Parsing Structures in MATLAB for .MAT File Reading: A Deep Understanding of Data Structures and Mastering Data Organization
发布时间: 2024-09-14 07:26:14 阅读量: 49 订阅数: 39 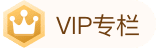
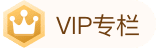
# 1. Understanding MATLAB MAT Files: A Deep Dive into Data Structures
**Overview of MAT File Format**
The MATLAB MAT file format is a binary file format designed for storing MATLAB variables and data. It consists of a header section and a data section. The header contains information about the file format, data types, and variable names. The data section holds the actual data.
**Organizing Data Structures**
Data in a MAT file is organized hierarchically. Data can be stored in variables, arrays, structures, and cell arrays. Variables are single values, while arrays are collections of multiple values of the same data type. Structures are collections of variables with named fields. Cell arrays are arrays that can contain elements of different data types.
# 2. Theoretical Foundations of Structure Parsing
### Concepts and Components of Structures
A structure is a data type used to organize related data of different data types. It consists of a set of key-value pairs known as fields, each with a unique name and data type. Data within a structure can be scalars, vectors, matrices, other structures, or even function handles.
### Representing Structures in MATLAB
In MATLAB, structures are created using the `struct` function. The `struct` function takes a list of field names and their corresponding data values. For example, creating a structure with name, age, and occupation:
```matlab
person = struct('name', 'John Doe', 'age', 30, 'occupation', 'Software Engineer');
```
### Basic Functions for Manipulating Structures
MATLAB provides basic functions for accessing and modifying structure fields:
- **fieldnames()**: Returns the names of all fields in a structure.
- **getfield()**: Retrieves the value of a field in a structure based on its name.
- **setfield()**: Sets the value of a field in a structure based on its name.
- **rmfield()**: Removes a field from a structure.
```matlab
% Getting the value of the "name" field
name = getfield(person, 'name');
% Setting the value of the "age" field
setfield(person, 'age', 31);
% Deleting the "occupation" field
rmfield(person, 'occupation');
```
# 3. Practical Methods for Structure Parsing
### Reading Structural Data from MAT Files
To read structural data from a MAT file, use the `load` function. This function takes the path of the MAT file as a parameter and returns a structure variable containing the file's content. For example:
```matlab
% Reading a MAT file named "data.mat"
data = load('data.mat');
```
### Accessing and Modifying Structure Fields
To access structure fields, use the dot operator (.`). For example:
```matlab
% Accessing the "name" field of a structure named "data"
name = data.name;
```
To modify structure fields, use the assignment operator (`` =). For example:
```matlab
% Changing the value of the "age" field in the "data" structure
data.age = 30;
```
### Parsing and Handling Nested Structures
MATLAB supports nested structures, ***arse nested structures, use the dot operator and subscripting. For example:
```matlab
% Accessing the "city" field within the "address" field of a structure named "data"
city = data.address.city;
```
```matlab
% Modifying the "street" field within the "address" field of a structure named "data"
data.address.street = 'New Street';
```
### Looping Through Nested Structures
To iterate through nested structures, you can use recursive functions or loops. Recursive functions call themselves to navigate through the nested levels of a structure. Looping iterates through the fields and subfields of a structure using loop statements.
**Recursive Function Example:**
```matlab
function printNestedStruct(struct)
% Iterating through the structure's fields
for field = fieldnames(struct)'
% If the field is a structure, recursively call the function
if isstruct(struct.(field{1}))
printNestedStruct(struct.(field{1}));
else
% If the field is not a structure, print the field name and value
fprintf('%s: %s\n', field{1}, struct.(field{1}));
end
end
end
```
**Looping Example:**
```matlab
% Iterating through the fields of a structure named "data"
for field = fieldnames(data)'
% If the field is a structure, iterate through its subfields
if isstruct(data.(field{1}))
for subfield = fieldnames(data.(field{1}))'
fprintf('%s.%s: %s\n', field{1}, subfield{1}, data.(field{1}).(subfield{1}));
end
else
% If the field is not a structure, print the field name and value
fprintf('%s: %s\n', field{1}, data.(field{1}));
end
end
```
# 4. Advanced Applications of Structure Parsing
### 4.1 Using Loops and Conditional Statements to Handle Complex Structures
In real-world applications, structure data often has complex structures, possibly including nested structures, arrays, and custom data types. To handle such complexity, loops and conditional statements can be utilized.
