Application of MATLAB Toolboxes Across Various Fields: Exploring Their Broad Use and Solving Industry-Specific Challenges
发布时间: 2024-09-14 12:32:26 阅读量: 29 订阅数: 31 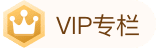
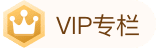
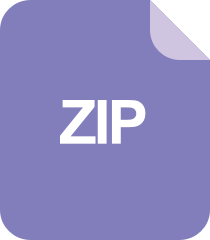
hogmatlab源码-Matlab_ToolBoxes:Matlab_ToolBoxes
# 1. Overview of MATLAB Toolboxes
MATLAB Toolboxes are a powerful collection of specialized algorithms and tools that extend the core functionality of MATLAB for specific domains. Developed and maintained by MathWorks, these toolboxes offer customized solutions tailored for various application areas.
The advantage of MATLAB Toolboxes lies in their provision of pre-built functions, algorithms, and graphical user interfaces (GUIs), enabling engineers and scientists to efficiently tackle complex problems. These toolboxes are optimized to leverage MATLAB's high-performance computing capabilities, ensuring rapid and accurate computation. Additionally, MATLAB Toolboxes include extensive documentation and examples, making them accessible to both newcomers and experienced users.
# 2. Applications of MATLAB Toolboxes in Scientific Computing
MATLAB Toolboxes play a crucial role in the field of scientific computing, providing researchers and engineers with powerful tools to solve complex problems and advance scientific discoveries. This section will explore the applications of MATLAB Toolboxes in numerical computation, linear algebra, optimization, and modeling.
### 2.1 Numerical Computation and Linear Algebra
#### 2.1.1 Matrix Operations and Solving Equations
MATLAB Toolboxes offer a wide range of functions for executing matrix operations and solving systems of equations. These functions include:
- **Matrix creation and manipulation:** `zeros()`, `ones()`, `eye()`, `rand()`, `svd()`, `eig()`
- **Solving linear systems:** `solve()`, `lu()`, `qr()`, `svd()`
- **Matrix decomposition:** `svd()`, `eig()`, `chol()`, `qr()`
**Code Block:**
```matlab
% Creating a random matrix A
A = rand(5, 5);
% Solving the linear system Ax = b
b = rand(5, 1);
x = A \ b;
% Computing the singular value decomposition of matrix A
[U, S, V] = svd(A);
```
**Logical Analysis:**
* The `rand()` function creates a 5x5 random matrix `A`.
* The `solve()` function uses the matrix left division operator (`\`) to solve the linear system `Ax = b`, where `b` is a random vector.
* The `svd()` function computes the singular value decomposition of matrix `A`, returning the singular value matrix `S`, the left singular vector matrix `U`, and the right singular vector matrix `V`.
#### 2.1.2 Data Analysis and Visualization
MATLAB Toolboxes also offer functions for data analysis and visualization. These functions include:
- **Data analysis:** `mean()`, `std()`, `max()`, `min()`, `corr()`
- **Data visualization:** `plot()`, `bar()`, `hist()`, `scatter()`
**Code Block:**
```matlab
% Importing data
data = importdata('data.csv');
% Calculating the mean and standard deviation of the data
mean_data = mean(data);
std_data = std(data);
% Plotting a histogram of the data
histogram(data);
xlabel('Data Values');
ylabel('Frequency');
title('Data Histogram');
```
**Logical Analysis:**
* The `importdata()` function imports data from a CSV file.
* The `mean()` and `std()` functions compute the mean and standard deviation of the data, respectively.
* The `histogram()` function plots a histogram of the data, showing the distribution of the data.
### 2.2 Optimization and Modeling
#### 2.2.1 Nonlinear Optimization and Constrained Optimization
MATLAB Toolboxes provide functions for nonlinear and constrained optimization. These functions include:
- **Nonlinear optimization:** `fminunc()`, `fminsearch()`, `fmincon()`
- **Constrained optimization:** `linprog()`, `quadprog()`, `intlinprog()`
**Code Block:**
```matlab
% Defining the objective function
f = @(x) x^2 + sin(x);
% Performing nonlinear optimization using fminunc()
x_optimal = fminunc(f, 0);
% Defining constraint conditions
A = [1; -1];
b = [1; 0];
lb = 0;
```
0
0
相关推荐







