MATLAB Function File Operations: Tips for Reading, Writing, and Manipulating Files with Functions
发布时间: 2024-09-14 12:21:25 阅读量: 30 订阅数: 31 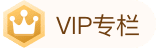
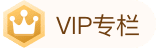
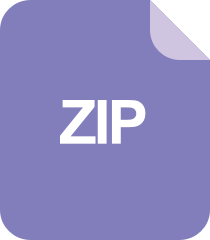
Series of MATLAB scripts and functions for manipulating ADCIRC
# 1. Overview of MATLAB Function File Operations
MATLAB function file operations refer to a set of functions in MATLAB designed for handling files. These functions enable users to create, read, write, modify, and delete files, as well as retrieve file attributes. Function file operations are crucial for data storage, file management, and interaction with external systems.
MATLAB function file operations can be categorized into three types:
* File I/O functions: Used for creating, reading, and writing file contents.
* File attribute functions: Used for retrieving and modifying file attributes, such as file size, creation date, and access permissions.
* Advanced file operation functions: Used for executing more complex operations, such as file positioning, moving, copying, and deleting.
# 2. MATLAB Function File Read/Write Operations
### 2.1 File I/O Functions
#### 2.1.1 fopen() Function
**Function:** Opens a file and returns a file identifier (fileID).
**Syntax:**
```matlab
fileID = fopen(filename, mode)
```
**Parameters:**
- `filename`: The name of the file to open, including its path.
- `mode`: The mode for opening the file, specifying read/***mon modes include:
- `'r'`: Read-only
- `'w'`: Write-only, overwrites the file if it exists
- `'a'`: Append, adds to the end of the file if it exists
- `'r+'`: Read and write, the file must exist
- `'w+'`: Read and write, overwrites the file if it exists
- `'a+'`: Read and write, adds to the end of the file if it exists
**Return Value:**
- `fileID`: The file identifier for subsequent file operations. Returns -1 if the file fails to open.
**Logical Analysis:**
The `fopen()` function first checks if the file exists, then opens the file according to the specified mode. If the file does not exist, it creates the file. If the file exists, it opens the file in the manner specified by the mode.
#### 2.1.2 fwrite() and fread() Functions
**Function:** Used for writing data to a file and reading data from a file, respectively.
**Syntax:**
**fwrite()**
```matlab
fwrite(fileID, data, format)
```
**fread()**
```matlab
data = fread(fileID, size, format)
```
**Parameters:**
**fwrite()**
- `fileID`: File identifier.
- `data`: Data to write to the file.
- `format`: Data format specifying the type of data to write.
**fread()**
- `fileID`: File identifier.
- `size`: The size of the data to read, in bytes.
- `format`: Data format specifying the type of data to read.
**Return Value:**
**fwrite()**
- Returns the number of bytes written to the file.
**fread()**
- Returns the data read.
**Logical Analysis:**
**fwrite()** function writes data to a file and converts it to binary form according to the specified format. **fread()** function reads data from a file and converts it to MATLAB data types according to the specified format.
#### 2.1.3 fclose() Function
**Function:** Closes a file.
**Syntax:**
```matlab
fclose(fileID)
```
**Parameters:**
- `fileID`: The file identifier to close.
**Logical Analysis:**
The `fclose()` function closes a file, releasing system resources associated with the file. It is essential to close files after completing file operations.
### 2.2 Examples of File Content Read/Write
#### 2.2.1 Reading Data from a File
```matlab
% Open the file
fileID = fopen('data.txt', 'r');
% Read the file contents
data = fread(fileID, inf, '*char');
% Close the file
fclose(fileID);
% Convert the data to a string
data = char(data');
```
**Logical Analysis:**
This example first uses the `fopen()` function to open a file named `data.txt`. Then, it uses the `fread()` function to read all character data from the file. Finally, it uses the `fclose()` function to close the file.
#### 2.2.2 Writing Data to a File
```matlab
% Open the file
fileID = fopen('data.txt', 'w');
% Write data
fwrite(fileID, 'Hello World!', '*char');
% Close the file
fclose(fileID);
```
**Logical Analysis:**
This example first uses the `fopen()` function to open a file named `data.txt` and specify the write mode. Then, it uses the `fwrite()` function to write the string `'Hello World!'` to the file. Finally, it uses the `fclose()` function to close the file.
# 3. MATLAB Function File Attribute Operations**
**3.1 File Attribute Functions**
MATLAB provides various functions to get and modify file attributes, including:
- **dir() function:** Retrieves information about files and folders in a specified directory, returning an array of structure elements containing the names, sizes, dates, and other information about the files and folders.
- **fileattrib() functi
0
0
相关推荐
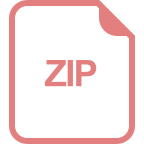
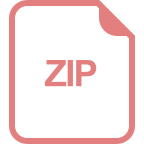
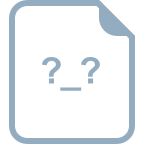
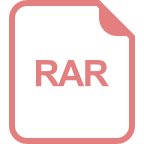
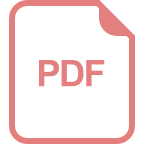
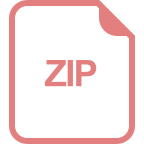
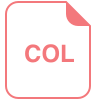
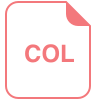