Unveiling the Secrets of MATLAB Custom Functions: From Novice to Expert
发布时间: 2024-09-14 11:54:03 阅读量: 29 订阅数: 37 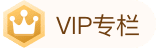
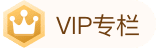
# Unveiling MATLAB Custom Function Secrets: From Novice to Expert
## 1. Overview of MATLAB Custom Functions
MATLAB custom functions are user-created functions designed to perform specific tasks or calculations. They enable users to encapsulate their code for reusability and ease of maintenance. Custom functions can accept input parameters, carry out computations, and return output results. They are vital tools for extending MATLAB's capabilities and simplifying complex tasks.
Custom functions in MATLAB are created using the `function` keyword. The function definition consists of a function name, optional input parameters, and optional output parameters. The function body contains the code to be executed. When calling a custom function, use the function name and pass input parameters if necessary. After execution, the function will return output parameters if required.
## 2. Creating and Syntax of MATLAB Custom Functions
### 2.1 Function Definition and Invocation
In MATLAB, custom functions are defined using the `function` keyword. The syntax for a function definition is as follows:
```matlab
function [output_arguments] = function_name(input_arguments)
% Function body
end
```
***function_name:** The name of the function, following MATLAB naming conventions.
***input_arguments:** A list of input parameters, which may include multiple parameters separated by commas.
***output_arguments:** A list of output parameters, enclosed in square brackets.
***Function body:** The block of code that the function executes.
**Function Invocation:**
```matlab
output_variables = function_name(input_variables);
```
***output_variables:** Variables that store the function's output results.
***input_variables:** Input parameters passed when invoking the function.
### 2.2 Input and Output Parameters and Variable Scope
**Input and Output Parameters:**
* Input parameters: Parameters specified in the function definition used to receive external data.
* Output parameters: Results returned after the function executes, enclosed in square brackets.
**Variable Scope:**
***Local variables:** Variables defined within a function, valid only inside the function.
***Global variables:** Variables defined outside a function, accessible within the function.
### 2.3 Function Handles and Anonymous Functions
**Function Handles:**
A function handle is a special data type in MATLAB that references a function. You can obtain a function handle using the `@` symbol:
```matlab
function_handle = @function_name;
```
Function handles can be passed and used like regular variables:
```matlab
new_function = function_handle(input_variables);
```
**Anonymous Functions:**
An anonymous function is a nameless function in MATLAB, defined using the syntax `@(input_arguments) expression`:
```matlab
anonymous_function = @(x) x^2;
```
Anonymous functions can be used like regular functions:
```matlab
result = anonymous_function(input_value);
```
# 3. Advanced Techniques for MATLAB Custom Functions
### 3.1 Conditional Statements and Loop Control
In custom functions, conditional statements and loop control are essential tools for controlling program flow and performing specific tasks.
**Conditional statements***mon conditional statements in MATLAB include:
- **if-else** statements: Execute different code blocks when conditions are true or false.
- **switch-case** statements: Execute different code blocks based on the value of a variable.
**Loop control***mon loop controls in MATLAB include:
- **for** loops: Execute code blocks for a series of values.
- **while** loops: Execute code blocks while a condition is true.
- **break** and **continue** statements: Used to control the loop execution flow.
### 3.2 Error Handling and Exception Capturing
During function execution, errors or exceptional conditions may occur. To handle these cases, MATLAB provides error handling and exception capturing mechanisms.
**Error Handling** uses **try-catch** statements to catch and process errors. The **try** block contains code that may raise errors, while the **catch** block contains code that processes the errors.
**Exception Capturing** uses **throw** and **catch** statements to catch and process exceptions. The **throw** statement is used to raise exceptions, while the **catch** block is used to handle specific types of exceptions.
### 3.3 Function Overloading and Variable Arguments
**Function Overloading** allows defining multiple functions with the same name but different parameter lists. When an overloaded function is called, MATLAB selects the function to execute based on the parameter list.
**Variable Arguments** allow functions to accept a variable number of input arguments. In MATLAB, **varargin** and **varargout** variables represent variable arguments. **varargin** is used to represent variable input arguments, while **varargout** is used to represent variable output arguments.
#### Code Examples
The following code examples demonstrate the use of conditional statements, loop control, error handling, and function overloading:
```
% Conditional Statements
if x > 0
disp('x is positive')
else
disp('x is non-positive')
end
% Loop Control
for i = 1:10
disp(['Iteration ', num2str(i)])
end
% Error Handling
try
a = 1 / 0;
catch ME
disp(['Error: ', ME.message])
end
% Function Overloading
function sum(x, y)
disp(['Sum of x and y: ', num2str(x + y)])
end
function sum(x, y, z)
disp(['Sum of x, y, and z: ', num2str(x + y + z)])
end
sum(1, 2)
sum(1, 2, 3)
```
**Code Logic Analysis:**
***Conditional Statements:** Check if `x` is greater than 0 and output different messages based on the condition.
***Loop Control:** Use a `for` loop to execute ten iterations and output the iteration number each time.
***Error Handling:** Use `try-catch` statements to catch division by zero errors and output an error message.
***Function Overloading:** Define two `sum` functions with the same name but different parameter lists. MATLAB selects the function to execute based on the parameter list.
