Best Practices for MATLAB Loading of MAT Files: Enhancing Read Efficiency and Accuracy, Avoiding Pitfalls
发布时间: 2024-09-14 07:46:46 阅读量: 28 订阅数: 39 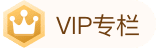
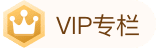
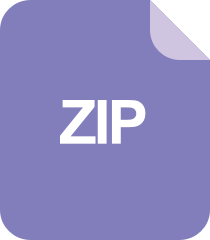
Two Scoops of Django 1.11: Best Practices for the Django Web Framework.pdf
# Best Practices for Reading MAT Files in MATLAB: Enhancing Efficiency and Accuracy, Avoiding Pitfalls
## 1. Basic Knowledge of Reading MAT Files in MATLAB
MAT files in MATLAB are a binary file format designed for storing data and variables. They are widely used in scientific computing, engineering, and data analysis. This chapter will introduce the syntax and basic operations of reading MAT files in MATLAB, laying the foundation for subsequent advanced techniques and applications.
### 1.1 Reading MAT Files
The `load()` function can be used to read MAT files. The syntax is as follows:
```matlab
load('filename.mat')
```
Here, `filename.mat` is the name of the MAT file. After executing this command, all variables in the MAT file will be loaded into the current workspace.
### 1.2 Specifying Variable Names
If you need to load only specific variables from a MAT file, you can use the `-mat` option of the `load()` function to specify variable names. The syntax is as follows:
```matlab
load('filename.mat', 'variable1', 'variable2', ...)
```
Here, `variable1`, `variable2`, etc., are the names of the variables you wish to load.
## 2. Advanced Techniques for Reading MAT Files in MATLAB
### 2.1 MAT File Structure and Data Types
#### 2.1.1 Overview of MAT File Structure
A MAT file is a binary file format for storing MATLAB variables and data. It includes a header section with information such as file version, data type, and variable names, as well as a data section where the actual data is stored.
#### 2.1.2 Common MAT File Data Types
MAT files support various data types, including:
- Numeric types: double, single, int8, int16, int32, int64, uint8, uint16, uint32, uint64
- Logical type: logical
- Character types: char, string
- Cell arrays: cell
- Structures: struct
- Objects: object
### 2.2 Optimizing MAT File Reading Performance
#### 2.2.1 Avoiding Unnecessary Loading
When reading MAT files, only load the variables you need and avoid loading unnecessary data. You can specify the variable names to load using the syntax `load(filename, 'var1', 'var2', ...)`.
#### 2.2.2 Using Preallocation and Data Type Conversion
Preallocate variables before loading MAT files to improve reading speed. Additionally, you can use the `typecast()` function to convert data to the desired data type, avoiding unnecessary type conversions.
## 3. MATLAB Reading MAT Files in Practical Applications
### 3.1 Extracting Specific Data from MAT Files
#### 3.1.1 Using the load() Function to Specify Variable Names
The load() function can be used to load specific variables from a MAT file. The syntax is as follows:
```matlab
load(filename, 'var1', 'var2', ..., 'varN')
```
Where:
* `filename`: The name of the MAT file
* `var1`, `var2`, ..., `varN`: The names of the variables to be loaded
For example, to load variables `x` and `y` from a MAT file named `data.mat`, the following code can be used:
```matlab
load('data.mat', 'x', 'y')
```
#### 3.1.2 Using the whos() Function to View MAT File Contents
The whos() function can be used to view the variables and their attributes within a MAT file. The syntax is as follows:
```matlab
whos(filename)
```
Where:
* `filename`: The name of the MAT file
Executing the whos() function will print a table containing the following information about the variables in the MAT ***
***
***
***
***
*** `data.mat` file, the following code can be used:
```matlab
whos('data.mat')
```
### 3.2 Converting MAT Files to Other Data Formats
#### 3.2.1 Converting MAT Files to CSV or Excel Files
The `writematrix()` function can be used to convert data from a MAT file into a CSV or Excel file. The syntax is as follows:
```matlab
writematrix(data, filename, 'FileType', 'csv' | 'excel')
```
Where:
* `data`: The data to be written into the file
* `filename`: The name of the output file
* `FileType`: The type of the output file, which can be `'csv'` or `'excel'`
For example, to convert the data from `data.mat` into a CSV file named `data.csv`, the following code can be used:
```matlab
data = load('data.mat');
writematrix(data.x, 'data.csv', 'FileType', 'csv')
```
#### 3.2.2 Converting CSV or Excel Files to MAT Files
The `readmatrix()` function can be used to convert data from CSV or Excel files into MAT files. The syntax is as follows:
```matlab
data =
```
0
0
相关推荐







