Optimized Use of MATLAB Toolboxes: Boosting Efficiency and Performance, Making Your Code Soar
发布时间: 2024-09-14 12:23:57 阅读量: 9 订阅数: 16 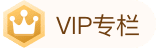
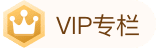
# 1. Introduction to MATLAB Toolboxes
MATLAB toolboxes are a suite of powerful extensions that provide a range of specialized functions for the MATLAB platform. These toolboxes span a wide array of disciplines, including image processing, data analysis, scientific computing, parallel computing, and artificial intelligence.
MATLAB toolboxes simplify the execution of complex tasks by offering pre-built functions, classes, and algorithms. They enable researchers, engineers, and scientists to concentrate on their core problems without spending excessive time on low-level implementation details.
The modular design of the toolboxes allows users to select and combine functionalities as needed, thus creating customized solutions to meet the requirements of specific applications.
# 2. Optimization Techniques for MATLAB Toolboxes
MATLAB toolboxes offer a series of optimization techniques that can significantly enhance the performance and efficiency of code. By following these techniques, users can reduce computation time, improve memory utilization, and achieve more accurate results.
### 2.1 Optimizing Code Structure and Algorithms
#### 2.1.1 Using Vectorized Operations
Vectori***pared to looping through each element individually, vectorized operations can dramatically improve performance by leveraging MATLAB's built-in optimizers.
**Code Block:**
```matlab
% Looping through each element
for i = 1:n
result(i) = x(i) + y(i);
end
% Vectorized operation
result = x + y;
```
**Logical Analysis:**
Looping through each element individually traverses arrays x and y and performs addition operation by operation. This can lead to performance issues, especially with large arrays.
Vectorized operations leverage MATLAB's built-in optimizers to perform addition operations on each element of arrays x and y simultaneously. This eliminates the overhead of looping, thus significantly improving performance.
#### 2.1.2 Avoiding Unnecessary Loops
Loops in MATLAB are unavoidable, but overuse can lead to performance degradation. Careful analysis of code can identify and eliminate unnecessary loops.
**Code Block:**
```matlab
% Unnecessary loop
for i = 1:n
if x(i) > 0
result(i) = x(i);
else
result(i) = 0;
end
end
% Avoiding unnecessary loops
result = x .* (x > 0);
```
**Logical Analysis:**
The unnecessary loop uses if-else statements to check each element of array x to see if it is greater than 0. This incurs performance overhead, particularly for large arrays.
Avoiding unnecessary loops uses MATLAB's element-wise operators (.*), which multiplies each element of x with a logical vector (x > 0). This eliminates the overhead of the loop and improves performance.
#### 2.1.3 Optimizing Data Storage and Processing
The way data is stored and processed in MATLAB can also affect performance. Efficiency can be improved by choosing appropriate data structures and optimizing data access.
**Code Block:**
```matlab
% Optimizing data storage
data = [1, 2, 3; 4, 5, 6; 7, 8, 9];
% Optimizing data access
result = data(1:end, 1:2);
```
**Logical Analysis:**
Optimizing data storage uses efficient data structures such as matrices or arrays to store data. This reduces memory consumption and increases the speed of data access.
Optimizing data access uses indexing or slicing to access specific elements or subsets of data. This can prevent unnecessary looping and data duplication, thereby improving performance.
### 2.2 Fully Utilizing MATLAB Toolboxes
MATLAB toolboxes provide a wealth of functions and features that can simplify complex tasks and enhance code efficiency. By selecting the appropriate toolboxes and fully utilizing their resources, users can significantly improve the performance of their code.
#### 2.2.1 Selecting the Right Toolbox
MATLAB offers various toolboxes, each optimized for specific domains or applications. Choosing the right toolbox can provide specialized functions and features, thus simplifying tasks and improving performance.
