLAB programs. This chapter introduces several effective methods for optimizing MATLAB code, including avoiding memory leaks, optimizing algorithms and data structures, as well as debugging and error handling.
### 2.1 Avoiding Memory Leaks
Memory leaks refer to a program's inability to release memory that is no longer in use, causing memory usage to continually increase until the program crashes. Avoiding memory leaks is crucial, and the following measures can be taken:
#### 2.1.1 Using Appropriate Data Types
Choosing appropriate data types can effectively reduce memory usage. For instance, using the `logical` type instead of the `double` type for boolean values can save half the memory space.
```matlab
% Using logical type
a = logical([1, 0, 1]);
% Using double type
b = double([1, 0, 1]);
% Comparing memory usage
whos a
whos b
```
#### 2.1.2 Correctly Releasing Variables and Objects
Variables and objects should be released promptly when they are no longer needed to free up memory. The `clear` and `delete` commands can be used to release variables and objects.
```matlab
% Creating an object
obj = MyClass();
% Using the object
% ...
% Releasing the object
delete(obj);
```
### 2.2 Optimizing Algorithms and Data Structures
The choice of algorithms and data structures has a significant impact on program performance. Here are some optimization suggestions:
#### 2.2.1 Choosing the Right Algorithms and Data Structures
Selecting the appropriate algorithms and data structures can significantly improve program efficiency. For example, using a hash table for lookup operations is more efficient than linear search.
| Data Structure | Lookup Time Complexity |
|---|---|
| Linear Search | O(n) |
| Hash Table | O(1) |
#### 2.2.2 Avoiding Unnecessary Loops and Calculations
Unnecessary loops and calculations waste time and resources. Code should be carefully examined to avoid repetitive or unnecessary computations.
```matlab
% Unnecessary loop
for i = 1:100
% ...
end
% Optimized code
for i = 1:10:100
% ...
end
```
### 2.3 Debugging and Error Handling
Debugging and error handling are essential for identifying and resolving issues in the program. MATLAB provides powerful debugging and error handling tools, including:
#### 2.3.1 Using Breakpoints and Debuggers
Breakpoints and debuggers can help execute programs step by step, examine variable values, and identify errors.
```matlab
% Setting breakpoints
setdbstops('myFunction');
% Running the program
run myFunction
```
#### 2.3.2 Catching and Handling Errors
Catching and handling errors can prevent program crashes and allow the program to recover gracefully when an error occurs.
```matlab
try
% Code block
catch err
% Error handling code
end
```
## 3.1 Hardware Optimization
#### 3.1.1 Ensuring Sufficient Memory and CPU Resources
MATLAB is a memory-intensive application that requires a large amount of memory to store data and intermediate computation results. When memory is insufficient, MATLAB may experience crashes or slow performance. Therefore, ensuring that the computer has enough memory is crucial for optimizing MATLAB performance.
Generally, for most MATLAB tasks, it is recommended to use at least 8GB of memory. For large datasets or complex computations, 16GB or more may be required. The following command can be used to check the memory usage of the computer:
```
>> memory
```
This command will display the available memory, used memory, and total memory of the computer.
In addition to memory, MATLAB also has high CPU resource requirements. Multi-core processors can significantly improve MATLAB performance because MATLAB can distribute computation tasks across multiple cores for parallel execution. It is recommended to use a CPU with at least 4 cores.
#### 3.1.2 Using Solid State Drives (SSD)
Solid state drives (SSD) have faster read and write speeds than traditional hard drives (HDD). Using an SSD can significantly reduce the time MATLAB takes to load data and save results, ***
***pared to HDD, the advantages of SSD include:
***Faster read and write speeds:** SSDs can be several orders of magnitude faster than HDDs.
***Lower access latency:** SSDs have much lower access latency, meaning MATLAB can access data more quickly.
***Higher reliability:** SSDs have no moving parts and are therefore more durable than HDDs.
If budget allows, it is strongly recommended to use an SSD to optimize MATLAB performance.
## 4. Advanced MATLAB Optimization Techniques
### 4.1 Parallel Computing
#### 4.1.1 Utilizing Multi-core Processors
Modern computers are usually equipped with multi-core processors, each of which can independently execute tasks. MATLAB can leverage this parallelism to increase computational speed.
```matlab
% Creating a parallel pool
parpool;
% Parallel computing a loop
parfor i = 1:1000000
% Perform some computation
end
% Closing the parallel pool
delete(gcp);
```
**Parameter Explanation:**
* `parpool`: Creates a parallel pool, specifying the number of cores to use.
* `parfor`: Creates a parallel loop, distributing the tasks in the loop body to the cores in the parallel pool.
* `delete(gcp)`: Closes the parallel pool, releasing the resources used.
**Logical Analysis:**
This code uses the Parallel Computing Toolbox to create a parallel pool, specifying the use of all available cores. Then, it creates a parallel loop that distributes the tasks in the loop body to the cores in the parallel pool. Finally, it closes the parallel pool, releasing the resources used.
#### 4.1.2 Using the Parallel Computing Toolbox
The MATLAB Parallel Computing Toolbox provides more advanced parallel programming features, such as parallel arrays and parallel algorithms.
```matlab
% Creating a parallel array
A = parallel.array(1:1000000);
% Using parallel array for parallel computation
A = A + 1;
% Getting the results of the parallel array
result = gather(A);
```
**Parameter Explanation:**
* `parallel.array`: Creates a parallel array, distributing data across the cores in the parallel pool.
