MATLAB Function Unit Testing: A Guide to Ensuring Function Reliability and Accuracy
发布时间: 2024-09-14 12:06:19 阅读量: 25 订阅数: 33 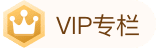
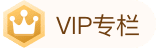
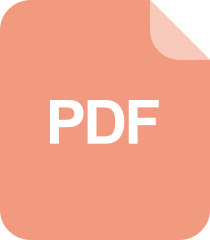
Ensuring Data Confidentiality Via plausibly deniable encryption and secure deletion - a survey
# Guide to MATLAB Function Unit Testing: Ensuring Reliability and Accuracy
## 1. Overview of MATLAB Function Unit Testing
MATLAB function unit testing is a technique used to verify the correctness and reliability of MATLAB functions. It involves creating test cases that use assertions to check if the function's output matches the expected results. Unit testing plays a crucial role in software development by improving code quality, reducing errors, and increasing confidence in code changes.
Unit testing frameworks, such as xUnit in MATLAB, provide a structure for creating and running test cases. These frameworks utilize assertions to compare actual output with expected output and generate test reports that show the results and coverage of the test cases.
With unit testing, developers can isolate and test individual functions without running the entire program. This makes debugging and fixing errors easier and allows for rapid verification of function behavior after code changes.
## 2. MATLAB Unit Testing Practices
### 2.1 Overview of Unit Testing Framework
MATLAB provides an integrated unit testing framework that enables developers to create and run unit tests. The main components of a unit testing framework include:
- **Test Function:** A function that contains the code to be tested.
- **Assertion:** A statement used to verify if the test function output meets expectations.
- **Test Case:** A set of test functions and assertions used to test a specific functionality.
- **Test Suite:** A collection of related test cases used to test a particular module or functionality.
### 2.2 Creating and Running Unit Tests
To create a unit test, follow these steps:
1. Create a new script file in MATLAB.
2. Define the test function that contains the code to be tested.
3. Use assertions within the test function to verify if the output matches the expected results.
4. Create a test case that combines the test function and assertions.
5. Add the test case to a test suite.
6. Run the test suite using the `runtests` command.
### ***
***mon assertions include:
- `assertEqual(actual, expected)`: Checks if `actual` and `expected` are equal.
- `assertLessThan(actual, expected)`: Checks if `actual` is less than `expected`.
- `assertGreaterThan(actual, expected)`: Checks if `actual` is greater than `expected`.
A test case combines a test function and assertions. A test case can be created as follows:
```matlab
function testMyFunction
% Define the test function
actual = myFunction(1, 2);
% Use an assertion to verify the output
assertEqual(actual, 3);
end
```
### 2.4 Test Coverage and Reporting
Test coverage measures how much of the code is covered by the test suite. MATLAB provides the `coverage` function to calculate test coverage.
To generate a test coverage report, follow these steps:
1. Run the test suite and use the `coverage` function to collect coverage data.
2. Use the `coverageviewer` function to generate a coverage report.
The coverage report provides information on how many code lines are covered by the test suite. It helps identify areas of the code that have not been tested and guides further test development.
**Code Block:**
```matlab
% Create a test suite
testSuite = testsuite.empty();
testSuite(1) = testsuite('MyFunctionTests');
% Add test cases to the test suite
addTest(testSuite, 'testMyFunction');
% Run the test suite
results = runtests(testSuite);
% Generate a coverage report
coverageReport = coverage(testSuite);
coverageviewer(coverageReport);
```
**Code Logic Analysis:**
This code block creates a test suite, adds a test case, runs the test suite, and generates a coverage report.
**Parameter Explanation:**
- `testsuite`: An array of structures representing the test suite.
- `testSuite(1)`: The first test suite.
- `addTest(testSuite, 'testMyFunction')`: Adds the `testMyFunction` test case to the test suite.
- `runtests(testSuite)`: Runs the test suite and returns the results.
- `coverage(testSuite)`: Calculates the coverage of the test suite and returns a cov
0
0
相关推荐