# 4. Practical Applications of MATLAB Custom Functions
### 4.1 Numerical Computation and Data Processing
MATLAB custom functions have broad applications in numerical computation and data processing. For instance, we can write functions to perform operations such as:
- **Numerical Operations:** Solving equations, matrix operations, calculating statistics, etc.
- **Data Processing:** Data cleaning, data transformation, data analysis, etc.
**Code Block 1: Solving a Quadratic Equation**
```matlab
function [x1, x2] = quadratic_solver(a, b, c)
% Solving a quadratic equation ax^2 + bx + c = 0
% Input: a, b, c are the coefficients of the equation
% Output: x1, x2 are the two solutions of the equation
% Calculate the discriminant
D = b^2 - 4*a*c;
% Determine the type of equation based on the discriminant
if D > 0
% Real number solutions
x1 = (-b + sqrt(D)) / (2*a);
x2 = (-b - sqrt(D)) / (2*a);
elseif D == 0
% Repeated roots
x1 = x2 = -b / (2*a);
else
% No real number solutions
x1 = NaN;
x2 = NaN;
end
end
```
**Logic Analysis:**
* The `quadratic_solver` function accepts three parameters: `a`, `b`, and `c`, representing the coefficients of a quadratic equation.
* It first calculates the discriminant `D` to determine the type of the equation.
* Depending on the discriminant, the function returns two solutions `x1` and `x2`, or `NaN` if there are no real number solutions.
### 4.2 Graph Drawing and Visualization
MATLAB custom functions can also be used to create various types of graphs, including:
- **Line Graphs:** Connecting lines between data points.
- **Scatter Plots:** A collection of data points.
- **Bar Graphs:** Bars representing data values.
- **Pie Charts:** A pie chart showing the proportion of data values.
**Code Block 2: Drawing a Line Graph**
```matlab
function plot_line(x, y)
% Drawing a line graph
% Input: x, y are the data points
% Output: None
plot(x, y, 'b-o');
xlabel('x');
ylabel('y');
title('Line Graph');
grid on;
end
```
**Logic Analysis:**
* The `plot_line` function accepts two parameters: `x` and `y`, representing the x and y coordinates of the data points.
* It uses the `plot` function to draw connecting lines between data points and sets the line style, color, marker, and labels.
* The function also adds grid lines and a title to enhance the graph's readability.
### 4.3 File Reading and Writing for Data Persistence
MATLAB custom functions can be used to read and write files, achieving data persistence. For example, we can write functions to perform operations such as:
- **File Reading:** Reading data from text files, CSV files, or other data sources.
- **File Writing:** Writing data to text files, CSV files, or other data sources.
**Code Block 3: Reading a CSV File**
```matlab
function data = read_csv(filename)
% Reading a CSV file
% Input: filename is the name of the CSV file
% Output: data is the data read
% Open the CSV file
fid = fopen(filename, 'r');
% Read the file header
header = fgetl(fid);
% Read the data
data = textscan(fid, '%f,%f,%f');
% Close the CSV file
fclose(fid);
end
```
**Logic Analysis:**
* The `read_csv` function accepts one parameter: `filename`, indicating the name of the CSV file.
* It first opens the CSV file and reads the header.
* Then, it uses the `textscan` function to read the data and stores it in the `data` variable.
* Finally, it closes the CSV file.
# 5. Performance Optimization of MATLAB Custom Functions
### 5.1 Algorithm Selection and Code Optimization
**Algorithm Selection**
* Choose efficient algorithms, such as quicksort, binary search, etc.
* Consider the time and space complexity of algorithms to avoid using those with excessively high complexity.
**Code Optimization**
***Avoid unnecessary loops and branches:** Use vectorized operations and conditional operators to simplify code.
***Reduce function calls:** Inline frequently called functions into the main code.
***Use preallocation:** Allocate memory for arrays and matrices in advance to avoid multiple allocations and deallocations.
***Leverage MATLAB built-in functions:** MATLAB offers many efficient built-in functions, such as `sum()` and `mean()`, which can replace manual loops.
### 5.2 Memory Management and Parallel Computing
**Memory Management**
***Avoid memory leaks:** Ensure all variables in functions are released using `clear` or `delete` commands.
***Optimize memory allocation:** Use the `prealloc` function to preallocate memory and avoid frequent memory allocation and deallocation.
***Use memory-mapped files:** For large datasets, memory-mapped files can improve memory access speed.
**Parallel Computing**
***Leverage parallel toolboxes:** MATLAB provides parallel toolboxes that support parallel computing.
***Use `parfor` loops:** Parallelize loops to increase computing speed.
***Pay attention to data partitioning:** Reasonably partition data to fully utilize parallel computing.
### 5.3 Code Testing and Debugging
**Code Testing**
***Write unit tests:** Use the `unittest` framework to write unit tests to verify the correctness of functions.
***Use assertions:** Insert `assert` statements in the code to check if the function's output matches expectations.
***Boundary condition testing:** Test the function's behavior under boundary conditions, such as invalid or empty inputs.
**Code Debugging**
***Use a debugger:** MATLAB provides a debugger that allows you to step through the code line by line and inspect variable values.
***Use `disp()` and `fprintf()`:** Insert `disp()` and `fprintf()` in the code to print variable values to help locate issues.
***Use a profiler:** Use MATLAB's profiler to analyze code performance and identify bottlenecks.
0
0
相关推荐