**Table:**
| Toolbox | Domain |
|---|---|
| Image Processing Toolbox | Image Processing |
| Computer Vision Toolbox | Computer Vision |
| Statistics and Machine Learning Toolbox | Statistics and Machine Learning |
| Optimization Toolbox | Model Fitting and Optimization |
| Partial Differential Equation Toolbox | Numerical Simulation |
| Simulink | System Modeling and Simulation |
#### 2.2.2 Exploring Functions and Features Provided by Toolboxes
Each MATLAB toolbox offers a rich set of functions and features that can perform a variety of tasks. By exploring the documentation and examples of the toolboxes, users can discover specific functions that can simplify code and enhance performance.
**Code Block:**
```matlab
% Using Image Processing Toolbox for image enhancement
image = imread('image.jpg');
enhancedImage = imadjust(image, [0.5, 1]);
```
**Logical Analysis:**
The imadjust function of the Image Processing Toolbox can easily adjust the contrast and brightness of an image. This can simplify image processing tasks and improve performance.
#### 2.2.3 Utilizing Documentation and Examples Provided by Toolboxes
MATLAB toolboxes provide comprehensive documentation and examples to help users understand the usage of functions and features. By leveraging these resources, users can quickly learn how to use the toolboxes and avoid common mistakes.
**Mermaid Flowchart:**
```mermaid
sequenceDiagram
participant User
participant MATLAB Toolbox
User->MATLAB Toolbox: Request function documentation
MATLAB Toolbox->User: Provide documentation
User->MATLAB Toolbox: Request function example
MATLAB Toolbox->User: Provide example
User->MATLAB Toolbox: Use function in code
```
# 3. Practical Applications of MATLAB Toolboxes
MATLAB toolboxes are widely used in various fields. Here are some common practical application scenarios:
**3.1 Image Processing Optimization**
**3.1.1 Using Image Processing Toolbox for Image Enhancement**
The Image Processing Toolbox provides a rich set of image processing functions that can be used for image enhancement, such as:
```matlab
% Reading an image
image = imread('image.jpg');
% Adjusting contrast and brightness
enhancedImage = imadjust(image, [0.2 0.8], []);
% Displaying the enhanced image
imshow(enhancedImage);
```
**Logical Analysis of the Code:**
* The imread function reads the image file.
* The imadjust function adjusts the contrast and brightness of the image. The first parameter is the input image, the second parameter is the adjustment range, and the third parameter is an empty array, indicating the use of default values.
* The imshow function displays the image.
**3.1.2 Using Computer Vision Toolbox for Image Recognition**
The Computer Vision Toolbox provides image recognition and analysis functions, such as:
```matlab
% Reading an image
image = imread('image.jpg');
% Creating an image detector
detector = vision.CascadeObjectDetector('FrontalFaceCART');
% Detecting faces in the image
bboxes = detector(image);
% Drawing face bounding boxes
for i = 1:size(bboxes, 1)
rectangle('Position', bboxes(i, :), 'EdgeColor', 'r', 'LineWidth', 2);
end
```
**Logical Analysis of the Code:**
* The imread function reads the image file.
* The vision.CascadeObjectDetector function creates a face detector.
* The detector function detects faces in the image and returns bounding boxes.
* A loop draws the bounding boxes of faces.
**3.2 Data Analysis Optimization**
**3.2.1 Using Statistics and Machine Learning Toolbox for Data Exploration**
The Statistics and Machine Learning Toolbox provides data exploration and analysis functions, such as:
```matlab
% Loading data
data = load('data.mat');
% Calculating data statistics
stats = grpstats(data, 'group', {'mean', 'std', 'min', 'max'});
% Creating a histogram
histogram(data.value, 10);
```
**Logical Analysis of the Code:**
* The load function loads the data file.
* The grpstats function calculates data statistics based on groups.
* The histogram function creates a histogram.
