Unveiling the Secrets of MATLAB Legends: Creating, Modifying, and Deleting for More Flexibility
发布时间: 2024-09-15 05:08:11 阅读量: 38 订阅数: 32 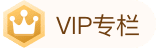
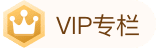
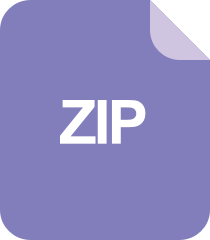
《COMSOL顺层钻孔瓦斯抽采实践案例分析与技术探讨》,COMSOL模拟技术在顺层钻孔瓦斯抽采案例中的应用研究与实践,comsol顺层钻孔瓦斯抽采案例 ,comsol;顺层钻孔;瓦斯抽采;案例,COM
# Demystifying MATLAB Legends: Creation, Customization, and Deletion for Flexibility
## 1. Overview of MATLAB Legends
A MATLAB legend is a graphical element that identifies the meaning of different data series or data points in a chart. It typically appears next to the chart, containing a series of small squares or symbols, each corresponding to a data series in the chart. Legends help the audience understand the meaning of the data in the chart and easily distinguish between different data series.
## 2. Creating and Customizing Legends
### 2.1 Ways to Create a Legend
#### 2.1.1 Using the `legend` Function
The most common method to create a legend in MATLAB is by using the `legend` function. The `legend` function accepts multiple input arguments, including handles to lines, points, or bar charts to display in the legend, and labels for the legend items.
```matlab
% Create a chart with three lines
figure;
plot(1:10, rand(1, 10), 'r-', 'LineWidth', 2);
hold on;
plot(1:10, rand(1, 10), 'b--', 'LineWidth', 2);
plot(1:10, rand(1, 10), 'g:', 'LineWidth', 2);
% Create the legend
legend('Red Solid Line', 'Blue Dashed Line', 'Green Dotted Line');
```
* `linespec`: Handles to the lines, points, or bar charts to display in the legend.
* `labels`: Labels for the legend items.
#### 2.1.2 Using the `legendbox` Function
The `legendbox` function offers a more advanced method for creating legends. It allows you to specify attributes such as the position, size, title, and font of the legend.
```matlab
% Create a legend box
figure;
plot(1:10, rand(1, 10));
legendbox('off'); % Turn off the default legend
% Create a legend using the legendbox function
legendbox('Position', [0.75, 0.75, 0.2, 0.2], ...
'String', {'Red Solid Line'}, ...
'FontSize', 12, ...
```
## 3. Associating Legends with Data
### 3.1 Correspondence Between Legend Items and Data Points
There is a one-to-one correspondence between legend items and data points, meaning each legend item corresponds to one or more data points in the chart. This correspondence can be controlled by setting the HandleVisibility property of the legend items, which has the following options:
- `off`: Hide the legend item; no data points are displayed.
- `on`: Display the legend item and all data points associated with it.
- `callback`: Display the legend item, but only show associated data points when the mouse hovers over the legend item.
### 3.2 Dynamic Update of Legends
MATLAB provides functionality to dynamically update legends, allowing the legend to automatically update when changes occur in the chart data. This can be achieved using the `legend('update')` function, which recreates the legend based on the current chart data.
```
% Create a line chart
x = 1:10;
y = rand(1, 10);
figure;
plot(x, y, 'b-o');
legend('Data');
% Update the data
y = rand(1, 10);
plot(x, y, 'r-o');
% Dynamically update the legend
legend('update');
```
### 3.3 Interactive Features of Legends
#### 3.3.1 Click and Double-Click Events on Legend Items
MATLAB supports click and double-click events on legend items, which can be implemented by setting the ButtonDownFcn and DoubleButtonDownFcn properties of the legend items. These event handlers can perform various actions, such as:
- Displaying or hiding data points associated with the legend item.
- Changing the style or color of the legend item.
- Opening a dialog box displaying detailed information about the data associated with the legend item.
