"MATLAB Legend Drawing Secret Techniques": 10 Steps from Beginner to Expert, Creating Eye-Catching Legends
发布时间: 2024-09-15 05:05:57 阅读量: 35 订阅数: 32 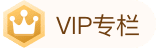
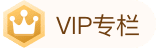
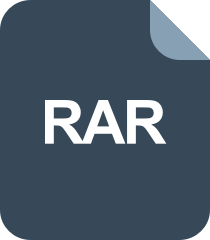
Python: The Ultimate Python Quickstart Guide - From Beginner To Expert [2016]
# The Secret Guide to Drawing Legends in MATLAB: Mastering the Art in 10 Steps
## 1. The Basics of Legends in MATLAB
A legend in MATLAB is a graphical element that explains the meaning of different lines, markers, or patches in a plot. It provides essential information about the data series, such as their names, colors, and line styles.
### Legend Location
By default, the legend is positioned in the top right corner of the plot. However, you can use the 'Location' parameter of the `legend` function to change its location. For example:
```matlab
% Place the legend in the top left corner
legend('Location', 'NorthWest');
```
## 2. Tips for Customizing Legends
The legend is a tool in MATLAB used to explain the elements of different lines, markers, or patches in a graph. It provides information about the data sources and graphic attributes, thereby enhancing the readability and understanding of the graph. This section will delve into techniques for customizing legends, including adjustments to position, size, content, and appearance.
### 2.1 Legend Position and Size
#### 2.1.1 Setting Legend Position
The position of the legend can be set using the `'Location'` parameter of the `legend` function. MATLAB offers several predefined location options, including:
| Position | Description |
|---|---|
| `'best'` | Automatically selects the best position |
| `'north'` | Top of the plot |
| `'south'` | Bottom of the plot |
| `'east'` | Right side of the plot |
| `'west'` | Left side of the plot |
| `'northwest'` | Top-left corner of the plot |
| `'northeast'` | Top-right corner of the plot |
| `'southwest'` | Bottom-left corner of the plot |
| `'southeast'` | Bottom-right corner of the plot |
```
% Create a plot
figure;
plot(1:10, rand(10, 1), 'b-', 1:10, rand(10, 1), 'r--');
% Set the legend position in the top right corner of the plot
legend('Blue Solid Line', 'Red Dashed Line', 'Location', 'northeast');
```
#### 2.1.2 Adjusting Legend Size
The size of the legend can be adjusted using the `'Position'` parameter of the `legend` function. This parameter accepts a four-element vector representing the x-coordinate of the lower-left corner, the y-coordinate of the lower-left corner, the width, and the height of the legend.
```
% Create a plot
figure;
plot(1:10, rand(10, 1), 'b-', 1:10, rand(10, 1), 'r--');
% Set the legend position and size
legend('Blue Solid Line', 'Red Dashed Line', 'Location', 'northeast', ...
'Position', [0.75, 0.75, 0.2, 0.2]);
```
### 2.2 Customizing Legend Content
#### 2.2.1 Modifying Legend Text
The text in the legend can be modified using the `'String'` parameter of the `legend` function. This parameter accepts an array of strings, with each string corresponding to the text of a legend item.
```
% Create a plot
figure;
plot(1:10, rand(10, 1), 'b-', 1:10, rand(10, 1), 'r--');
% Modify the legend text
legend('Blue Data', 'Red Data');
```
#### 2.2.2 Adding Legend Markers
Markers such as lines, markers, or patches can be added to the legend to represent data more intuitively. Markers can be added using the `'Marker'` and `'LineStyle'` parameters of the `legend` function.
```
% Create a plot
figure;
plot(1:10, rand(10, 1), 'b-', 1:10, rand(10, 1), 'r--', 1:10, rand(10, 1), 'g*');
% Add legend markers
legend('Blue Solid Line', 'Red Dashed Line', 'Green Stars', ...
'Marker', {'none', 'none', '*'}, ...
'LineStyle', {'-', '--', 'none'});
```
### 2.3 Optimizing Legend Appearance
#### 2.3.1 Setting the Legend Background Color
The background color of the legend can be set using the `'Color'` parameter of the `legend` function. This parameter accepts a color value, which can be a string (like `'white'`, `'blue'`) or an RGB value (like `[1, 0, 0]`).
