Best Practices for uint8 Value Range Limitation: Ensuring Data Integrity and Avoiding Data Errors
发布时间: 2024-09-14 13:11:03 阅读量: 16 订阅数: 20 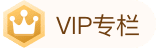
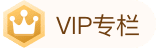
# Understanding the Basics of uint8 Data Type
The uint8 data type is an unsigned integer type that represents an 8-bit unsigned integer in computer systems. It can represent integer values between 0 and 255, where 0 is the minimum value and 255 is the maximum. The uint8 data type is commonly used to store small integers, such as boolean values, enumeration values, or counters.
The advantages of the uint8 data type include its small memory footprint and fast processing speed. However, its drawbacks are its limited value range and its inability to represent negative numbers or integers greater than 255. Therefore, when using the uint8 data type, it is essential to consider its range limitations to avoid data overflow or other errors.
# Theoretical Foundation of uint8 Range Limitations
### 2.1 Characteristics and Limitations of uint8 Data Type
The uint8 data type is an unsigned integer type that occupies 8 bits of memory space. Its value range is from 0 to 255 in decimal, or 0x00 to 0xFF in hexadecimal. The uint8 data type is widely used in embedded systems, network protocols, and data structures.
The characteristics and limitations of the uint8 data type are as follows:
- **Unsigned:** The uint8 data type can only represent positive integers and cannot represent negative numbers.
- **8 bits:** The uint8 data type occupies 8 bits of memory space, thus its value range is limited.
- **Overflow:** When the value of a uint8 variable exceeds its value range, overflow occurs. Overflow can lead to data loss or incorrect behavior.
### 2.2 Necessity of Value Range Limitations
Limiting the value range of the uint8 data type is crucial for the following reasons:
- **Data Integrity:** Limiting the value range ensures data integrity and prevents values outside the range from corrupting the system or causing errors.
- **Performance Optimization:** By limiting the value range, compilers can optimize code because they know the possible values of the variables.
- **Maintainability:** Limiting the value range can improve code maintainability, as developers can clearly understand the expected values of variables.
- **Security Enhancement:** Limiting the value range can enhance security because attackers cannot exploit values outside the range to compromise the system.
# Practical Methods for uint8 Range Limitations
### 3.1 Compiler-Level Limitations
#### 3.1.1 Using Enumeration Types
Enumeration types are a mechanism for defining a set of related values as constants. For the uint8 data type, enumeration types can be used to define the allowed value range. For example:
```c++
enum class MyUint8 {
Value1 = 1,
Value2 = 2,
Value3 = 3
};
```
This enumeration type defines three allowed uint8
0
0
相关推荐
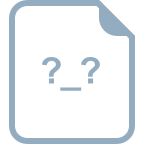
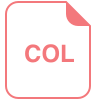
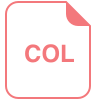
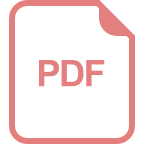
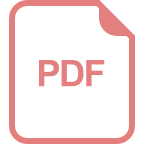
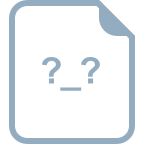
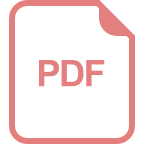
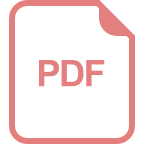