MATLAB Custom Functions: Mastering Function Definition, Invocation, and Parameter Passing
发布时间: 2024-09-14 11:55:23 阅读量: 33 订阅数: 37 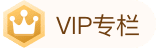
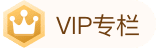
# 1. Overview of MATLAB Functions
A MATLAB function is a reusable block of code designed to encapsulate code and perform specific tasks. Functions define input and output parameters, allowing users to organize code in a modular and reusable way. Functions can enhance the readability, maintainability, and scalability of the code.
# 2. Function Definition
### 2.1 Function Declaration and Syntax
The definition of a MATLAB function starts with the keyword `function`, followed by the function name and a pair of parentheses, which enclose the function's parameter list. The function body is enclosed in a pair of curly braces {}, containing the function's statement block.
```matlab
function [output_args] = function_name(input_args)
% Function body
% ...
end
```
**Parameter Explanation:**
* `function_name`: The function name must be a valid MATLAB identifier.
* `input_args`: The function parameter list can have multiple parameters, separated by commas.
* `output_args`: The function return value list can have multiple return values, enclosed in square brackets.
### 2.2 Function Body and Statement Block
The function body is the execution part of the function code, composed of a series of statement blocks. A statement block ends with the keyword `end`, indicating the end of the function body.
```matlab
function [output_args] = function_name(input_args)
% Function body
% Statement block 1
% ...
% Statement block 2
% ...
% ...
% Statement block n
% ...
end
```
**Code Logic Analysis:**
The statement blocks in the function body execute sequentially. Each block usually performs a specific task, such as computation, conditional judgment, or input/output operations.
### 2.3 Function Parameters and Return Values
Function parameters are used to pass data to the function, and return values are used to return data from the function.
**Function Parameters**
Function parameters are specified in the function declaration and can have multiple parameters separated by commas. Parameter types can be scalar, vector, matrix, or structure.
**Function Return Values**
Function return values are specified in the function declaration and can have multiple return values enclosed in square brackets. Return value types can be scalar, vector, matrix, or structure.
**Code Example:**
```matlab
% Define a function to calculate the area of a circle
function area = circle_area(radius)
% Function body
area = pi * radius^2;
end
% Call the function and get the return value
radius = 5;
area = circle_area(radius);
% Output the result
fprintf('The area of the circle is: %.2f\n', area);
```
**Code Logic Analysis:**
* The `circle_area` function accepts one parameter `radius`, which represents the radius of the circle.
* The function body calculates the area of the circle and stores it in the variable `area`.
* The `area` variable is returned as the function's return value.
* In the function call, the radius `radius` is passed as a parameter to the function, and the function's return value `area` is obtained.
* Finally, the calculated circle area is output.
# 3. Function Calling
### 3.1 Function Calling Syntax
A function call is completed by using the function name and its parameter list. The syntax for a function call is as follows:
```
function_name(input_arguments)
```
Where:
* `function_name` is the name of the function to be called.
* `input_arguments` is the list of parameters passed to the function, separated by commas.
For example, to call a function named `my_function` and pass two parameters `x` and `y`, the syntax would be:
```
my_function(x, y)
```
### 3.2 Parameter Passing Mechanism
The parameter passing mechanism in MATLAB is divided into two types: value passing and reference passing.
#### 3.2.1 Value Passing
Value passing involves copying the value of the parameter into the function. This means that any modifications to the parameter within the function will not affect the original value outside the function.
```matlab
function my_function(x)
x = x + 1;
end
x = 10;
my_function(x);
disp(x) % Outputs: 10
```
In the example above, the original value of `x` is 10. When `my_function` is called, the value of `x` is copied into the function. Any modifications to `x` within the function do not affect the original value outside the function, so `disp(x)` outputs 10.
#### 3.2.2 Reference Passing
Reference passing involves passing the reference of the parameter into the function. This means that any modifications to the parameter within the function will affect the original value outside the function.