```
% Create a plot
figure;
plot(1:10, rand(10, 1), 'b-', 1:10, rand(10, 1), 'r--');
% Set the legend background color
legend('Blue Solid Line', 'Red Dashed Line', 'Color', 'white');
```
#### 2.3.2 Adjusting the Legend Border
The legend's border can be adjusted using the `'Box'` parameter of the `legend` function. This parameter accepts a string, which can be `'on'` (show border) or `'off'` (hide border).
```
% Create a plot
figure;
plot(1:10, rand(10, 1), 'b-', 1:10, rand(10, 1), 'r--');
% Hide the legend border
legend('Blue Solid Line', 'Red Dashed Line', 'Box', 'off');
```
# 3.1 Legend Click Events
#### 3.1.1 Responding to Legend Clicks
MATLAB provides the `'SelectionChangeFcn'` property of the `legend` function, which allows users to specify a click event handler function for legend items. When a user clicks on a legend item, this function will be triggered.
**Code Block:**
```
% Create a legend
legend('Data 1', 'Data 2', 'Data 3');
% Set the legend click event handler function
legend('SelectionChangeFcn', @legendClickCallback);
% Legend click event handler function
function legendClickCallback(~, event)
% Get the clicked legend item
clickedItem = event.Target;
% Get the label of the clicked legend item
clickedItemLabel = clickedItem.String;
% Perform corresponding actions based on the clicked legend item label
switch clickedItemLabel
case 'Data 1'
% Perform operations on data 1
case 'Data 2'
% Perform operations on data 2
case 'Data 3'
% Perform operations on data 3
end
end
```
**Logical Analysis:**
* `legend('SelectionChangeFcn', @legendClickCallback)` sets the `'SelectionChangeFcn'` property of the legend to the `legendClickCallback` function, which will be triggered when a user clicks on a legend item.
* `function legendClickCallback(~, event)` is the legend click event handler function, which takes two parameters: `~` (unused) and `event` (the event object).
* `clickedItem = event.Target` gets the clicked legend item.
* `clickedItemLabel = clickedItem.String` gets the label of the clicked legend item.
* `switch clickedItemLabel` performs corresponding actions based on the clicked legend item label.
#### 3.1.2 Hiding/Showing Legend Items
By responding to legend click events, you can implement the functionality to hide or show legend items.
**Code Block:**
```
% Create a legend
legend('Data 1', 'Data 2', 'Data 3');
% Set the legend click event handler function
legend('SelectionChangeFcn', @legendClickCallback);
% Legend click event handler function
function legendClickCallback(~, event)
% Get the clicked legend item
clickedItem = event.Target;
% Get the label of the clicked legend item
clickedItemLabel = clickedItem.String;
% Hide or show the legend item
switch clickedItemLabel
case 'Data 1'
% Hide data 1
plot1.Visible = 'off';
case 'Data 2'
% Hide data 2
plot2.Visible = 'off';
case 'Data 3'
% Hide data 3
plot3.Visible = 'off';
end
end
```
**Logical Analysis:**
* `plot1.Visible = 'off'`, `plot2.Visible = 'off'`, and `plot3.Visible = 'off'` hide the corresponding data plot objects.
# 4. Advanced Applications of Legends
### 4.1 Sub-legends in Legends
#### 4.1.1 Creating Sub-legends
In MATLAB, sub-legends can be created using the `subplot` function. A sub-legend is a smaller legend nested within the main legend, used to further subdivide a specific group of data in the main legend.
The syntax for creating a sub-legend is as follows:
```matlab
subplot(m, n, p)
```
Where:
* `m`: The row number where the sub-legend is located within the main legend
* `n`: The column number where the sub-legend is located within the main legend
* `p`: The position of the sub-legend within the main legend
For example, the following code creates a sub-legend located in the first row, first column, position 1, within the main legend:
```matlab
subplot(1, 1, 1)
```
#### 4.1.2 Customizing the Appearance of Sub-legends
Similar to the main legend, the appearance of a sub-legend can also be customized. The `legend` function's `'SubLegend'` option can be used to set the attributes of the sub-legend.
