MATLAB Function Exception Handling: Gracefully Managing Errors and Exceptions within Functions
发布时间: 2024-09-14 12:14:46 阅读量: 32 订阅数: 37 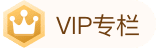
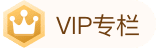
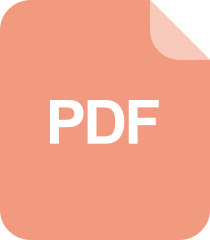
无需编写任何代码即可创建应用程序:Deepseek-R1 和 RooCode AI 编码代理.pdf
# 1. Overview of MATLAB Function Exception Handling
MATLAB function exception handling is a mechanism used to manage and deal with errors and exceptions that occur during the execution of a function. It enables developers to define and throw exceptions within their code, and to catch and handle these exceptions in appropriate places, thereby enhancing the robustness and maintainability of the code.
The exception handling mechanism comprises two primary components: exception throwing and exception catching. Exception throwing is triggered by the `error` or `warning` functions, indicating errors or exceptional conditions encountered during function execution. Exception catching is implemented using `try-catch` statement blocks, allowing developers to specify code to execute when specific exceptions occur.
Through exception handling, developers can control how exceptions are dealt with, preventing errors from propagating up the function call stack, and thereby improving the stability and robustness of the code.
# 2.1 Exception Types and Hierarchy
### Exception Types
Exceptions in MATLAB are divided into two categories:
***Built-in exceptions:** Thrown by the MATLAB kernel, indicating system-level errors or exceptional conditions.
***Custom exceptions:** Defined and thrown by users, indicating application-specific errors or exceptional conditions.
### Exception Hierarchy
Exceptions in MATLAB follow a hierarchy, with each exception class being a subclass of its parent class. The root exception class is `MException`, which represents the base class for all exceptions.
Some common built-in exception classes include:
* `MException`: The root exception class.
* `MATLAB:UndefinedFunctionException`: Undefined function.
* `MATLAB:BadFunctionCallException`: Function call error.
* `MATLAB:IndexOutOfRangeException`: Index out of range.
* `MATLAB:NaNException`: NaN value error.
Users can create their own custom exception classes, inheriting from `MException` or its subclasses. This allows users to define application-specific exception types.
### Exception Identifiers
Each exception has a unique identifier, known as the exception identifier. The exception identifier is a string that identifies the type of exception. For example, the identifier for the `MATLAB:UndefinedFunctionException` exception is `"MATLAB:UndefinedFunctionException"`.
Exception identifiers are used to match exception types in exception handling statements. For example, the following code captures exceptions with the identifier `"MATLAB:UndefinedFunctionException"`:
```matlab
try
% Code block
catch ME when strcmp(ME.identifier, 'MATLAB:UndefinedFunctionException')
% Handle undefined function exception
end
```
# 3.1 Customizing and Throwing Exceptions
In MATLAB, we can customize exception classes to meet specific needs. Custom exception classes allow us to create more descriptive and informative error messages, thereby enhancing the readability and maintainability of the code.
### Steps for Customizing Exception Classes
The steps to customize an exception class are as follows:
1. Create a subclass that inherits from the `MException` class.
2. Define the constructor for the exception class, which accepts a string parameter as the error message.
3. In the constructor of the exception class, call the constructor of the parent class `MException`, passing the error message.
### Example of a Custom Exception Class
Here is an example of a custom exception class:
```matlab
classdef MyCustomException < MException
methods
function obj = MyCustomException(message)
obj = obj@MException('MyCustomException:Error', message);
end
end
end
```
### Throwing a Custom Exception
To throw a custom exception, the `throw` function can be used. The `throw` function accepts an exception object as a parameter and throws it.
### Example of Throwing a Custom Exception
Here is an example of throwing a custom exception:
```matlab
try
% Code block
catch myCustomException
% Code block to handle custom exception
end
```
## 3.2 Catching and Handling Exceptions
Catching and handling exceptions involves using `try-catch` statement blocks to catch and process exceptions. A `try-catch` statement block contains the following parts:
- `try` block: Contains
0
0
相关推荐





