Mastering MATLAB Custom Functions: Advanced Usage and Best Practices Guide
发布时间: 2024-09-14 11:57:09 阅读量: 35 订阅数: 30 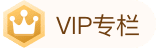
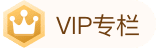
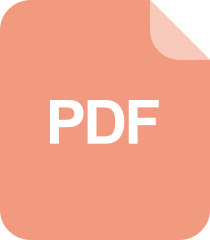
Vue Step-By-Step Guide To Mastering Vue.js From Beginner To Advanced 无水印pdf

# Mastering MATLAB Custom Functions: Advanced Usage and Best Practices Guide
MATLAB custom functions are user-defined functions that perform specific tasks or computations. They offer the advantages of modularity, reusability, and code organization.
### 1.1 Function Definition
MATLAB functions are defined using the `function` keyword, followed by the function name and a list of input parameters. The function body contains the code to be executed and ends with the `end` keyword.
```matlab
function myFunction(x, y)
% Function body
z = x + y;
disp(z);
end
```
# 2. Advanced Usage of MATLAB Custom Functions
### 2.1 Function Inputs and Outputs
#### 2.1.1 Definition and Passing of Input Parameters
MATLAB functions can receive input parameters, which are specified at the time of function definition. The format for defining input parameters is as follows:
```
function output_args = function_name(input_arg1, input_arg2, ...)
```
Where:
* `output_args`: The function's output arguments, which can be multiple.
* `function_name`: The name of the function.
* `input_arg1`, `input_arg2`, ...: The function's input arguments, which can be multiple.
Input parameters can be passed to a function by including the parameter values as arguments in the function call. For example:
```
result = my_function(x, y, z);
```
#### 2.1.2 Definition and Return of Output Parameters
MATLAB functions can return output parameters, which are specified at the time of function definition. The format for defining output parameters is as follows:
```
function [output_arg1, output_arg2, ...] = function_name(input_arg1, input_arg2, ...)
```
Where:
* `output_arg1`, `output_arg2`, ...: The function's output arguments, which can be multiple.
* `function_name`: The name of the function.
* `input_arg1`, `input_arg2`, ...: The function's input arguments, which can be multiple.
Output parameters can be returned through the assignment statement of the function call. For example:
```
[a, b, c] = my_function(x, y, z);
```
### 2.2 Function Handles and Anonymous Functions
#### 2.2.1 Concept and Usage of Function Handles
A function handle is a reference to a function. It allows functions to be passed as arguments to other functions or stored in data structures. The format for creating a function handle is as follows:
```
function_handle = @function_name;
```
Where:
* `function_handle`: A handle that points to the function.
* `function_name`: The name of the function.
Function handles can be called like normal functions, using parentheses and parameters. For example:
```
result = function_handle(x, y, z);
```
#### 2.2.2 Definition and Application of Anonymous Functions
Anonymous functions are functions without a name, defined directly at the point of function call. The format for defining an anonymous function is as follows:
```
@(input_arg1, input_arg2, ...) output_expression
```
Where:
* `input_arg1`, `input_arg2`, ...: The anonymous function's input arguments, which can be multiple.
* `output_expression`: The anonymous function's output expression.
Anonymous functions can be called like normal functions, using parentheses and parameters. For example:
```
result = @(x, y, z) x + y + z;
```
### 2.3 Function Nesting and Recursion
#### 2.3.1 Principle and Application of Function Nesting
Function nesting refers to defining one function within another. Nested functions can access variables and parameters of the outer function. The benefits of function nesting include:
* Code organization and modularity.
* Reduction of code duplication.
* Increased code readability.
#### 2.3.2 Definition and Implementation of Recursive Functions
Recursive functions are those that call themselves. Recursive functions are used to solve problems with self-similar structures. The format for defining a recursive function is as follows:
```
function output_args = function_name(input_args)
% Recursive base case
if (base_condition)
return output_args;
end
% Recursive call
output_args = function_name(new_input_args);
end
```
Where:
* `output_args`: The function's output arguments, which can be multiple.
* `function_name`: The name of the function.
* `input_args`: The function's input arguments, which can be multiple.
* `base_condition`: The recursive base case, which when met causes the function to stop recursing.
* `new_input_args`: The new input arguments used in the recursive call.
The advantages of recursive functions include:
* Simplification of code.
* Increased code readability.
* Reduction of code duplication.
# 3.1 Function Design Principles
#### 3.1.1 Modularity and Reusability
Modularity is a technique that breaks down large, complex functions into smaller, manageable modules or sub-functions. This method enhances code reusability, allowing the same modules to be used in different programs, thereby reducing duplicate code and maintenance costs.
