MATLAB Function Analysis: In-depth Understanding of Internal Operation Mechanisms
发布时间: 2024-09-14 12:00:18 阅读量: 25 订阅数: 37 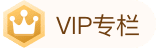
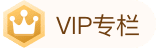
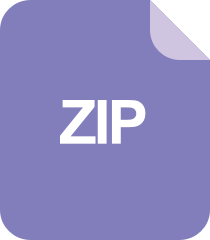
matlab代码sqrt-Design-and-Analysis-of-2-and-4-bar-mechanisms:该项目包括同时使用MAT
# 1. Fundamentals of MATLAB Functions
MATLAB functions are powerful tools for encapsulating code blocks to perform specific tasks and enhance the reusability and modularity of code. A function consists of a name, a list of parameters, and a function body, which contains the code to be executed.
The syntax for defining a function is as follows:
```
function [output_arguments] = function_name(input_arguments)
% Function body
end
```
When a function is called, MATLAB passes the actual arguments to the corresponding formal parameters in the function body and then executes the code in the function body. A function can return one or more output arguments, which will be assigned to the output variables in the calling function.
# 2. Creating and Calling MATLAB Functions
### 2.1 Syntax and Structure of Function Definitions
The definition of a MATLAB function follows a specific syntax structure:
```
function [output_args] = function_name(input_args)
% Function body
% ...
end
```
Where:
- `function`: A keyword indicating the start of the function definition.
- `[output_args]`: Optional, specifies the list of output arguments of the function, enclosed in square brackets.
- `function_name`: The name of the function, which must start with a letter and can contain letters, numbers, and underscores.
- `(input_args)`: Optional, specifies the list of input arguments of the function, enclosed in parentheses.
- `% Function body`: The actual code of the function, starting with a percent sign (%), used for commenting.
### 2.2 Methods of Calling Functions and Parameter Passing
MATLAB functions can be called in two ways:
**1. Direct Call**
```
output_value = function_name(input_value1, input_value2, ...)
```
Where:
- `output_value`: The return value of the function call.
- `function_name`: The name of the function to be called.
- `input_value1`, `input_value2`, ...: The input arguments of the function.
**2. Using the `feval` Function**
```
output_value = feval(function_name, input_value1, input_value2, ...)
```
Where:
- `output_value`: The return value of the function call.
- `feval`: A built-in MATLAB function used for dynamically calling functions.
- `function_name`: The name of the function to be called (in string form).
- `input_value1`, `input_value2`, ...: The input arguments of the function.
**Parameter Passing**
Parameter passing in MATLAB functions is done via **value passing**, meaning that modifications to the parameters within the function do not affect variables outside the function.
**Code Block:**
```
% Define a function to calculate the sum of two numbers
function sum = add_numbers(num1, num2)
% Function body
sum = num1 + num2;
end
% Call the function to calculate the sum of two numbers
result = add_numbers(10, 20);
% Print the return value of the function
disp(result);
```
**Logical Analysis:**
- The `add_numbers` function defines two input parameters `num1` and `num2`, and returns their sum.
- In the function call, `10` and `20` are passed to `num1` and `num2`.
- After the function executes, the `sum` variable is assigned the value of 30 and printed to the console.
**Parameter Description:**
| Parameter | Description |
|---|---|
| `num1` | The first number |
| `num2` | The second number |
| `sum` | The sum of the two numbers |
# 3. The Internal Mechanism of MATLAB Functions
### 3.1 Function
0
0
相关推荐







