MATLAB Function Deployment: Seamlessly Integrating Functions into Applications and Systems
发布时间: 2024-09-14 12:09:32 阅读量: 33 订阅数: 33 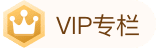
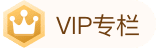
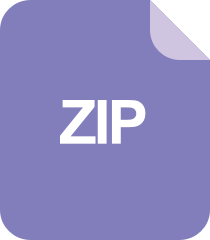
matlab分时代码-azurefunction_matlab_deployment:azurefunction_matlab_deploym
# 1. Overview of MATLAB Function Deployment
## 1.1 Importance and Advantages of Function Deployment
Function deployment in MATLAB enables the seamless integration of MATLAB functions into other applications and systems. This process offers several benefits:
***Code Reusability:** Deploy MATLAB functions as standalone modules for reuse in different applications and systems.
***Cross-Platform Compatibility:** Functions can be deployed across various platforms, including Windows, Linux, and macOS, ensuring compatibility.
***Efficiency:** Deployed MATLAB functions can enhance the performance of applications and systems by leveraging MATLAB's high-performance computing capabilities.
## 1.2 Deployment Options and Best Practices
MATLAB function deployment provides a range of options, such as:
***Packaging Toolboxes:** Bundle MATLAB functions into standalone toolboxes for easy distribution and deployment.
***MATLAB Engine:** Integrate MATLAB functions into Java and C/C++ applications using the MATLAB Engine.
***Web Services:** Deploy MATLAB functions as web services for HTTP-based access.
***Cloud Deployment:** Deploy MATLAB functions to cloud platforms, such as AWS and Azure, for scalability and high availability.
The optimal deployment option depends on specific requirements of the application or system, including performance, compatibility, and security.
# 2. Fundamentals of MATLAB Function Deployment
## 2.1 Function Packaging and Publishing
### 2.1.1 MATLAB Packaging Toolboxes
MATLAB provides a feature for packaging toolboxes that allows users to bundle functions, data, and documentation into a single distributable package. A toolbox can include:
* MATLAB functions
* Data files
* Documentation
* Examples and test scripts
The process of packaging a toolbox involves steps like:
```
>> matlabFunctionToolbox('myToolbox', {'myFunction1', 'myFunction2'}, ...
'TargetDirectory', 'myToolboxDirectory');
```
* `myToolbox`: Name of the toolbox
* `{'myFunction1', 'myFunction2'}`: List of functions to include in the toolbox
* `myToolboxDirectory`: Directory where the toolbox will be saved
### 2.1.2 Deployment Options and Considerations
When packaging toolboxes, consider the following deployment options and considerations:
***Dependencies:** Ensure the toolbox contains all dependencies for deployment, including external libraries and data files.
***Licenses:** If the toolbox includes licensed code or data, ensure compliance with license terms.
***Platform Compatibility:** Consider the target deployment platform's compatibility and package the toolbox accordingly.
***Version Control:** Use version control systems to manage changes to the toolbox for collaboration and updates.
## 2.2 Function Interface Design
### 2.2.1 Defining Input/Output Parameters
The interface design of MATLAB functions is crucial for deployment. Input and output parameters should be clearly defined and follow these guidelines:
***Typed Parameters:** Specify parameter types using MATLAB data types (e.g., `double`, `char`).
***Documented Parameters:** Use `@param` and `@return` tags in function documentation to record descriptions of parameters and return values.
***Validated Parameters:** Use `nargin` and `nargout` to check parameter counts and use `assert` or `validateattributes` to verify parameter types and values.
### 2.2.2 Function Documentation and Comments
Clear function documentation and comments are essential for understanding and using deployed functions. Documentation should include:
* Purpose and functionality of the function
* Descriptions of input and output parameters
* Usage examples
* A brief explanation of the algorithm or implementation
Comments should be embedded in the code to explain the logic and implementation of specific function parts.
# 3. Integrating MATLAB Functions into Applications
### 3.1 Integration into Java Applications
#### 3.1.1 Java Native Interface (JNI)
The Java Native Interface (JNI) is an API that allows Java applications to interact with native code. Through JNI, Java applications can invoke MATLAB functions, thereby integrating MATLAB capabilities into Java applications.
**Code Block:**
```java
import com.mathworks.engine.Engine;
import com.mathworks.engine.EngineException;
import com.mathworks.engine.MatlabEngine;
public class JavaApp {
public static void main(String[] args) throws EngineException {
// Create a MATLAB engine
Engine engine = MatlabEngine.startMatlab();
// Invoke MATLAB function
double[] x = {1, 2, 3, 4, 5};
double[] y = engine.feval("polyfit", x, y, 1);
// Print results
for (double value : y) {
System.out.println(value);
}
// Close the MATLAB engine
engine.close();
}
}
```
**Logical Analysis:**
* The `MatlabEngine.startMatlab()` method creates a MATLAB engine.
* The `feval` method calls a MATLAB function, where the first argument is the function name, and subsequent arguments are the function inputs.
* A loop prints the results returned by the function.
* The `engine.close()` method closes the MATLAB engine.
#### 3.1.2 MATLAB Engine for Java
The MATLAB Engine for Java is a MATLAB toolbox that provides a higher-level API for integrating MATLAB with Java applications. It simplifies the use of JNI and offers additional features such as object passing and event handling.
**Code Block:**
```java
import com.mathworks.matlab.engine.MatlabEngine;
import com.mathworks.matlab.engine.Ma
```
0
0
相关推荐