```
% Create a bar chart
x = categorical({'A', 'B', 'C', 'D'});
y = [10, 20, 30, 40];
figure;
bar(x, y);
legend('Data');
% Set the click event handler for legend items
legend_items = findobj(gca, 'Type', 'Legend');
set(legend_items, 'ButtonDownFcn', @legend_item_click);
% Legend item click event handler
function legend_item_click(hObject, eventdata)
% Get the label of the legend item
item_label = get(hObject, 'String');
% Find associated data points based on the label
data_points = findobj(gca, 'Type', 'Bar', 'DisplayName', item_label);
% Display or hide associated data points
if strcmp(get(data_points, 'Visible'), 'on')
set(data_points, 'Visible', 'off');
else
set(data_points, 'Visible', 'on');
end
end
```
#### 3.3.2 Dragging and Sorting of Legend Items
MATLAB also supports dragging and sorting of legend items, which can be achieved by setting the DragBehavior property of the legend items. This property has the following options:
- `off`: Disable dragging and sorting of legend items.
- `drag`: Allow dragging of legend items, but not sorting.
- `sort`: Allow both dragging and sorting of legend items.
```
% Create a scatter plot
x = rand(100, 1);
y = rand(100, 1);
figure;
scatter(x, y);
legend('Data');
% Set the dragging and sorting behavior of legend items
legend_items = findobj(gca, 'Type', 'Legend');
set(legend_items, 'DragBehavior', 'sort');
```
# 4. Applications of Legends in Different Chart Types
The legend is an essential element in MATLAB that helps users understand the meaning of data in charts. Depending on the chart type, the display and functionality of the legend can vary. This section will introduce the application of legends in line charts, bar charts, scatter plots, pie charts, 3D plots, and polar plots.
### 4.1 Legends in Line Charts and Bar Charts
In line charts and bar charts, legends typically display the color and label for each line or bar. This helps users distinguish between different data series.
**Code Example:**
```
% Create a line chart
figure;
plot(1:10, rand(1, 10), 'b-', 1:10, rand(1, 10), 'r--');
legend('Data Series 1', 'Data Series 2');
% Create a bar chart
figure;
bar(1:10, rand(1, 10));
legend('Data Series');
```
**Logical Analysis:**
* The `legend` function is used to create legends. It takes two arguments: the labels for legend items and the line style or color of the legend items.
* In the line chart, `'b-'` represents a blue solid line, and `'r--'` represents a red dashed line.
* In the bar chart, `'Data Series'` represents the label for the legend item.
### 4.2 Legends in Scatter Plots and Pie Charts
In scatter plots and pie charts, legends usually display different shapes or colors representing different data points or groups of data.
**Code Example:**
```
% Create a scatter plot
figure;
scatter(rand(100, 1), rand(100, 1), 50, rand(100, 1));
legend('Data Points');
% Create a pie chart
figure;
pie(rand(1, 5));
legend('Data Group 1', 'Data Group 2', 'Data Group 3', 'Data Group 4', 'Data Group 5');
```
**Logical Analysis:**
* In the scatter plot, the `scatter` function takes three arguments: data point coordinates, marker size, and marker color.
* The first argument of the `legend` function specifies the labels for the legend items, and the second argument specifies the marker shape and color of the legend items.
* In the pie chart, the `pie` function takes one argument: the proportions of data groups.
* The first argument of the `legend` function specifies the labels for the legend items, and the second argument specifies the color of the legend items.
### 4.3 Legends in 3D and Polar Plots
In 3D and polar plots, legends can display different colors of surfaces or line styles, representing different data series or data points.
**Code Example:**
```
% Create a 3D plot
figure;
surf(peaks(10));
legend('Surface');
% Create a polar plot
figure;
polar(linspace(0, 2*pi, 100), rand(1, 100));
legend('Polar Line');
```
**Logical Analysis:**
* In the 3D plot, the `surf` function takes one argument: the data for the 3D surface.
* The first argument of the `legend` function specifies the label for the legend item, and the second argument specifies the color of the legend item.
* In the polar plot, the `polar` function takes two arguments: angle and radius.