```
% Define a module for calculating the area of a circle
function area = circle_area(radius)
area = pi * radius^2;
end
% Define a module for calculating the circumference of a circle
function circumference = circle_circumference(radius)
circumference = 2 * pi * radius;
end
% Use the modules to calculate the area and circumference of a circle
radius = 5;
area = circle_area(radius);
circumference = circle_circumference(radius);
```
#### 3.1.2 Clarity and Readability
Clarity and readability are crucial for writing functions that are easy to understand and maintain. Here are some guidelines to improve code clarity and readability:
- Use meaningful variable names and function names.
- Organize code with indentation and whitespace.
- Use comments to explain complex code segments.
- Avoid lengthy code and nested statements.
```
% A clear and readable function example
function [mean, std_dev] = calculate_stats(data)
% Calculate mean
mean = sum(data) / length(data);
% Calculate standard deviation
std_dev = sqrt(sum((data - mean).^2) / (length(data) - 1));
end
```
# 4. Application Cases of MATLAB Custom Functions
### 4.1 Scientific Computing and Modeling
#### 4.1.1 Numerical Integration and Differentiation
Custom functions are widely used in numerical integration and differentiation. For complex functions, analytical integration or differentiation can be difficult, and custom functions provide flexible methods to approximate these operations.
**Example: Using a custom function for numerical integration**
```matlab
% Define the integration function
f = @(x) exp(-x^2);
% Integration interval
a = -2;
b = 2;
% Integration step size
h = 0.1;
% Use the composite trapezoidal rule for numerical integration
integral = 0;
for x = a:h:b
integral = integral + (h/2) * (f(x) + f(x+h));
end
fprintf('Numerical integration result: %.4f\n', integral);
```
**Code logic analysis:**
* Define the integration function `f(x)`.
* Set the integration interval `[a, b]` and step size `h`.
* Use the composite trapezoidal rule to numerically integrate the function `f(x)` over the interval `[a, b]`.
* Calculate the function values iteratively within the integration interval and accumulate them in the `integral` variable.
* Output the result of numerical integration.
#### 4.1.2 Matrix Operations and Linear Algebra
Custom functions also play an important role in matrix operations and linear algebra. They can implement complex matrix operations, such as solving linear equation systems, calculating eigenvalues and eigenvectors, etc.
**Example: Using a custom function to solve a linear equation system**
```matlab
% Define the coefficient matrix
A = [2 1; 4 3];
% Define the constant vector
b = [1; 2];
% Define a custom function to solve a linear equation system
solve_linear_system = @(A, b) A \ b;
% Solve the linear equation system
x = solve_linear_system(A, b);
fprintf('Solution of the linear equation system:\n');
disp(x);
```
**Code logic analysis:**
* Define the coefficient matrix `A` and the constant vector `b`.
* Define a custom function `solve_linear_system(A, b)`, which uses matrix left division `A \ b` to solve the linear equation system.
* Call the custom function `solve_linear_system` to solve the linear equation system and store the solution in the variable `x`.
* Output the solution to the linear equation system.
### 4.2 Data Processing and Analysis
#### 4.2.1 Data Preprocessing and Feature Extraction
Custom functions are very useful in data preprocessing and feature extraction. They can implement various data operations, such as data cleaning, normalization, and feature selection.
**Example: Using a custom function for data normalization**
```matlab
% Define data
data = [1 2 3; 4 5 6; 7 8 9];
% Define a normalization function
normalize_data = @(data) (data - min(data)) / (max(data) - min(data));
% Normalize data
normalized_data = normalize_data(data);
fprintf('Normalized data:\n');
disp(normalized_data);
```
**Code logic analysis:**
* Define the original data `data`.
* Define a custom function `normalize_data(data)`, which calculates the minimum and maximum values of the data and normalizes the data using these values.
* Call the custom function `normalize_data` to normalize the data and store the result in the variable `normalized_data`.
* Output the normalized data.
#### 4.2.2 Data Visualization and Report Generation
Custom functions can also be used for data visualization and report generation. They can create various charts and graphs, and generate reports containing data analysis results.
**Example: Using a custom function to generate a bar chart**
```matlab
% Define data
data = [***];
% Define a custom function to generate a bar chart
plot_bar_chart = @(data) bar(data);
% Generate a bar chart
plot_bar_chart(data);
title('Bar Chart');
xlabel('Category');
ylabel('Value');
```
**Code logic analysis:**
* Define data `data`.
