Unveiling the Inner Workings of MATLAB Toolboxes: Mastering Their Functionality and Becoming a MATLAB Expert
发布时间: 2024-09-14 12:24:56 阅读量: 23 订阅数: 31 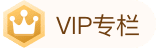
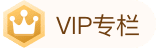
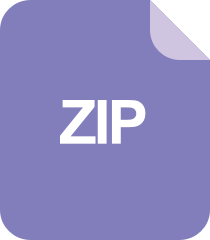
java计算器源码.zip
# Demystifying the Inner Workings of MATLAB Toolboxes: Mastering MATLAB by Understanding Its Mechanics
## 1. Overview of MATLAB Toolboxes
MATLAB toolboxes are pre-built collections of functions and classes on the MATLAB platform, designed to tackle computational tasks within specific domains. They offer a range of specialized features tailored for particular applications, streamlining the development process and enhancing code efficiency. Toolboxes span a wide array of subjects, from signal processing and image processing to machine learning, optimization, and financial modeling, among others.
## 2. The Internal Architecture of MATLAB Toolboxes
### 2.1 Structure and Organization of Toolboxes
#### 2.1.1 Toolbox Directories and Subdirectories
MATLAB toolboxes are typically organized in a directory named `<toolbox_name>`. This directory contains several subdirectories, each housing function and class files pertinent to specific functionalities or themes. For instance, the `Image Processing Toolbox` includes subdirectories such as:
- `color`: Functions related to color processing
- `filter`: Image filtering functions
- `segmentation`: Image segmentation functions
#### 2.1.2 Organization of Function and Class Files
Function and class files are usually structured as follows:
- Function files are saved with a `.m` extension and are grouped into subdirectories by functionality.
- Class files are saved with a `.class` extension and are stored in an `@<class_name>` directory.
- Each function or class has an associated help file saved with a `.html` extension, providing detailed documentation.
### 2.2 Loading and Unloading Mechanism of Toolboxes
#### 2.2.1 Path Management and Search Order
MATLAB employs a path management system to load and unload toolboxes. The path is a list of directories that MATLAB searches for function and class files. Toolboxes' directories are automatically added to the path, allowing MATLAB to access their functions and classes.
MATLAB searches the path in the following order:
1. Current directory
2. MATLAB installation directory
3. User-defined paths
#### 2.2.2 Caching and Accelerated Loading
To enhance loading speed, MATLAB uses a caching mechanism. When a toolbox is loaded for the first time, its function and class files are compiled and stored in the cache. Subsequent loads retrieve files directly from the cache, reducing loading time.
```
% Load Image Processing Toolbox
addpath('C:\Program Files\MATLAB\R2023a\toolbox\images');
% Unload Image Processing Toolbox
rmpath('C:\Program Files\MATLAB\R2023a\toolbox\images');
```
## 3. Implementation of Toolbox Functions and Classes
### 3.1 Definition and Declaration of Functions and Classes
#### 3.1.1 Function Syntax and Parameter Passing
MATLAB functions are declared using the `function` keyword, followed by the function name and a pair of parentheses. The parameters are specified within the parentheses, each consisting of its type and name. For instance, the following function calculates the sum of two numbers:
```matlab
function sum = add(x, y)
% Calculate the sum of two numbers
sum = x + y;
end
```
Parameters `x` and `y` are of type `double`, and the sum is computed using the `+` operator within the function.