* `gather`: Collects the results of the parallel array into a local array.
**Logical Analysis:**
This code uses the Parallel Computing Toolbox to create a parallel array, distributing the data across the cores in the parallel pool. Then, it performs parallel computation using the parallel array, and finally collects the results into a local array.
### 4.2 GPU Acceleration
#### 4.2.1 Understanding GPU Parallel Programming
Graphics Processing Units (GPUs) are hardware specifically designed for parallel computing. MATLAB supports GPU parallel programming, which can significantly improve the performance of applications involving large amounts of data parallel computing.
#### 4.2.2 Using the MATLAB GPU Computing Toolbox
The MATLAB GPU Computing Toolbox provides functions and tools for GPU parallel programming.
```matlab
% Creating a GPU array
A = gpuArray(1:1000000);
% Using GPU array for parallel computation
A = A + 1;
% Copying the results of the GPU array to the CPU
result = gather(A);
```
**Parameter Explanation:**
* `gpuArray`: Creates a GPU array, transferring data to the GPU.
* `gather`: Copies the results of the GPU array to a CPU array.
**Logical Analysis:**
This code uses the MATLAB GPU Computing Toolbox to create a GPU array, transferring data to the GPU. Then, it performs parallel computation using the GPU array, and finally copies the results to a CPU array.
### 4.3 Code Generation and Deployment
#### 4.3.1 Compiling MATLAB Code into Executable Files
MATLAB Compiler can compile MATLAB code into standalone executable files that can run without a MATLAB installation. This can enhance the speed and security of deploying MATLAB applications.
```matlab
% Compiling MATLAB code into an executable file
mcc -m my_function.m
```
**Parameter Explanation:**
* `mcc`: MATLAB Compiler command.
* `-m`: Specifies the main function to be compiled.
**Logical Analysis:**
This command uses MATLAB Compiler to compile the `my_function.m` file into an executable file named `my_function.exe`.
#### 4.3.2 Deploying MATLAB Applications
The MATLAB Application Deployment Toolbox can package MATLAB applications into standalone installers that can be deployed on various platforms. This simplifies the deployment and distribution of MATLAB applications.
```matlab
% Creating a MATLAB application
app = matlab.apps.new('my_app');
% Deploying a MATLAB application
deploytool(app);
```
**Parameter Explanation:**
* `matlab.apps.new`: Creates a new MATLAB application.
* `deploytool`: Opens the MATLAB Application Deployment Toolbox.
**Logical Analysis:**
This code uses the MATLAB Application Deployment Toolbox to create a new MATLAB application and then opens the deployment toolbox to deploy the application.
## 5. MATLAB Crash Troubleshooting
### 5.1 Log File Analysis
When MATLAB crashes, log files are usually generated, containing detailed information about the error. These log files are crucial for identifying and resolving crash issues.
#### 5.1.1 Finding MATLAB Log Files
MATLAB log files are typically located in the following directories:
* Windows: `C:\Users\<username>\AppData\Roaming\MathWorks\MATLAB\<version>\MATLAB.log`
* macOS: `/Users/<username>/Library/Logs/MATLAB/<version>/MATLAB.log`
* Linux: `/home/<username>/MATLAB/<version>/MATLAB.log`
#### 5.1.2 Analyzing Error Information in Log Files
MATLAB log files contain the following types of error information:
***Error Messages:** Short descriptions of the errors.
***Stack Traces:** Show the sequence of function calls that led to the error.
***Additional Information:** May include additional details about the error, such as variable values or memory usage.
To analyze the log files, follow these steps:
1. Open the log file.
2. Look for the error message corresponding to the crash.
3. Check the stack trace to understand the sequence of function calls that led to the error.
4. Analyze the additional information to get more context about the error.
### 5.2 Contacting the MATLAB Support Team
If the issue cannot be resolved through log file analysis, contact the MATLAB support team.
#### 5.2.1 Submitting an Error Report
MATLAB provides an error reporting tool that allows users to submit detailed information about crashes. To submit an error report, follow these steps:
1. Open MATLAB.
2. Go to the "Help" menu.
3. Select "Report a Problem."
4. Fill out the error report form, including the following information:
* Error Message
* Stack Trace
* Any other relevant information
5. Click the "Submit" button.
#### 5.2.2 Getting Technical Support
In addition to submitting an error report, technical support from the MATLAB support team can be obtained through the following means:
***Online Support:** Visit the MATLAB support website (***
***
***
***
***
***
***'s official team regularly releases new versions that include bug fixes, performance optimizations, and new features. Updating MATLAB versions regularly ensures the use of the latest and most stable version, reducing the likelihood of crashes.
**Operating Steps:**
1. Open MATLAB and click on the "Help" tab in the top menu bar.
2. Select the "Check for Updates" option.
3. If updates are available, follow the prompts to update.
### 6.1.2 Regularly Cleaning Up Temporary Files and Caches
MATLAB generates a large number of temporary files and caches during operation, which can occupy a lot of memory and lead to crashes. Regularly cleaning up these files can free up memory and improve the stability of MATLAB.
**Operating Steps:**
1. Open the MATLAB command window.
2. Enter the following command:
```matlab
delete(matlabroot, 'local', '*.mat');
```
3. Wait for the command to complete, as the cleaning process may take some time.
0
0
相关推荐