* Define a custom function `plot_bar_chart(data)`, which uses the `bar` function to generate a bar chart.
* Call the custom function `plot_bar_chart` to generate a bar chart.
* Set the chart title, x-axis, and y-axis labels.
### 4.3 Image Processing and Computer Vision
#### 4.3.1 Image Enhancement and Filtering
Custom functions are widely applied in image enhancement and filtering. They can implement various image processing operations, such as adjusting brightness, sharpening, and noise reduction.
**Example: Using a custom function for image sharpening**
```matlab
% Read in the image
image = imread('image.jpg');
% Define an image sharpening function
sharpen_image = @(image) imsharpen(image, 'Amount', 2);
% Sharpen the image
sharpened_image = sharpen_image(image);
% Display the sharpened image
imshow(sharpened_image);
```
**Code logic analysis:**
* Read in the image `image.jpg`.
* Define a custom function `sharpen_image(image)`, which uses the `imsharpen` function to sharpen the image.
* Call the custom function `sharpen_image` to sharpen the image and store the result in the variable `sharpened_image`.
* Display the sharpened image.
#### 4.3.2 Object Detection and Image Segmentation
Custom functions also play an important role in object detection and image segmentation. They can implement complex algorithms, such as edge detection, contour extraction, and object recognition.
**Example: Using a custom function for edge detection**
```matlab
% Read in the image
image = imread('image.jpg');
% Define an edge detection function
edge_detection = @(image) edge(image, 'canny');
% Perform edge detection
edges = edge_detection(image);
% Display the edge detection results
imshow(edges);
```
**Code logic analysis:**
* Read in the image `image.jpg`.
* Define a custom function `edge_detection(image)`, which uses the `edge` function to perform edge detection on the image.
* Call the custom function `edge_detection` to perform edge detection and store the result in the variable `edges`.
* Display the results of edge detection.
# 5. Expansion and Integration of MATLAB Custom Functions
### 5.1 Integration with Other Languages
#### 5.1.1 Interaction between MATLAB and Python
MATLAB and Python are two widely-used programming languages, each with its own strengths in different fields. Integrating MATLAB with Python can leverage the advantages of both, resulting in more powerful data processing and modeling capabilities.
**Ways to interact between MATLAB and Python:**
- **Using the MATLAB Engine API:** MATLAB provides a Python API that allows Python scripts to directly call MATLAB functions and access MATLAB data.
- **Using third-party libraries:** For example, PyCall and Oct2Py, these libraries provide convenient interfaces for Python to interact with MATLAB.
**Code example:**
```python
# Using PyCall to call a MATLAB function
from pycall import pycall
pycall.call_matlab('my_matlab_function', 1, 2)
# Using Oct2Py to access MATLAB data
import oct2py
oct2py.eval('x = [1, 2, 3]')
print(oct2py.get('x'))
```
#### 5.1.2 MATLAB Interface with C/C++
The integration of MATLAB with C/C++ is useful for applications that require high-performance computing or interaction with external libraries.
**Ways to interact between MATLAB and C/C++:**
- **Using MEX functions:** MEX (MATLAB executable) functions are written in C/C++ and can be called within MATLAB.
- **Using the MATLAB Engine library:** The MATLAB Engine library allows C/C++ programs to access the MATLAB interpreter and data.
**Code example:**
```c++
// Create a MEX function
#include "mex.h"
void mexFunction(int nlhs, mxArray *plhs[], int nrhs, const.mxArray *prhs[]) {
// Get input parameters
double x = mxGetScalar(prhs[0]);
// Calculate result
double y = x * x;
// Return output parameters
plhs[0] = mxCreateDoubleScalar(y);
}
```
### 5.2 Function Libraries and Toolboxes
MATLAB provides a rich collection of function libraries and toolboxes that extend its capabilities and simplify the implementation of specific tasks.
#### 5.2.1 Introduction to MATLAB Official Function Libraries
The MATLAB official function libraries cover a wide range of functions, spanning from mathematical computations to data analysis, image processing, and machine learning.