```matlab
function my_function(x)
x(1) = x(1) + 1;
end
x = [1, 2, 3];
my_function(x);
disp(x) % Outputs: [2, 2, 3]
```
In the example above, the original value of `x` is an array `[1, 2, 3]`. When `my_function` is called, the reference of `x` is passed into the function. Modifications to `x` within the function affect the original value outside the function, so `disp(x)` outputs `[2, 2, 3]`.
### 3.2.3 Selection of Parameter Passing Mechanism
When choosing a parameter passing mechanism, consider the following factors:
***Data Type:** Value passing is suitable for immutable data types such as scalars and strings. Reference passing is suitable for mutable data types such as arrays and structures.
***Function Behavior:** If the function needs to modify parameters, reference passing should be used. If the function does not need to modify parameters, value passing should be used.
***Efficiency:** Value passing is more efficient than reference passing because it does not require copying the value of the parameters.
### 3.2.4 Parameter Types
The parameters of MATLAB functions can be of the following types:
***Input Parameters:** Values passed to the function.
***Output Parameters:** Values returned by the function.
***Input/Output Parameters:** Values passed as both input and output parameters.
### 3.2.5 Variable Number of Parameters
MATLAB functions can accept a variable number of parameters. These parameters are represented using the `varargin` keyword.
```matlab
function my_function(varargin)
for i = 1:length(varargin)
disp(varargin{i})
end
end
my_function('Hello', 'World', 10)
```
In the example above, `my_function` can accept any number of parameters. `varargin` is a cell array containing all the parameters passed to the function.
# 4. Function Debugging and Optimization
### 4.1 Function Debugging Methods
**4.1.1 Breakpoint Debugging**
Breakpoint debugging is a method to pause the execution at a specific location during code execution, allowing the inspection of variable values and program flow. In MATLAB, the `dbstop` function can be used to set breakpoints.
```matlab
% Set breakpoint
dbstop in myFunction at 15
% Run code
myFunction()
```
When the code execution reaches line 15, the program will pause, and the debugger window will open. You can inspect variable values, set watchpoints, and step through the code in the debugger window.
**4.1.2 Output Debugging**
Output debugging involves adding `disp` or `fprintf` statements to the code to print variable values or messages. This helps track program execution and identify issues.
```matlab
% Output debugging
disp('Current value of x:')
disp(x)
```
### 4.2 Function Optimization Tips
**4.2.1 Vectorized Computation**
Vectorized computation involves using MATLAB's vector and matrix operations instead of loops. This can significantly improve the performance of the code.
```matlab
% Loop computation
for i = 1:100
y(i) = sin(x(i));
end
% Vectorized computation
y = sin(x);
```
**4.2.2 Avoid Unnecessary Loops**
Unnecessary loops can degrade the performance of the code. Loops can be avoided by using logical indexing or boolean operations.
```matlab
% Unnecessary loop
for i = 1:100
if x(i) > 0
y(i) = x(i);
end
end
% Avoid unnecessary loops
y = x(x > 0);
```
# 5. Function Libraries and Third-Party Functions
### 5.1 MATLAB Built-in Function Libraries
MATLAB provides a rich set of built-in function libraries covering a variety of mathematical, scientific, data processing, and visualization functionalities. These functions are optimized to perform common tasks efficiently, saving development time and effort.
#### Accessing Built-in Functions
To access built-in functions, simply enter the function name in the MATLAB command line or script. For example, to compute a sine value, the following command can be used:
```matlab
y = sin(x);
```
#### Classification of Built-in Functions
MATLAB's built-in function library is organized into the following categories:
- **Mathematical Functions:** Trigonometric functions, exponential functions, logarithmic functions, etc.
- **Statistical Functions:** Mean, standard deviation, regression analysis, etc.
- **Data Processing Functions:** Sorting, filtering, aggregation, etc.
- **Visualization Functions:** Plotting, charting, image processing, etc.
- **Others:** File operations, string processing, dates and times, etc.