**3.2.2 Utilizing Optimization Toolbox for Model Fitting**
The Optimization Toolbox provides model fitting and optimization functions, such as:
```matlab
% Defining the objective function
objective = @(x) sum((x - data).^2);
% Setting optimization options
options = optimset('Display', 'iter', 'PlotFcns', @optimplotfval);
% Optimizing model parameters
x = fminsearch(objective, initialGuess, options);
```
**Logical Analysis of the Code:**
* The objective function calculates the fitting error.
* The optimset function sets optimization options, including displaying iteration information and plotting the objective function value curve.
* The fminsearch function uses a gradient-free optimization algorithm to find the minimum value of the objective function.
**3.3 Scientific Computing Optimization**
**3.3.1 Using Partial Differential Equation Toolbox for Numerical Simulation**
The Partial Differential Equation Toolbox provides numerical simulation capabilities for partial differential equations, such as:
```matlab
% Defining a partial differential equation
pde = 'pde';
% Setting boundary conditions
bc = 'bc';
% Solving the partial differential equation
solution = pdesolve(pde, bc);
```
**Logical Analysis of the Code:**
* The pde variable defines the partial differential equation.
* The bc variable defines the boundary conditions.
* The pdesolve function solves the partial differential equation and returns the solution.
**3.3.2 Utilizing Simulink for System Modeling and Simulation**
Simulink provides system modeling and simulation capabilities, such as:
```matlab
% Creating a Simulink model
model = simulink('model.slx');
% Setting simulation parameters
simParams = simset('StopTime', '10');
% Simulating the model
sim(model, simParams);
```
**Logical Analysis of the Code:**
* The simulink function creates a Simulink model.
* The simset function sets simulation parameters, including simulation time.
* The sim function simulates the model.
# 4. Advanced Applications of MATLAB Toolboxes
### 4.1 Parallel Computing
#### 4.1.1 Using Parallel Computing Toolbox for Parallel Programming
Parallel computing involves distributing computational tasks across multiple processors or computers to improve performance. The MATLAB Parallel Computing Toolbox provides a set of functions and tools for creating and managing parallel programs.
**Code Block:**
```matlab
% Creating a parallel pool
parpool(4); % Creating a parallel pool with 4 worker processes
% Defining a parallel task
parfor i = 1:100
% Executing the parallel task
result(i) = heavy_computation(i);
end
% Destroying the parallel pool
delete(gcp); % Destroying the parallel pool
```
**Logical Analysis:**
* The parpool function creates a parallel pool, specifying the number of worker processes to use.
* The parfor loop is used for parallel execution of tasks. It distributes loop iterations to worker processes in the parallel pool.
* The heavy_computation function represents the parallel task to be executed.
* The delete(gcp) function destroys the parallel pool, releasing the resources used.
**Parameter Explanation:**
* `parpool(numWorkers)`: Creates a parallel pool with `numWorkers` worker processes.
* `parfor`: Parallel execution of loop iterations.
* `gcp`: Gets the current parallel pool object.
#### 4.1.2 Optimizing the Performance of Parallel Code
Optimizing the performance of parallel code is crucial to fully utilize the advantages of parallel computing. Here are some optimization tips:
***Reduce communication between tasks:** Minimize data exchange between parallel tasks, as communication incurs overhead.
***Balance task loads:** Ensure that parallel tasks have similar computational workloads to avoid load imbalance.
***Use parallel algorithms:** Choose specially designed parallel algorithms, such as vectorized operations and parallel loops.
***Use appropriate parallelization strategies:** Depending on the nature of the task, choose appropriate parallelization strategies, such as OpenMP or MPI.
### 4.2 Cloud Computing
#### 4.2.1 Using Cloud Computing Toolbox to Connect to Cloud Platforms
The MATLAB Cloud Computing Toolbox provides tools for connecting to cloud platforms, such as Amazon Web Services (AWS) and Microsoft Azure. This allows you to access cloud computing resources, such as compute instances, storage, and databases.
