Vectorization of MATLAB Functions: Optimizing Code for Enhanced Function Efficiency
发布时间: 2024-09-14 12:17:28 阅读量: 35 订阅数: 39 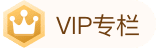
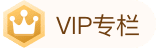
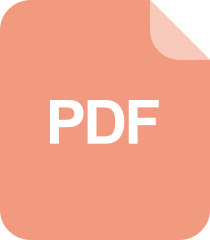
SIMD Vectorization of Histogram Functions (10.1.1.80.9041)-计算机科学
# Vectorization of MATLAB Functions: Optimizing Code and Enhancing Functionality
## 1. Basic Concepts of MATLAB Function Vectorization
Vectorization is a programming technique that leverages MATLAB's built-in functions and syntax features to perform array and matrix operations without explicit loops. Vectorized operations are more efficient in MATLAB because they utilize underlying optimization algorithms.
The core idea of vectorization technology is to use array and matrix operators to operate on all elements within an array or matrix simultaneously, rather than processing elements one by one with loops. This can significantly improve code efficiency and simplify the code structure.
## 2. Application of Vectorization Techniques in MATLAB Functions
### 2.1 Array Operations and Element Manipulations
#### 2.1.1 Vectorized Array Operations
MATLAB provides a set of vectorized array operators that can perform arithmetic operations on entire arrays without loops. These operators include:
- `+`, `-`, `*`, `/`: Addition, subtraction, multiplication, and division
- `.^`: Power operation
- `.*`, `./`: Element-wise multiplication and division
- `max`, `min`: Return the maximum and minimum values in an array
**Code Block:**
```
% Create two arrays
a = [1, 2, 3; 4, 5, 6];
b = [7, 8, 9; 10, 11, 12];
% Perform addition using vectorized array operations
c = a + b;
% Display the result
disp(c)
```
**Logical Analysis:**
This code uses the `+` operator to perform element-wise addition on two arrays `a` and `b`, and stores the result in array `c`. The `disp` function is used to display the result.
#### 2.1.2 Vectorized Element Operations
MATLAB also offers vectorized element operation functions that can perform specific operations on each element of an array. These functions include:
- `sqrt`: Calculate square root
- `exp`: Calculate exponent
- `log`: Calculate natural logarithm
- `sin`, `cos`, `tan`: Calculate trigonometric functions
**Code Block:**
```
% Create an array
a = [1, 2, 3, 4, 5];
% Calculate the square root using vectorized element operations
b = sqrt(a);
% Display the result
disp(b)
```
**Logical Analysis:**
This code uses the `sqrt` function to calculate the square root of each element in array `a`, and stores the result in array `b`. The `disp` function is used to display the result.
### 2.2 Vectorization of Loops
MATLAB provides several methods to vectorize loops, thereby enhancing code efficiency.
#### 2.2.1 Using Loop Vectorization
Loop vectorization involves using vectorized operators and functions to replace explicit loops. For example, the following code uses a loop to calculate the square of each element in an array:
```
% Create an array
a = [1, 2, 3, 4, 5];
% Use a loop to calculate squares
for i = 1:length(a)
b(i) = a(i)^2;
end
```
The same calculation can be achieved using vectorized array operators `.^`:
```
% Create an array
a = [1, 2, 3, 4, 5];
% Use vectorized array operators to calculate squares
b = a.^2;
```
**Logical Analysis:**
The second code block uses the `.^` operator to square each element in array `a`, thus avoiding explicit loops.
#### 2.2.2 Using Array Functions for Vectorization
MATLAB also offers a set of array functions that can perform specific operations on entire arrays, thereby avoiding the use of loops. These functions include:
- `sum`: Calculate the sum of array elements
- `mean`: Calculate the average of array elements
- `max`, `min`: Return the maximum and minimum values in an array
- `find`: Return the indices of array elements that satisfy a specific condition
**Code Block:**
```
% Create an array
a = [1, 2, 3, 4, 5];
% Use an array function to calculate the sum
b = sum(a);
% Display the result
disp(b)
```
**Logical Analysis:**
This code uses the `sum` function to calculate the sum of the elements in array `a`, and stores the result in variable `b`. The `disp` function is used to display the result.
# 3.1 Advantages of Vectorization
#### 3.1.1 Improved Code Efficiency
Vectorization technology significantly improves code efficiency by eliminating loops. In loops, MATLAB processes operations one element a
0
0
相关推荐