**Example: Iterating Through Nested Structures**
```matlab
% Loading nested structure data
data = load('nested_struct.mat');
% Iterating through nested structures
for i = 1:length(data.struct1)
for j = 1:length(data.struct1(i).struct2)
disp(['Element (', num2str(i), ', ', num2str(j), '): ', data.struct1(i).struct2(j).value]);
end
end
```
**Logical Analysis:**
* The outer loop iterates through the elements of the outer structure `struct1`.
* The inner loop iterates through the elements of the inner structure `struct2`.
* For each element, the `disp` function is used to display its value.
**Example: Filtering Structure Fields Based on Conditions**
```matlab
% Loading structure data
data = load('employee_data.mat');
% Filtering structure fields based on conditions
filtered_data = data.employees(data.employees.salary > 50000);
```
**Logical Analysis:**
* The `load` function is used to read the structure data.
* The condition `data.employees.salary > 50000` is used to filter structure fields that meet the criteria.
* The filtered data is stored in `filtered_data`.
### 4.2 Combining Other Data Types and Functions for In-Depth Data Analysis
Structure parsing can be combined with other data types and functions for more in-depth data analysis.
**Example: Using Array Operations to Process Structure Data**
```matlab
% Loading structure data
data = load('student_data.mat');
% Calculating the average grade for each student
mean_grades = mean([data.students.grades], 2);
```
**Logical Analysis:**
* The `mean` function is used to calculate each student's average grade.
* `[data.students.grades]` accesses the array of all students' grades.
* `mean([], 2)` computes the column-wise mean of each array.
**Example: Using Custom Functions to Process Structure Data**
```matlab
% Defining a custom function
function [max_value, max_index] = find_max_value(struct_array)
max_value = -Inf;
max_index = 0;
for i = 1:length(struct_array)
if struct_array(i).value > max_value
max_value = struct_array(i).value;
max_index = i;
end
end
end
% Loading structure data
data = load('sales_data.mat');
% Using a custom function to find the maximum sales
[max_sales, max_sales_index] = find_max_value(data.sales);
```
**Logical Analysis:**
* A custom function `find_max_value` is defined to find the maximum value and its index in a structure array.
* The `load` function is used to read the structure data.
* The custom function is called to find the maximum sales and its index.
### 4.3 Visualizing and Exporting Structure Data
Visualizing and exporting structure data are crucial for data analysis and reporting.
**Example: Using the `plot` Function to Visualize Structure Data**
```matlab
% Loading structure data
data = load('stock_data.mat');
% Visualizing stock prices
plot(data.stock_prices.dates, data.stock_prices.values);
```
**Logical Analysis:**
* The `plot` function is used to plot the stock prices over time.
* `data.stock_prices.dates` accesses the dates of the stock prices.
* `data.stock_prices.values` accesses the values of the stock prices.
**Example: Using the `writetable` Function to Export Structure Data**
```matlab
% Loading structure data
data = load('customer_data.mat');
% Exporting structure data to a CSV file
writetable(struct2table(data.customers), 'customer_data.csv');
```
**Logical Analysis:**
* The `struct2table` function is used to convert structures to tables.
* The `writetable` function is used to export the table to a CSV file.
# 5. Best Practices for Structure Parsing in MATLAB
### Enhancing Code Readability and Maintainability
- **Use meaningful variable names:** Choose clear and descriptive names for structure fields and variables to improve code readability.
- **Adopt consistent naming conventions:** Use consistent naming conventions throughout the code, such as capitalization or underscores, to enhance maintainability.
- **Add comments:** Include comments to explain the purpose of the code, algorithms, and any potential limitations, which helps other developers understand and maintain the code.
- **Use structure validation:** Use the `isstruct` function to verify that a variable is a structure to avoid errors and unintended behavior.
### Optimizing Structure Parsing Performance
- **Avoid unnecessary copying:** Use `structfun` or loops to manipulate structure fields instead of creating copies of structures.
- **Use preallocation:** When parsing structures in a loop, preallocate the output variable's size to improve performance.
- **Leverage parallel processing:** For large structures, parallel processing can speed up the parsing process.
### Avoiding Common Errors and Pitfalls
- **Avoid using the dot operator:** Accessing structure fields with the dot operator (``.) can lead to errors as it is prone to string interpolation.
- **Be cautious with nested structures:** When handling nested structures, ensure you use the correct syntax for accessing subfields.
- **Avoid modifying the original structure:** When parsing structures, it's best to create a copy to modify to prevent accidentally altering the original data.
- **Use error handling:** Utilize `try-catch` blocks to handle errors during structure parsing to ensure the robustness of the code.
0
0
相关推荐