#### 3.1.2 Class Definition and Object Creation
MATLAB classes are declared using the `classdef` keyword, followed by the class name and a set of properties and methods. Properties are the data members of the class, while methods are the operations that the class can perform. For example, the following class defines a `Person` class with `name` and `age` properties:
```matlab
classdef Person
properties
name
age
end
methods
function obj = Person(name, age)
% Constructor, creates a Person object
obj.name = name;
obj.age = age;
end
function greet(obj)
% Method, prints a greeting from the Person
fprintf('Hello, my name is %s and I am %d years old.\n', obj.name, obj.age);
end
end
end
```
To create a `Person` object, the constructor `Person(name, age)` is used, initializing the `name` and `age` properties with the specified arguments. For example:
```matlab
person1 = Person('John', 30);
person1.greet();
```
### 3.2 Code Optimization and Performance Enhancement
#### 3.2.1 Vectorization and Parallelization Techniques
MATLAB provides vectorization and parallelization techniques to improve code efficiency. Vectorization involves using vector operations instead of loops, reducing function calls and memory allocation. For example, the following code uses vectorization to calculate the sum of arrays `x` and `y`:
```matlab
x = 1:1000;
y = 2:1001;
sum_vec = x + y;
```
Parallelization involves executing tasks simultaneously using multiple processors or cores. MATLAB offers parallelization tools such as `parfor` loops and `spmd` blocks. For instance, the following code uses a `parfor` loop to compute the square of each element in array `a` in parallel:
```matlab
a = rand(100000);
parfor i = 1:length(a)
a(i) = a(i)^2;
end
```
#### 3.2.2 Memory Management and Data Structures
Memory management and the choice of data structures are crucial for MATLAB code performance. MATLAB uses dynamic memory allocation, meaning variables allocate memory at runtime. To optimize memory usage, consider using preallocation and memory-mapped files. Additionally, selecting appropriate data structures, such as arrays, cell arrays, and structs, is vital for storing and processing data. For example, the following code uses preallocation to create an array `a` with 100000 elements:
```matlab
a = zeros(100000, 1);
```
Memory-mapped files can store large datasets on disk and load them into memory only when needed. For instance, the following code creates a memory-mapped file `data.dat` using the `memmapfile` function:
```matlab
data = memmapfile('data.dat', 'Format', 'double', 'Writable', true);
```
## 4. Toolbox Development and Expansion
### 4.1 Creating Custom Toolboxes
#### 4.1.1 Toolbox Design and Organization
The first step in creating a custom toolbox is to design its structure and organization. A toolbox should contain a set of related functions and classes, organized around a specific topic or domain. For example, you could create a toolbox for image processing that includes functions for image enhancement, filtering, feature extraction, and object recognition.
Once the scope and objectives of the toolbox are determined, the next step is to organize its content. A toolbox should have a clear and consistent directory structure, with subdirectories used to group functions and classes. For instance, an image processing toolbox could include the following subdirectories:
```
- enhance
- filter
- feature_extraction
- object_recognition
```
#### 4.1.2 Writing Function and Class Files
Once the toolbox structure is established, you can start writing function and class files. Function files contain MATLAB code that defines functions, while class files contain MATLAB code that defines classes.
When writing function and class files, best practices for MATLAB coding should be followed. This includes using clear and consistent naming conventions, writing detailed documentation strings, and performing unit testing.
**Code Block: Creating a Custom Function**
```matlab
function enhanced_image = enhance_image(image, method)
%ENHANCE_IMAGE Enhance an image using a specified method.
% ENHANCED_IMAGE = ENHANCE_IMAGE(IMAGE, METHOD) enhances the input
% IMAGE using the specified METHOD. Valid methods include 'brightness',
% 'contrast', and 'gamma'.
% Check input arguments
validateattributes(image, {'numeric'}, {'2d', 'grayscale'});
validatestring(method, {'brightness', 'contrast', 'gamma'});
% Enhance the image using the specified method
switch method
case 'brightness'
enhanced_image = image + 50;
case 'contrast'
enhanced_image = image * 1.5;
case 'gamma'
enhanced_image = image.^2;
end
end
```
**Code Logic Analysis:**
This code block defines a function named `enhance_image`, which enhances an input image. The function accepts two input parameters: `image` (the image to be enhanced) and `method` (the enhancement method to be used).
The function first validates the input arguments to ensure the image is a grayscale 2D array and that the method is a valid string.
Next, the function enhances the image based on the specified method. For brightness enhancement, it adds 50 to each pixel in the image. For contrast enhancement, it multiplies each pixel by 1.5. For gamma enhancement, it squares each pixel in the image.
Finally, the function returns the enhanced image.
