MATLAB Crash Debugging Secrets: Skillfully Utilizing Tools and Techniques to Rapidly Identify the Root Cause
发布时间: 2024-09-13 14:24:37 阅读量: 33 订阅数: 32 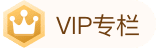
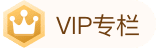
# Debugging Crashes in MATLAB: Mastering Tools and Techniques for Quick Issue Resolution
## 1. Introduction to MATLAB Crashes
A MATLAB crash refers to the sudden termination of a MATLAB program during execution without providing any error messages or prompts. This is typically caused by errors or exceptions in the code. Crashes can be extremely inconvenient for users and may result in data loss or task interruptions.
The causes of MATLAB crashes are varied and include:
- Code errors: syntax errors, logical errors, or memory access errors.
- Exceptions: unexpected events during MATLAB runtime, such as division by zero errors or file not found errors.
- Memory issues: insufficient memory or memory leaks that lead to MATLAB crashing.
## 2. Theoretical Analysis of MATLAB Crashes
### 2.1 Common Causes of Crashes
MATLAB crashes are usually caused by:
- **Insufficient memory:** A crash due to insufficient memory occurs when MATLAB tries to allocate more memory than is available. This can be caused by processing large datasets, loading multiple large variables, or performing memory-intensive calculations.
- **Stack overflow:** A stack overflow occurs when the depth of MATLAB function calls exceeds available stack space. This is often due to excessive recursion or nested loops.
- **Invalid pointer access:** A crash due to invalid pointer access occurs when MATLAB tries to access an invalid memory address. This can be caused by using uninitialized pointers, accessing beyond array bounds, or using memory that has been deallocated.
- **Failed exception handling:** When MATLAB encounters an exception (such as division by zero or a file not found), it tries to handle it through exception handling mechanisms. If this fails, it can lead to a crash.
- **External factors:** Sometimes, MATLAB crashes may be caused by external factors, such as operating system failures, hardware problems, or viral infections.
### 2.2 Principles of Debugging Crashes
Debugging crashes involves the following steps:
- **Identifying the trigger point of the crash:** Determine the specific line of code or function that causes the crash.
- **Analyzing code logic:** Examine the code to identify potential errors or flaws, such as memory leaks, stack overflows, or invalid pointer accesses.
- **Using debugging tools:** Utilize the MATLAB built-in debugger or external debugging tools to step through the code, inspect variables, and analyze the call stack to identify the root cause of the problem.
- **Fixing errors:** Once the error has been identified, modify the code to rectify it.
- **Testing and verification:** After the fix, rerun the code to verify that the issue has been resolved.
**Code Example:**
The following code example demonstrates how to use the MATLAB built-in debugger to debug a crash:
```matlab
% Set breakpoint
setdbstop('in', 'myFunction');
% Execute code
try
myFunction();
catch ME
% Handle the exception
disp(ME.message);
end
```
**Logical Analysis:**
This code sets a breakpoint in the `myFunction` function, and when execution reaches this function, the debugger will pause. It then attempts to execute `myFunction` and uses a `try-catch` block to capture any exceptions. If an exception occurs, it will display the error message.
## 3. Practical Debugging of MATLAB Crashes
### 3.1 Using the MATLAB Built-in Debugger
The MATLAB built-in debugger is a powerful tool for debugging crashes and other errors. It allows users to set breakpoints, step through code, and inspect variables.
#### 3.1.1 Setting Breakpoints and Stepping Through Code
To set a breakpoint in the code, place the cursor on the line where you want to interrupt and press F9. This will add a breakpoint to the line, and when execution reaches this line, MATLAB will pause.
To step through code, press F10. This will execute the current line and pause on the next line. Users can further control step execution using F11 (step into) and F12 (step out).
#### 3.1.
0
0
相关推荐
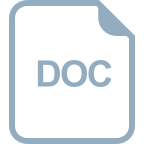
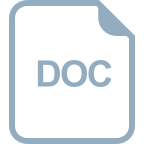
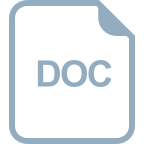
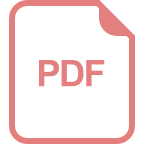
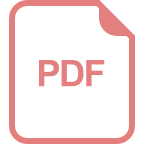
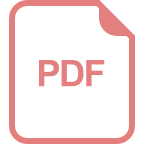
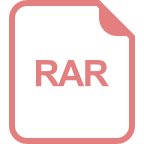
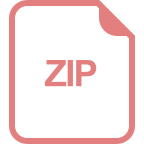
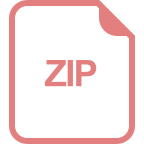