MATLAB Processing of Multiple MAT Files: Reading Multiple MAT Files at Once for Increased Efficiency
发布时间: 2024-09-14 07:34:50 阅读量: 26 订阅数: 28 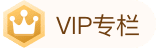
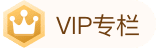
# Introduction to MATLAB MAT Files
MATLAB MAT files are a binary file format used for storing variables from the MATLAB workspace. They offer a convenient way to save and load data, variables, and objects, enabling data sharing and persistence. MAT files are typically used to store large datasets, intermediate calculation results, and custom objects.
# Tips for Reading MAT Files in MATLAB
## 2.1 Loading a Single MAT File
### 2.1.1 Using the load Function
The load function is the simplest method for loading MAT files. It accepts a path to a MAT file as input and loads all variables from the file into the current workspace.
```
% Load MAT file
load('my_data.mat');
```
**Argument Explanation:**
* `'my_data.mat'`: The path to the MAT file to be loaded.
**Code Logic Analysis:**
* The load function opens the specified MAT file and reads its data.
* It loads all variables from the MAT file into the current workspace.
* The names of the variables are the same as those stored in the MAT file.
### 2.1.2 Applying the who Function
The who function can display all variables loaded into the current workspace. It helps verify if the MAT file has been successfully loaded.
```
% View loaded variables
who
```
**Argument Explanation:**
* None.
**Code Logic Analysis:**
* The who function prints the names and types of all variables in the current workspace.
* If the MAT file has been successfully loaded, you should see the names of the variables from the MAT file in the output.
## 2.2 Loading Multiple MAT Files
### 2.2.1 Applying the dir Function
The dir function retrieves information about all files and folders in the specified directory. It can be used to find and load multiple MAT files.
```
% Get all MAT files in the current directory
files = dir('*.mat');
```
**Argument Explanation:**
* `'*.mat'`: The file extension to be searched for.
**Code Logic Analysis:**
* The dir function returns a structure array containing detailed information about each file and folder.
* The files structure array contains a field named name, which stores the names of the files and folders.
### 2.2.2 Using the cell2mat Function
The cell2mat function can convert a cell array into a matrix. It can be used to convert the structure array returned by the dir function into a matrix of MAT file names.
```
% Convert structure array into a matrix of MAT file names
file_names = cell2mat({files.name});
```
**Argument Explanation:**
* `{files.name}`: The field name of the structure array to be converted.
**Code Logic Analysis:**
* The cell2mat function converts the name field of the files structure array into a cell array.
* It then converts the cell array into a matrix where each row contains the name of a MAT file.
## 2.3 Extracting Variables from a MAT File
### 2.3.1 Applying the whos Function
The whos function can display detailed information about the variables stored in a MAT file, including the variable names, types, sizes, and complexities.
```
% Display variable information in a MAT file
whos('-file', 'my_data.mat')
```
**Argument Explanation:**
* `'-file'`: The path to the MAT file to be checked.
**Code Logic Analysis:**
* The whos function reads the specified MAT file and displays detailed information about the variables stored in it.
* The output includes the names, types, sizes, and complexities of the variables.
### 2.3.2 Using the evalin Function
The evalin function allows you to execute MATLAB expressions contained in a string. It can be used to extract specific variables from a MAT file.
```
% Extract variables from a MAT file
variable_value = evalin('base', 'my_variable');
```
**Argument Explanation:**
* `'base'`: The scope in which to execute the expression.
* `'my_variable'`: The name of the variable to be extracted.
**Code Logic Analysis:**
* The evalin function executes the MATLAB expression contained in the string `'my_variable'`.
* After evaluation, the result is stored in the variable_value variable.
# 3.1 Merging Multiple MAT Files
### 3.1.1 Creating a New MAT File
To merge multiple MAT files, you first need to create a new MAT file to store the combined data. You can create a new MAT file using the `save` function as follows:
```
% Create a new MAT file
save('merged_data.mat');
```
### 3.1.2 Saving Variables to the New MAT File
Next, you need to save the variables from each MAT file into the newly created MAT file. You can use the `save` function to save variables into a MAT file, as shown in the syntax below:
```
save(filename, variables)
```
Where `filename` is the name of the MAT file, and `variables` is the name of the variables to be saved.
F
0
0
相关推荐
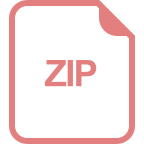
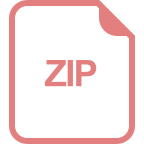
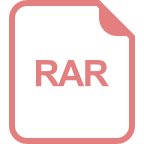
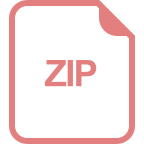
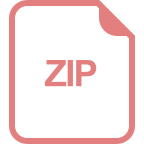
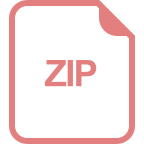
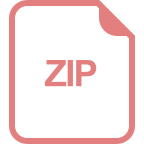
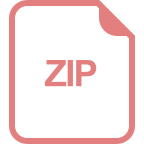
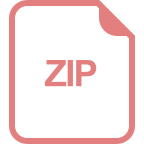