**Example functions:**
- `solve`: Solve linear equation systems or nonlinear equations
- `fft`: Perform fast Fourier transform
- `imshow`: Display images
- `trainNetwork`: Train neural networks
#### 5.2.2 Installation and Use of Third-Party Toolboxes
In addition to the official function libraries, there are many third-party toolboxes available for MATLAB. These toolboxes provide specialized functionality for specific domains, such as:
**Example toolboxes:**
- **Image Processing Toolbox:** Image processing and analysis
- **Statistics and Machine Learning Toolbox:** Statistics and machine learning
- **Parallel Computing Toolbox:** Parallel computing
**Installation and use of third-party toolboxes:**
1. Download and install the toolbox.
2. Use the `addpath` command in MATLAB to add the toolbox path.
3. Use the functions and objects provided by the toolbox.
**Code example:**
```matlab
% Install the Image Processing Toolbox
install_image_processing_toolbox
% Add toolbox path
addpath(genpath('C:\path\to\Image Processing Toolbox'))
% Use toolbox functions
I = imread('image.jpg');
imshow(I);
```
# 6. Future Development and Trends of MATLAB Custom Functions
### 6.1 Cloud Computing and Parallel Programming
#### 6.1.1 Application of MATLAB on Cloud Computing Platforms
Cloud computing provides MATLAB custom functions with a platform for extension and acceleration. By offloading computational tasks to the cloud, users can access powerful computing resources such as high-performance computing clusters and distributed storage. This allows large and complex MATLAB functions to be executed in a shorter amount of time.
To use MATLAB on cloud computing platforms, users can leverage MATLAB cloud services (MATLAB Online) or deploy MATLAB to cloud virtual machines (VMs). MATLAB Online is a browser-based cloud environment that allows users to run MATLAB functions directly in a browser. It is suitable for lightweight computing tasks that do not require local installation of MATLAB.
For more complex computing tasks, users can deploy MATLAB to cloud VMs. This provides access to the full set of MATLAB capabilities, including parallel computing toolboxes and third-party toolboxes. Users can configure the computing power and storage capacity of VMs according to their needs, optimizing performance and cost.
#### 6.1.2 Implementation of Parallel Computing Technologies in MATLAB
Parallel computing technologies in MATLAB allow functions to execute simultaneously on multiple processors or cores. This can significantly improve the performance of large and computationally intensive functions. MATLAB provides a parallel computing toolbox that includes functions and tools for parallel programming.
Parallel computing technologies in MATLAB include:
- **Parallel pool:** Create a parallel computing environment that allows users to distribute tasks across multiple worker processes.
- **Parallel loop:** Use the `parfor` loop to parallelize loop structures, executing loop iterations simultaneously on multiple processors.
- **GPU computing:** Utilize the parallel processing capabilities of graphics processing units (GPUs) to accelerate computationally intensive tasks.
### 6.2 Artificial Intelligence and Machine Learning
#### 6.2.1 Application of MATLAB in the Field of Artificial Intelligence
MATLAB plays a significant role in the field of artificial intelligence (AI), providing a range of tools and algorithms for developing and deploying AI models. MATLAB supports various AI technologies, including:
- **Machine learning:** MATLAB provides a series of machine learning algorithms for classification, regression, clustering, and dimensionality reduction.
- **Deep learning:** MATLAB integrates with deep learning frameworks such as TensorFlow and PyTorch, allowing users to build and train deep neural networks.
- **Computer vision:** MATLAB offers a series of image processing and computer vision algorithms for object detection, image segmentation, and image classification.
- **Natural language processing:** MATLAB supports natural language processing tasks such as text classification, sentiment analysis, and machine translation.
#### 6.2.2 Implementation of Machine Learning Algorithms in MATLAB
Machine learning algorithms in MATLAB are implemented through `fit` and `predict` functions. The `fit` function is used for training models, while the `predict` function is used for making predictions with trained models.
For example, the following code uses MATLAB's `fitcsvm` function to train a Support Vector Machine (SVM) classification model:
```
% Import data
data = readtable('data.csv');
% Split data into training and test sets
[trainingData, testData] = splitData(data, 0.75);
% Create an SVM classifier
classifier = fitcsvm(trainingData, 'Species', 'KernelFunction', 'rbf');
% Evaluate the classifier using the test set
predictedLabels = predict(classifier, testData);
accuracy = mean(predictedLabels == testData.Species);
```
0
0
相关推荐
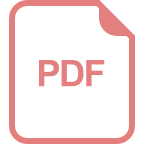
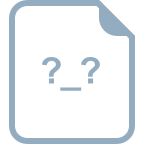
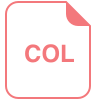
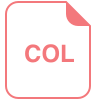
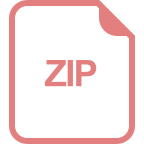
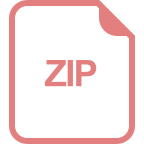
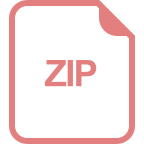
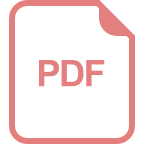