* The first argument of the `legend` function specifies the label for the legend item, and the second argument specifies the line style and color of the legend item.
Legends make it easy for users to understand the meaning of data in different chart types. The display and functionality of the legend can be customized according to the chart type to meet specific visualization requirements.
# 5. Best Practices for Legends
### 5.1 Clarity and Readability of Legends
The primary purpose of a legend is to provide additional information about the data in a chart, so its clarity and readability are crucial. Here are some best practices:
***Use short and descriptive labels:** Avoid using lengthy or ambiguous labels; instead, use labels that concisely and accurately describe the data they represent.
***Ensure font size and color are easy to read:** Choose font colors that contrast well with the chart background and use sufficiently large font sizes for easy reading on various screen sizes.
***Avoid overcrowding:** If the legend contains many items, consider organizing them with a multi-column or multi-row layout to improve readability.
***Use color and shape to distinguish:** Differentiate data series using various colors and shapes, which helps to quickly identify and compare data.
***Sort legend items:** Sort legend items in ascending or descending order to facilitate users' search for specific data series.
### 5.2 Position and Layout of Legends
The position and layout of the legend are vital for the overall readability and aesthetics of the chart. Here are some best practices:
***Choose an appropriate location:** Legends are typically placed above, below, to the left, or to the right of the chart. Choose a position that does not obscure important data or interfere with the interpretation of the chart.
***Adjust size and shape:** Customize the size and shape of the legend according to the number of items and the size of the chart. Avoid making the legend too large or too small to maintain the overall balance of the chart.
***Use layout options:** MATLAB offers various layout options, such as horizontal, vertical, or compact layout. Choose the one that best suits the chart layout and the amount of data.
***Consider the shape of the legend:** Legends can be rectangular, circular, or other custom shapes. Choose a shape that matches the overall design of the chart.
### 5.3 Harmonization of Legends with the Chart
Legends should be harmonious with the overall chart to enhance its readability and aesthetics. Here are some best practices:
***Match the chart theme:** The style and color of the legend should match the chart theme to create a consistent appearance.
***Add background color:** Give the legend a background color to make it stand out from the chart background and improve readability.
***Use borders and shadows:** Adding borders or shadows can differentiate the legend from the rest of the chart, enhancing its visual appeal.
***Consider the chart type:** Different chart types may require different legend layouts and styles. For example, bar charts might need vertical legends, while scatter plots might need horizontal legends.
# 6. Troubleshooting MATLAB Legends
### 6.1 Legends Not Displaying or Displaying Incorrectly
**Causes:**
- Legends not created or added correctly.
- Legend properties (such as position or size) are improperly set.
- Legend items not correctly associated with data points.
- Chart type does not support legends.
**Solutions:**
- Check the legend creation code for correctness and ensure the use of `legend` or `legendbox` functions.
- Adjust the position and size of the legend to make it visible in the chart.
- Verify the association between legend items and data points to ensure each legend item corresponds to a data point.
- Confirm that the chart type supports legends, as line charts and pie charts do not display legends by default.
### 6.2 Legend Items Not Corresponding to Data Points
**Causes:**
- The number of data points does not match the number of legend items.
- The order of data points does not match the order of legend items.
- Information about the association between legend items and data points is lost or corrupted.
**Solutions:**
- Check if the number of data points equals the number of legend items.
- Rearrange data points or legend items to align their order.
- Try using the `'AutoUpdate'`, `'off'` options of the `legend` function to prevent automatic updates and manually update the association information for legends.
### 6.3 Inoperative Legend Interaction Features
**Causes:**
- Legend interaction features not enabled.
- Legend interaction event handlers not properly defined.
- Chart or legend objects damaged or unavailable.
**Solutions:**
- Ensure that legend interaction features are enabled, for example, by setting the `'Enable'`, `'on'` property.
- Check if legend interaction event handlers are properly defined, such as click or double-click events.
- Attempt to recreate the chart or legend objects to resolve any potential damage or availability issues.
0
0
相关推荐





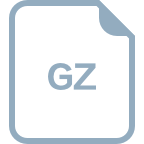
