uint8 Verification in an Automated Testing Framework: Enhancing Code Quality and Ensuring Software Reliability
发布时间: 2024-09-14 13:13:02 阅读量: 15 订阅数: 18 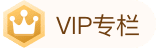
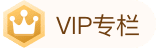
# Validation of uint8 in Automation Testing Framework: Enhancing Code Quality and Ensuring Software Reliability
The validation of uint8 is crucial in automation testing frameworks as it ensures the correctness of the uint8 data type, thereby improving the reliability and stability of software. The uint8 data type is an 8-bit unsigned integer widely used in embedded systems and communication protocols. Due to its limited range, uint8 variables are prone to overflow or underflow errors, which can cause abnormal program behavior. Therefore, validating uint8 variables in automation testing is essential.
# Theoretical Foundations of uint8 Validation
### 2.1 uint8 Data Type and Its Characteristics
The uint8 data type is an unsigned 8-bit integer data type that can represent integers ranging from 0 to 255. The uint8 type is widely used in embedded systems and microcontrollers due to its small memory footprint and fast processing speed.
The characteristics of the uint8 data type include:
- **Range:** 0 to 255
- **Memory Footprint:** 1 byte
- **Unsigned:** Only positive integers can be represented
- **Overflow:** When the value of a uint8 variable exceeds 255, it will start counting from 0 again
### 2.2 Necessity and Importance of uint8 Validation
uint8 validation is a key step in ensuring the correctness and integrity of the uint8 data type. Here are some reasons for the necessity and importance of uint8 validation:
- **Ensuring Data Integrity:** uint8 validation can help ensure that uint8 data is not corrupted or tampered with during storage, transmission, or processing.
- **Preventing Buffer Overflow:** uint8 validation can prevent buffer overflows, a common security vulnerability caused by uint8 variables exceeding their allocated memory space.
- **Increasing Reliability:** By validating uint8 data, the overall reliability of embedded systems and microcontrollers can be improved, thereby reducing faults and errors.
- **Compliance with Industry Standards:** Many industry standards, such as MISRA-C and IEC 61508, require the validation of uint8 data.
Therefore, uint8 validation is crucial in the development of embedded systems and microcontrollers, as it ensures data integrity, prevents buffer overflow, improves reliability, and complies with industry standards.
# Validation of uint8 in Unit Testing
Unit testing targets individual functions or modules to verify the correctness of their internal logic. Conducting uint8 validation in unit testing is essential as it can help ensure that functions or modules do not produce errors when processing uint8 data types.
### 3.1.1 Using Assertion Libraries for Validation
An assertion library provides macros or functions for checking if test conditions are true. In unit testing, assertion libraries can be used to verify if uint8 variables match expected values. For example, in C, the `assert` macro can be used to verify that a uint8 variable equals a certain value:
```c
#include <assert.h>
void test_uint8_addition() {
uint8_t a = 10;
uint8_t b = 5;
uint8_t expected_result = 15;
uint8_t result = a + b;
assert(result == expected_result);
}
```
In the above code, the `assert` macro checks if the `result` variable equals the `expected_result` variable. If the condition is false, the test fails and an error message is printed.
### 3.1.2 Custom uint8 Validation Functions
In addition to using assertion libraries, custom uint8 validation functions can be created. This is useful for validating more complex conditions or performing additional operations, such as logging failure information. For example, a custom uint8 validation function can be created in C++ as follows:
```cpp
#include <iostream>
bool validate_uint8(uint8_t value, uint8_t expected_value) {
if (
```
0
0
相关推荐
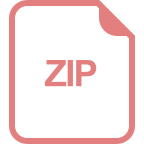
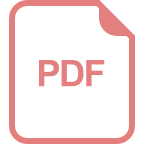
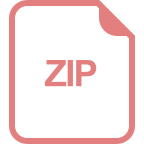
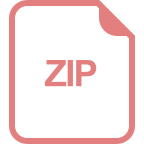
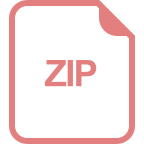
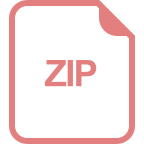
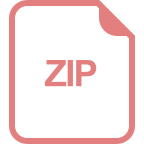
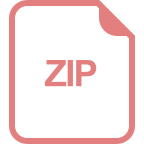