MATLAB Reading MAT Files Unit Test: Ensuring Accuracy of Read Results, Increasing Reliability
发布时间: 2024-09-14 07:43:22 阅读量: 29 订阅数: 39 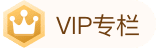
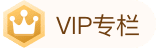
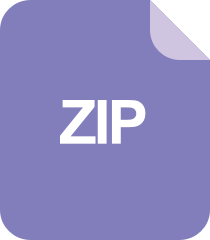
《COMSOL顺层钻孔瓦斯抽采实践案例分析与技术探讨》,COMSOL模拟技术在顺层钻孔瓦斯抽采案例中的应用研究与实践,comsol顺层钻孔瓦斯抽采案例 ,comsol;顺层钻孔;瓦斯抽采;案例,COM
# 1. Overview of MATLAB Unit Testing
MATLAB unit testing is a method to verify the correctness and reliability of MATLAB code. It involves creating test cases that examine the behavior of specific functions or modules and validate whether the output meets expectations.
The benefits of unit testing include:
- **Enhanced code quality:** By identifying errors and defects, unit testing helps ensure the robustness and reliability of the code.
- **Increased development efficiency:** Unit testing can reduce the time required for debugging and maintaining code by automating the testing process.
- **Augmented confidence:** Unit tests provide developers with confidence in the correctness of their code, allowing them to focus on higher-level tasks.
# 2. MATLAB Unit Testing Practices for Reading MAT Files
### 2.1 Testing Plan and Case Design
#### 2.1.1 Testing Objectives and Scope
A unit testing plan should clearly define the objectives and scope. For the function of reading MAT files, testing objectives might include:
- Verifying that the file is read correctly
- Ensuring that the extracted data is consistent with expected values
- Checking the performance and resource consumption of file reading
The testing scope should cover all possible file formats, data types, and reading scenarios.
#### 2.1.2 Case Design Principles and Methods
Unit test cases should follow these principles:
- **Atomicity:** Each case tests a specific function or scenario.
- **Independence:** Cases are independent of each other and can be executed separately.
- **Repeatability:***
***mon case design methods include:
- **Equivalent class partitioning:** Divide input data into equivalent classes and design cases for each class.
- **Boundary value analysis:** Test boundary values of input data, including minimum, maximum, and invalid values.
- **Error guessing:** Design cases based on guesses about possible errors.
### 2.2 Writing Unit Test Code
#### 2.2.1 Structure and Syntax of Test Functions
MATLAB unit test functions follow a specific structure and syntax:
```matlab
function test_function_name(testCase)
% Arrange: Set up the test data and environment
% Act: Perform the function under test
% Assert: Verify the results using assert statements
end
```
Where:
- `testCase` is an object provided by the unit testing framework to manage the test execution.
- The `Arrange` section sets up test data and environment.
- The `Act` section calls the function under test.
- The `Assert` section uses assert statements to verify results.
#### 2.2.2 Using and Verifying Assert Statements
MATLAB provides a series of assert statements to verify test results, including:
- `assertEqual(actual, expected)`: Verifies that the actual value equals the expected value.
- `assertNotEqual(actual, expected)`: Verifies that the actual value does not equal the expected value.
- `assertGreaterThan(actual, expected)`: Verifies that the actual value is greater than the expected value.
- `assertLessThan(actual, expected)`: Verifies that the actual value is less than the expected value.
Assert statement verification results are either true or false. If an assertion fails, the test case will fail.
### 2.3 Executing Unit Tests and Analyzing Results
#### 2.3.1 Test Execution Process and Results Display
MATLAB unit tests can be executed through the following steps:
1. Write test code and save it as a `.m` file.
2. Run the `runtests` command in the MATLAB command window.
3. MATLAB will execute all test cases and display the results.
Test results will be shown in the command window, including:
- Number of passed test cases
- Number of failed test cases
- Error messages and stack traces
#### 2.3.2 Result Analysis and Defect Localization
Analyzing test results is crucial for identifying and locating defects. If a test case fails, carefully examine the error message and stack trace to determine the root cause of the error.
Additionally, coverage tools can be used to analyze the coverage of test cases and identify uncovered code paths. This helps to improve the effectiveness and reliability of testing.
# 3. Advanced MATLAB Unit Testing for Reading MAT Files
### 3.1 Coverage Analysis and Optimization
#### 3.1.1 Coverage Concept and Calculation Method
Coverage is an important indicator for measuring the effectiveness of unit tests, indicating the proportion of executed statements or branches in the tested code. Higher coverage suggests more comprehensive test cases and higher test quality.
MATLAB provides the `codecov` tool for calculating coverage. Using `c
0
0
相关推荐





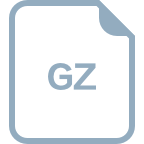