**Code Block:**
```matlab
% Creating an AWS EC2 instance
instance = createInstance('t2.micro', 'ami-id', 'key-name');
% Connecting to the instance
ssh(instance);
% Executing commands on the instance
system('ls -l');
% Terminating the instance
terminateInstance(instance);
```
**Logical Analysis:**
* The createInstance function creates an AWS EC2 instance, specifying the instance type, AMI ID, and key name.
* The ssh function connects to the instance, allowing you to execute commands on the remote computer.
* The system function executes a specified command on the instance.
* The terminateInstance function terminates the instance, releasing the resources used.
**Parameter Explanation:**
* `createInstance(instanceType, amiId, keyName)`: Creates an AWS EC2 instance of the specified type.
* `ssh(instance)`: Connects to an AWS EC2 instance.
* `system(command)`: Executes a specified command on the remote computer.
* `terminateInstance(instance)`: Terminates an AWS EC2 instance.
#### 4.2.2 Utilizing Cloud Computing Resources for Large-Scale Computations
Cloud computing offers scalable computing resources that can be used for large-scale computational tasks. By leveraging cloud computing resources, you can process large datasets, run complex simulations, and accelerate development processes.
***Elastic Computing:** Cloud computing allows you to dynamically scale computational resources up or down as needed.
***High-Performance Computing:** Cloud platforms offer high-performance computing (HPC) instances with powerful computational capabilities and parallel processing features.
***Storage and Databases:** Cloud computing provides scalable storage and database services for storing and managing large datasets.
### 4.3 Artificial Intelligence
#### 4.3.1 Using Deep Learning Toolbox for Deep Learning
The MATLAB Deep Learning Toolbox provides tools and functions for building and training deep learning models. Deep learning is a form of artificial intelligence used for processing complex data, such as images, text, and speech.
**Code Block:**
```matlab
% Creating a deep neural network
layers = [
imageInputLayer([224 224 3])
convolution2dLayer(3, 32, 'Padding', 'same')
reluLayer
maxPooling2dLayer(2, 'Stride', 2)
...
fullyConnectedLayer(10)
softmaxLayer
classificationLayer
];
% Training the neural network
net = trainNetwork(trainData, layers);
% Evaluating the neural network
predictions = classify(net, testData);
```
**Logical Analysis:**
* The `imageInputLayer` defines the size and number of channels of the input image.
* The `convolution2dLayer` creates a convolutional layer for extracting image features.
* The `reluLayer` applies the ReLU activation function.
* The `maxPooling2dLayer` performs max pooling to reduce the size of the feature maps.
* The `fullyConnectedLayer` creates a fully connected layer for classification.
* The `softmaxLayer` applies the softmax function to compute the probabilities of the classes.
* The `classificationLayer` defines the classification loss function.
* The `trainNetwork` function trains the neural network.
* The `classify` function classifies new data using the trained network.
**Parameter Explanation:**
* `imageInputLayer(inputSize)`: Creates an input image layer.
* `convolution2dLayer(filterSize, numFilters, 'Padding', 'same')`: Creates a convolutional layer.
* `reluLayer`: Applies the ReLU activation function.
* `maxPooling2dLayer(poolSize, 'Stride', stride)`: Performs max pooling.
* `fullyConnectedLayer(numClasses)`: Creates a fully connected layer.
* `softmaxLayer`: Applies the softmax function.
* `classificationLayer`: Defines the classification loss function.
* `trainNetwork(data, layers)`: Trains the neural network.
* `classify(net, data)`: Classifies new data using the trained network.
#### 4.3.2 Utilizing Machine Learning Toolbox for Machine Learning
The MATLAB Machine Learning Toolbox provides tools and functions for building and training various machine learning models. Machine learning is a form of artificial intelligence used for learning patterns from data and making predictions.
**Code Block:**
```matlab
% Loading data
data = load('data.mat');
% Creating a decision tree model
tree = fitctree(data.features, data.labels);
% Predicting new data
predictions = predict(tree, newData.features);
```
**Logical Analysis:**
* The `load` function loads the data file.
