MATLAB Insufficient Input Parameters Unit Testing: Ensuring Code Robustness and Reliability
发布时间: 2024-09-14 14:41:24 阅读量: 24 订阅数: 22 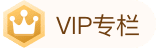
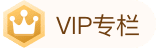
# 1. Unit Testing Overview
Unit testing is a software testing method that verifies the correctness of the smallest testable units in software (通常是 functions or methods). It is achieved by providing inputs to the unit and checking whether the output matches the expected result. Unit testing is essential for ensuring the robustness, reliability, and maintainability of the code.
The purpose of unit testing is to uncover errors in the code that might be difficult to find through manual testing. By automating the testing process, unit tests can improve test coverage and ensure that the code functions as intended under various input conditions. Additionally, unit tests help quickly identify regression issues when code changes, thus reducing maintenance costs.
# 2. Handling Input Parameters in MATLAB Unit Testing
### 2.1 The Importance of Input Parameter Validation
Input parameter validation is a critical step in unit testing, ensuring that functions operate correctly under various input conditions. Unvalidated input parameters might lead to unexpected behavior or even crashes, impacting the reliability and robustness of the code.
### 2.2 Mechanisms for Input Parameter Validation in MATLAB
MATLAB provides several mechanisms for input parameter validation, including:
#### 2.2.1 Input Parameter Check Functions
MATLAB has built-in several input parameter check functions that can be used to validate the type, size, and range of input parameters. These functions include:
- `nargin`: Checks the number of input parameters
- `isnumeric`: Checks if the input parameter is numeric
- `ischar`: Checks if the input parameter is a character
- `islogical`: Checks if the input parameter is a logical value
- `iscell`: Checks if the input parameter is a cell array
For example:
```matlab
function myFunction(x, y)
% Check if x is numeric
if ~isnumeric(x)
error('Input parameter x must be numeric');
end
% Check if y is positive
if y <= 0
error('Input parameter y must be positive');
end
end
```
#### 2.2.2 Custom Input Parameter Validation
In addition to built-in functions, custom functions can be created to validate input parameters. This is particularly useful for verifying more complex conditions or executing application-specific checks.
For example:
```matlab
function validateInput(x, y)
% Check if x is within the range [0, 1]
if x < 0 || x > 1
error('Input parameter x must be within [0, 1]');
end
% Check if y is even
if mod(y, 2) ~= 0
error('Input parameter y must be even');
end
end
```
### 2.2.3 Benefits of Using Input Parameter Validation
Using input parameter validation can offer the following benefits:
- Enhancing code robustness: Validating input parameters can prevent functions from crashing when dealing with invalid input.
- Improving code readability: Clear input parameter validation can help other developers understand the intended inputs of a function.
- Reducing debugging time: Validating input parameters in unit tests can help identify issues early, thus reducing debugging time.
# 3. Unit Testing with Insufficient Input Parameters
### 3.1 Definition and Impact of Insufficient Input Parameters
Insufficient input parameters refer to the situation where a function or method is called without one or more required parameters. This can prevent the function or method from executing properly, resulting in errors or unexpected outcomes.
The impacts of insufficient input parameters include:
- **Program crashes:** Func
0
0
相关推荐
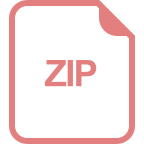
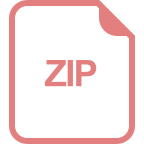
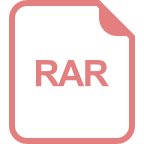
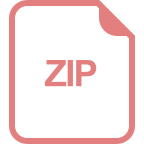