Tips and Tricks for Coding and Debugging in Visual Studio
发布时间: 2024-09-14 09:59:10 阅读量: 33 订阅数: 30 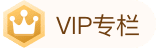
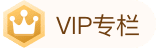
# 1. Code Editing and Debugging Tips in Visual Studio
## 1. Utilizing Shortcuts
Visual Studio is a powerful integrated development environment, and mastering some commonly used shortcuts can greatly enhance programming efficiency. Here are some of the frequently used shortcuts for code editing and debugging:
### 1.1 Common Code Editing Shortcuts
| Shortcut | Function |
|----------------|-------------------------|
| Ctrl + Space | Code Auto-Completion |
| Ctrl + D | Copy Current Line |
| Ctrl + X | Cut Current Line |
| Ctrl + C | Copy Selected Content |
| Ctrl + V | Paste |
| Ctrl + Z | Undo |
| Ctrl + Y | Redo |
### 1.2 Shortcuts Commonly Used During Debugging
| Shortcut | Function |
|----------------|-----------------------------------|
| F5 | Start Debugging |
| F9 | Set/Breakpoint |
| F10 | Step Over |
| F11 | Step Into |
| Shift + F11 | Step Out of Function Calls |
| Ctrl + Shift + B | Build Solution |
Mastering these shortcuts will make your code editing and debugging process in Visual Studio smoother and more efficient.
# 2. Code Refactoring Tools
During the software development process, code refactoring is a very important aspect that can improve the readability and maintainability of the code. Visual Studio offers many powerful code refactoring tools, and this section will introduce some commonly used tools and their specific usage methods.
### 2.1 Using the Extract Method Tool
A common task in code refactoring is to extract a piece of code into a new method to improve code reusability and readability. Visual Studio provides an "Extract Method" tool to help us accomplish this task.
Here is an example code snippet, where we will extract a piece of logic:
```csharp
public void ProcessData(int[] data)
{
int total = 0;
// Calculate sum of data
foreach (var num in data)
{
total += num;
}
// Output sum
Console.WriteLine("Total: " + total);
}
```
1. Select the code block you want to extract, such as the code inside the `foreach` loop above.
2. Right-click on the selected code block, choose "Refactor" -> "Extract Method".
3. In the pop-up window, enter a name for the new method, such as `CalculateTotal`, and click "OK".
4. Visual Studio will automatically generate the new method for us and call it within the original method, completing the code extraction.
By using the "Extract Method" tool, we can split complex code logic into multiple concise methods, improving code maintainability and readability.
### 2.2 Applying the Rename Variable Tool
Another commonly used code refactoring tool is the rename variable tool, which helps us quickly and safely change variable names in the code without affecting other parts of the code.
Consider the following code example:
```csharp
public void CalculateArea(double radius)
{
double pi = 3.14;
double area = pi * radius * radius;
Console.WriteLine("The area is: " + area);
}
```
If we want to change the variable `pi` to a more descriptive name `piValue`, we can follow these steps:
1. Place the cursor on the variable `pi`, right-click, and choose "Refactor" -> "Rename".
2. Enter the new variable name, such as `piValue`, and press Enter.
3. Visual Studio will automatically change all references to this variable, including within the current method and other methods.
Using the rename variable tool can help us avoid potential errors that may occur when manually renaming variables, improving the accuracy and efficiency of code changes.
Above are the specific applications of code refactoring tools in Visual Studio, and through reasonable refactoring operations, we can make our code clearer and easier to maintain and extend.
# 3. Debugging with Breakpoints
During the software development process, debugging is a very important环节. Visual Studio provides rich debugging features, and using breakpoints is a very common and effective debugging technique. In this section, we will learn how to use breakpoints for debugging.
## 3.1 Tips for Setting Breakpoints
Setting breakpoints in Visual Studio is very simple, just click on the left side of the code line. In addition to simply setting breakpoints on code lines, the following tips can be used to improve the efficiency of using breakpoints:
- Set breakpoints on conditional statements to pause program execution when certain conditions are met.
- Add "Conditional Breakpoint
0
0
相关推荐