* The `fitctree` function creates a decision tree model, trained using feature data and label data.
* The `predict` function predicts using the trained model on new data.
**Parameter Explanation:**
* `load(filename)`: Loads the data file.
* `fitctree(features, labels)`: Creates a decision tree model.
* `predict(tree, features)`: Predicts using the trained model on new data.
# 5. Debugging and Maintenance of MATLAB Toolboxes
### 5.1 Debugging Techniques
Debugging is the process of identifying and fixing errors in code. MATLAB provides various tools and techniques to assist in debugging code.
**5.1.1 Using Debuggers and Breakpoints**
The MATLAB debugger allows you to execute code line by line, examine variable values, and set breakpoints. A breakpoint is a location in the code where execution pauses when it reaches that point. This allows you to examine variable values and determine the source of errors.
To use the debugger, use the `debug` command or click the debug button in the editor. Then, you can use the `step`, `next`, and `continue` commands to execute the code line by line. To set a breakpoint, click next to the line number in the code.
**5.1.2 Analyzing Error Messages and Log Files**
MATLAB generates error messages and log files to help you identify and fix errors. Error messages usually provide detailed information about the cause of the error. Log files contain detailed information about the execution of the code, which can help you trace the occurrence of errors.
To view error messages, use the `lasterror` command. To view log files, use the `diary` command.
### 5.2 Maintenance Strategies
Maintenance is the process of keeping code healthy and efficient. MATLAB provides various tools and techniques to help maintain code.
**5.2.1 Version Control and Code Management**
Version control systems (e.g., Git) allow you to track changes to your code and easily revert to previous versions. This is very useful for collaborative projects and avoiding errors.
To use version control, use the `git` command or install third-party version control tools.
**5.2.2 Performance Monitoring and Optimization**
Performance monitoring tools (e.g., MATLAB Profiler) can help you identify performance bottlenecks in your code. This allows you to optimize your code to improve its speed and efficiency.
To use MATLAB Profiler, use the `profile` command or click the analyze button in the editor. The Profiler will generate a report containing detailed information about your code's performance.
# 6. Future Prospects of MATLAB Toolboxes
### 6.1 Emerging Technologies and Trends
MATLAB toolboxes will continue to keep pace with emerging technologies and trends to meet the ever-changing industry needs. Some areas of interest include:
- **Artificial Intelligence (AI)**: MATLAB has robust tools in the AI field, including deep learning and machine learning functionalities. As AI applications continue to grow, MATLAB toolboxes will continue to expand their AI capabilities to support more complex models and algorithms.
- **Cloud Computing**: Cloud computing platforms offer scalability and computational power, and MATLAB toolboxes support seamless integration with cloud platforms through the Cloud Computing Toolbox. In the future, MATLAB toolboxes will further optimize cloud computing integration to meet the needs of large-scale computations.
- **Internet of Things (IoT)**: MATLAB toolboxes provide tools for connecting and analyzing IoT device data. As IoT applications become widespread, MATLAB toolboxes will expand their IoT capabilities to support more devices and protocols.
### 6.2 Development Directions of MATLAB Toolboxes
The development direction of MATLAB toolboxes will be driven by the following factors:
- **User Feedback**: MATLAB collects user feedback to identify areas that need improvement and enhancement. User feedback is crucial for shaping the future of MATLAB toolboxes.
- **Technological Advancements**: As underlying technologies progress, MATLAB toolboxes will integrate these advancements to offer more powerful functionalities and performance.
- **Industry Trends**: MATLAB toolboxes will continue to align with industry trends to meet evolving industry needs.
### 6.3 Contributions and Su***
***munity members contribute code, examples, and documentation to support the toolboxes. The MATLAB team actively engages with the community, collecting feedback and providing support. The contributions and support from the community ensure the continuous innovation and improvement of MATLAB toolboxes.
0
0