MATLAB Performance Optimization for Reading MAT Files: Enhancing Read Speed and Saving Time
发布时间: 2024-09-14 07:32:53 阅读量: 31 订阅数: 33 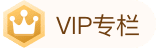
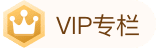
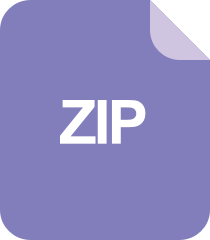
Efficient Trajectory Optimization for Robot Motion Planning:机器人运动规划的高效轨迹优化示例-matlab开发
# 1. Overview of MATLAB MAT File Reading
MATLAB MAT files are binary file formats used for storing MATLAB variables. They are an efficient and compact data storage format, widely used for data exchange and persistence in MATLAB. MAT file reading is a common operation in MATLAB, and its performance is crucial for the overall efficiency of applications. This chapter will outline the basic principles of MAT file reading and explore the key factors affecting reading performance.
# 2. Theoretical Aspects of MATLAB MAT File Reading Performance Optimization
### 2.1 MAT File Structure and Reading Principles
MATLAB MAT files are binary file formats used for storing MATLAB variables and data. MAT files consist of the following parts:
- **File Header:** Contains information such as file version, data type, and size.
- **Data Area:** Stores the actual data values.
- **Global Dictionary:** Contains mappings of variable names to data types.
When MATLAB reads a MAT file, it first reads the file header to determine the file version and data types. Then, it loads the data in the data area into memory. Finally, it uses the global dictionary to associate variable names with data values.
### 2.2 Factors Affecting Reading Performance
Factors affecting the performance of MATLAB MAT file reading include:
- **File Size:** The larger the file, the longer the reading time.
- **Data Types:** Complex data types (such as structures and cell arrays) take longer to read than simple data types (such as numbers and strings).
- **Number of Variables:** The more variables, the longer the reading time.
- **Disk Speed:** Slower disk speed will affect the file reading speed.
- **Memory Size:** Insufficient memory can cause data to be frequently swapped to disk during reading, thus reducing performance.
**Code Block 1:** Example code for reading MAT files
```matlab
% Open MAT file
matfile = matfile('data.mat');
% Read variables
data = matfile.data;
```
**Code Logic Analysis:**
- The `matfile` function opens the MAT file and returns a `matfile` object.
- The `data` attribute retrieves the variable named `data`.
**Parameter Explanation:**
- `matfile('data.mat')`: Opens the MAT file named `data.mat`.
- `data`: Returns the variable named `data`.
# 3. Practical Aspects of MATLAB MAT File Reading Performance Optimization
### 3.1 Parallel Reading Using parfor
Parallel computing can significantly improve MAT file reading performance, especially for large files. MATLAB provides the `parfor` statement, which can parallelize loops and read different parts of the file simultaneously.
**Example Code:**
```matlab
% Create a MAT file with 1000 variables
save('test.mat', 'var1', 'var2', ..., 'var1000');
% Use parfor to parallel read the file
parfor i = 1:1000
data{i} = load('test.mat', ['var' num2str(i)]);
end
```
**Logic Analysis:**
The `parfor` statement parallelizes the loop, reading different variables from the file simultaneously. `num2str(i)` converts the numeric index to a string to dynamically build variable names.
**Parameter Explanation:**
* `i`: Loop index, indicating the variable index to be read.
### 3.2 Optimizing Data Structures and Variable Types
The structure of data and the types of variables in a MAT file also affect reading performance. Optimizing data structures and variable types can reduce memory consumption and reading time.
**Optimizing Data Structures:**
* Use structures or classes to organize data, instead of using nested arrays.
* Avoid using sparse matrices, as they require additional processing during reading.
**Optimizing Variable Types:**
* Use numeric types (such as `double`, `single`) to store data, instead of strings or cell arrays.
* Consider using compressed data types (such as `uint8`, `int16`) to reduce memory consumption.
0
0
相关推荐
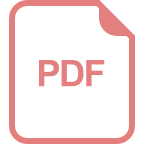
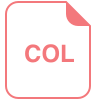
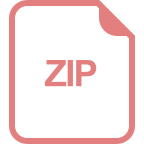
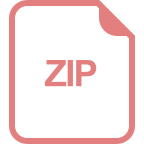
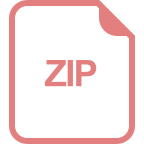
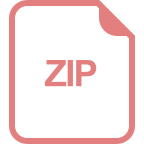
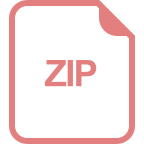