### 5.2 Third-Party Function Libraries
In addition to MATLAB's built-in function libraries, there are many third-party function libraries available. These function libraries provide a wider range of functionalities, including:
- **Domain-specific toolboxes:** Image processing, signal processing, machine learning, etc.
- **General utilities:** Data structures, algorithms, file operations, etc.
- **Community contributions:** Functions created and shared by users.
#### Installation and Usage of Third-Party Functions
To install a third-party function library, use MATLAB File Exchange or other online resources. After installation, follow these steps to use these functions:
1. **Add Path:** Use the `addpath` command to add the function library's directory to the MATLAB path.
2. **Load Function:** Use the `load` command to load the required function.
3. **Call Function:** Call the third-party function just like a built-in function, using the function name.
#### Creating Your Own Function Library
Users can also create their own function libraries to organize and reuse custom functions. To create a function library, simply store the functions in a folder and use the `save` command to save it as a `.mat` file. To load the function library, use the `load` command.
### 5.2.1 Installation and Usage of Third-Party Functions
**Installation of Third-Party Function Libraries**
The installation of third-party function libraries can be done through the following steps:
1. **Find the Function Library:** Search for the desired function library on MATLAB File Exchange or other online resources.
2. **Download the Function Library:** Download the `.zip` or `.tar` file of the function library.
3. **Unzip the Function Library:** Extract the downloaded file to a local directory.
**Usage of Third-Party Function Libraries**
After installing third-party function libraries, follow these steps to use their functions:
1. **Add Path:** Use the `addpath` command to add the function library's directory to the MATLAB path. For example:
```matlab
addpath('path/to/function_library');
```
2. **Load Function:** Use the `load` command to load the required functions. For example:
```matlab
load('function_library.mat');
```
3. **Call Function:** Call the third-party function just like a built-in function, using the function name. For example:
```matlab
y = my_custom_function(x);
```
### 5.2.2 Creating Your Own Function Library
**Creating a Function Library**
To create your own function library, follow these steps:
1. **Create a Folder:** Create a folder to store the functions.
2. **Save Functions:** Save the custom functions in that folder, using the `.m` extension.
3. **Save the Function Library:** Use the `save` command to save all the functions in the folder as a `.mat` file. For example:
```matlab
save('my_function_library.mat');
```
**Loading the Function Library**
To load the function library, follow these steps:
1. **Add Path:** Use the `addpath` command to add the function library's directory to the MATLAB path. For example:
```matlab
addpath('path/to/my_function_library');
```
2. **Load the Function Library:** Use the `load` command to load the function library. For example:
```matlab
load('my_function_library.mat');
```
# 6. Function Design Principles
### ***
***anizing code into independent function modules can improve the readability, maintainability, and reusability of the code.
***Readability:** Modular code breaks down complex tasks into smaller, more understandable units, making the code easier to read and comprehend.
***Maintainability:** Modular code is easier to maintain and update because individual functions can be modified or replaced without affecting other parts of the code.
***Reusability:** Modular functions can be reused across different programs and projects, saving time and effort.
### 6.2 Readability and Maintainability
Readability and maintainability are essential considerations in function design. Here are some best practices to improve the readability and maintainability of functions:
***Clear Naming:** Choose meaningful and descriptive names for functions, variables, and parameters to clearly convey their purpose.
***Comments:** Use comments to explain the behavior of the function, its parameters, and return values.
***Proper Indentation:** Use appropriate indentation to organize the code and improve readability.
***Error Handling:** Handle potential errors and provide meaningful error messages to help users debug and fix problems.
### 6.3 Performance and Efficiency
Function design should also consider performance and efficiency. Here are some tips to improve the performance and efficiency of functions:
***Avoid Unnecessary Calculations:** Only perform calculations when needed and avoid repeated calculations.
***Use Preallocation:** Preallocate variables to avoid dynamically allocating memory within loops.
***Vectorized Computation:** Utilize MATLAB's vectorization capabilities to improve the performance of loops.
***Leverage Parallel Computing:** If possible, take advantage of MATLAB's parallel computing features to increase computation speed.
0
0
相关推荐








