Error Handling and Debugging in MATLAB Toolboxes: Quickly Solve Problems and Make Your Code More Stable
发布时间: 2024-09-14 12:30:46 阅读量: 28 订阅数: 25 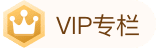
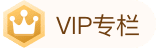
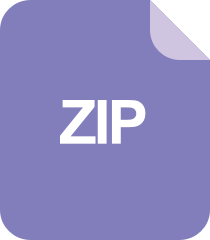
jjq-ch10-error-handling-and-debugging
# 1. Basic Error Handling in MATLAB Toolboxes
MATLAB toolboxes offer the capability to manage and handle errors within code, ensuring the robustness and reliability of programs. The fundamentals of error handling include:
- **Error Types:** There are two primary error types in MATLAB: runtime errors and syntax errors. Runtime errors occur during program execution, while syntax errors occur at compile time.
- **Error Objects:** Errors in MATLAB are represented as `MException` objects, which contain detailed information about the error, including the error identifier, message, and stack trace.
- **Error Handling Blocks:** MATLAB provides `try-catch` blocks to handle errors. The `try` block contains code that may produce errors, while the `catch` block contains code to handle the errors.
# 2. Tips for Error Handling in MATLAB Toolboxes
### 2.1 Exception Handling Mechanism
The exception handling mechanism in MATLAB allows you to catch and handle errors that occur during code execution. It provides fine-grained control over error handling, enabling you to handle exceptions gracefully and robustly.
#### 2.1.1 try-catch-end Blocks
The `try-catch-end` block is the primary mechanism for handling exceptions in MATLAB. The `try` block contains code that may trigger an exception, and the `catch` block contains code to handle the exception. The `end` block is used to terminate the `try-catch-end` structure.
```
try
% Code that may cause an exception
catch
% Code to handle the exception
end
```
#### 2.1.2 throw and rethrow Statements
The `throw` statement is used to manually raise an exception. It can be used to create custom exceptions or to re-raise caught exceptions. The `rethrow` statement is used to re-raise a caught exception.
```
try
% Code that may cause an exception
catch
% Code to handle the exception
rethrow(lasterror); % Reraise the exception
end
```
### 2.2 Error Message Handling
MATLAB provides powerful functions to handle error messages. These functions allow you to obtain detailed information about exceptions and format and display error messages in a user-friendly manner.
#### 2.2.1 getReport and lasterror Functions
The `getReport` function returns a structure containing detailed information about the exception, including the error message, stack trace, and exception identifier. The `lasterror` function returns the error report structure of the most recent exception.
```
try
% Code that may cause an exception
catch
errorReport = getReport; % Get the exception's error report
errorMessage = errorReport.message; % Get the error message
end
```
#### 2.2.2 Formatting and Customizing Error Messages
MATLAB allows you to customize the appearance and content of error messages. You can format error messages using the `sprintf` function and use the `error` function to throw custom error messages.
```
try
% Code that may cause an exception
catch
errorMessage = sprintf('Error: %s', lasterror.message); % Format the error message
error(errorMessage); % Throw a custom error message
end
```
### 2.3 Debugging Tools
MATLAB offers powerful debugging tools to help you identify and resolve errors. These tools allow you to set breakpoints, inspect variable values, and step through code.
#### 2.3.1 dbstop and dbcont Commands
The `dbstop` command is used to set breakpoints; when code execution reaches the breakpoint, the program pauses. The `dbcont` command is used to continue program execution.
```
dbstop if error % Set a breakpoint to pause when an error occurs
dbcont
```
0
0
相关推荐
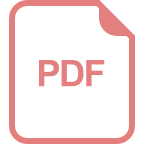
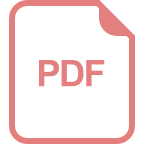
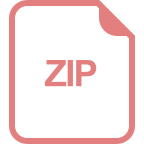
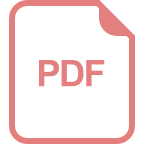
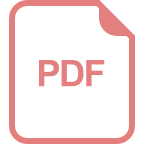
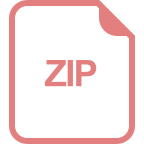
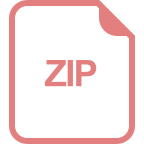
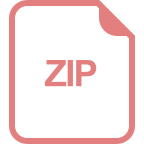