For example, the following code sets the background color of the sub-legend to green:
```matlab
legend('SubLegend', 'BackgroundColor', 'green')
```
### 4.2 Legends Associated with Data
#### 4.2.1 Dynamically Updating Legends Based on Data
MATLAB provides the `legend('update')` function, which can dynamically update the legend based on changes in the data. When the data is updated, the legend will automatically update to reflect the new data.
For example, the following code creates a plot and uses `legend('update')` to dynamically update the legend:
```matlab
x = 1:10;
y = rand(1, 10);
plot(x, y)
legend('Data')
while true
y = rand(1, 10);
plot(x, y)
legend('update')
pause(0.1)
end
```
#### 4.2.2 Using Legends to Control Data Display
Legends can also be used to control the display of data in a plot. By clicking on items in the legend, the corresponding data groups can be shown or hidden.
For example, the following code creates a plot and uses the legend to control data display:
```matlab
x = 1:10;
y1 = rand(1, 10);
y2 = rand(1, 10);
plot(x, y1, 'r', x, y2, 'b')
legend('Data1', 'Data2')
while true
choice = input('Enter 1 to show Data1, 2 to show Data2, or 0 to exit: ');
switch choice
case 1
set(gca, 'Visible', 'on')
set(findobj(gca, 'Tag', 'legend'), 'Visible', 'on')
set(findobj(gca, 'Type', 'line', 'Color', 'blue'), 'Visible', 'off')
case 2
set(gca, 'Visible', 'on')
set(findobj(gca, 'Tag', 'legend'), 'Visible', 'on')
set(findobj(gca, 'Type', 'line', 'Color', 'red'), 'Visible', 'off')
case 0
break
end
end
```
# 5.1 Design Principles of Legends
### 5.1.1 Clarity and Simplicity
The design of legends should follow the principles of clarity and simplicity to ensure users can quickly understand the information contained within the legend. Here are some specific suggestions:
- **Use clear text:** The text in the legend should be concise and clearly describe the data or function it represents. Avoid ambiguous or unclear language.
- **Maintain consistency:** Text, markers, and colors in the legend should be consistent throughout the entire graphic. This helps users quickly identify and understand the information in the legend.
- **Logical organization:** Items in the legend should be organized in a logical order, such as by data type, color, or other relevance. This can help users easily find the information they need.
- **Avoid redundancy:** The legend should not contain duplicate or unnecessary information. Only include information that is crucial for understanding the graphic.
### 5.1.2 Aesthetic Coordination
In addition to clarity and simplicity, legends should be aesthetically pleasing and harmonize with the overall design of the graphic. Here are some suggestions for aesthetically designing legends:
- **Choose appropriate colors:** Colors in the legend should match the data or functions in the graphic. Colors should be clear and distinct, avoiding overly bright or harsh colors.
- **Adjust size and position:** The size and position of the legend should be coordinated with the overall layout of the graphic. The legend should be large enough for users to read comfortably but should not occupy too much space in the graphic.
- **Use appropriate fonts:** The font in the legend should be clear and easy to read. Avoid using overly fancy or hard-to-read fonts.
- **Maintain consistency:** Design elements in the legend, such as fonts, colors, and layout, should be consistent with other elements in the graphic. This helps create a consistent and aesthetically pleasing overall design.
# 6.1 Complex Data Visualization
### 6.1.1 Using Legends to Display Multiple Data Sets
In complex data visualization, legends play a crucial role in helping users distinguish and understand multiple different data sets. MATLAB provides several methods to use legends to display multiple data sets:
```
% Create a bar chart containing multiple data sets
data = [1, 3, 5; 2, 4, 6; 7, 8, 9];
bar(data);
% Add legend labels for each data set
legend('Group 1', 'Group 2', 'Group 3');
% Set the legend position
legend('Location', 'northeast');
```
### 6.1.2 Optimizing Legend Layout
When the legend contains a large number of items, optimizing the legend layout is crucial to ensure clarity and understandability. MATLAB offers various options to adjust the legend layout:
```
% Set legend title
legend('
0
0
相关推荐