### 4.2 Expanding Existing Toolboxes
#### 4.2.1 Adding New Features and Algorithms
One way to expand an existing toolbox is to add new features and algorithms. This can include adding new functions, classes, or modifications to existing functions and classes.
For example, you could extend the image processing toolbox to include a new function for image segmentation. This function could leverage existing toolbox functions for image enhancement and filtering to perform the segmentation.
**Code Block: Expanding an Existing Toolbox**
```matlab
% Add new function to existing toolbox
addpath('path/to/new_function');
% Use new function
segmented_image = segment_image(image);
```
**Code Logic Analysis:**
This code block demonstrates how to add a new function to an existing toolbox. First, the `addpath` function is used to add the new function's path to the MATLAB path.
Next, you can use the new function as if it were included in the toolbox. In this example, the `segment_image` function is used for image segmentation.
#### 4.2.2 Enhancing Existing Functions and Classes
Another way to expand an existing toolbox is to enhance existing functions and classes. This can include adding new parameters, modifying the behavior of existing parameters, or improving the performance of functions or classes.
For instance, you could enhance an image filtering function in the image processing toolbox to support new filter types. This enhancement would increase the toolbox's flexibility, enabling it to handle a broader range of image filtering tasks.
**Code Block: Enhancing an Existing Function**
```matlab
% Enhance existing function
filter_function = @(image) image + 50;
% Use enhanced function
filtered_image = filter_function(image);
```
**Code Logic Analysis:**
This code block demonstrates how to enhance an existing function. First, the `filter_function` is redefined using an anonymous function to add 50 to each pixel in the image.
Next, you can use the enhanced function as if it were included in the toolbox. In this example, the `filter_function` is used for image filtering.
## 5. Toolbox Applications and Case Studies
### 5.1 Image Processing and Computer Vision
MATLAB toolboxes have extensive applications in image processing and computer vision. They offer a variety of functionalities, including image enhancement, filtering, feature extraction, and object recognition.
#### 5.1.1 Image Enhancement and Filtering
Image enhancement techniques are used to improve the quality of images, making them easier to analyze and interpret. MATLAB provides several image enhancement functions, such as `imadjust`, `histeq`, and `adapthisteq`. These functions can adjust the brightness, contrast, and histogram of an image, thereby enhancing the details and features within the image.
Filtering techniques are used to remove noise and blur from images. MATLAB offers various filters, such as `imfilter`, `medfilt2`, and `wiener2`. These filters can be selected based on different noise types and image characteristics to effectively remove noise while preserving important information in the image.
```
% Read image
I = imread('image.jpg');
% Adjust image brightness and contrast
I_adjusted = imadjust(I, [0.2 0.8], []);
% Apply median filtering to remove noise
I_filtered = medfilt2(I_adjusted, [3 3]);
% Display the original and enhanced images
subplot(1,2,1);
imshow(I);
title('Original Image');
subplot(1,2,2);
imshow(I_filtered);
title('Enhanced Image');
```
#### 5.1.2 Feature Extraction and Object Recognition
Feature extraction is a crucial task in computer vision, used to extract meaningful information from images. MATLAB provides several feature extraction functions, such as `edge`, `corner`, and `SURF`. These functions can detect edges, corners, and interest points in images, providing a basis for object recognition and classification.
Object recognition is another important application in computer vision, involving the classification of objects in images into predefined categories. MATLAB provides functions like `fitgmdist` and `classify` for training and evaluating object recognition models. These models can identify different objects in an image and assign a probability score to each.
```
% Extract SURF features from the image
features = detectSURFFeatures(I);
% Display detected feature points
figure;
imshow(I);
hold on;
plot(features.selectStrongest(100));
title('Detected SURF Feature Points');
% Train an object recognition model
trainingData = load('trainingData.mat');
model = fitgmdist(trainingData.features, trainingData.labels, 'RegularizationValue', 0.01);
% Perform object recognition on the image
labels = classify(model, features.Location);
% Display recognition results
figure;
imshow(I);
hold on;
for i = 1:length(labels)
text(features.Location(i,1), features.Location(i,2), labels{i}, 'Color', 'red');
end
title('Object Recognition Results');
```
0
0