PyCharm Python Unit Testing Guide: Writing Efficient and Reliable Code
发布时间: 2024-09-14 21:43:38 阅读量: 23 订阅数: 28 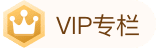
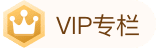
# 1. Overview of Python Unit Testing
Unit testing is a software testing technique used to verify the correctness of software components such as functions, classes, or modules. In Python, unit testing frameworks provide a simple and powerful mechanism for writing and running unit tests.
Unit tests can aid developers in:
- Improving code quality: By identifying and fixing errors, unit tests ensure the robustness and reliability of the code.
- Promoting refactoring and maintenance: Unit tests act as a safety net for code changes, ensuring that refactoring and maintenance do not break existing functionality.
- Enhancing development efficiency: By automating the testing process, unit tests save time and improve development efficiency.
# 2. PyCharm Unit Testing Tools
### 2.1 Configuring PyCharm Unit Test Environment
**Steps to configure the PyCharm unit test environment:**
1. **Install PyCharm:** Download and install the professional or community edition of PyCharm from the official website.
2. **Create a Python project:** Open PyCharm and create a new Python project.
3. **Install unittest:** Install the unittest library in the project with `pip install unittest`.
4. **Configure the unit test runner:** In PyCharm, navigate to "Preferences" > "Tools" > "Python Integrated Tools" > "Testing." Select "Unittest" as the default test runner.
5. **Create a test directory:** Create a directory named "tests" in the project to hold unit tests.
### 2.2 Creating and Running Unit Tests
**Creating Unit Tests:**
1. **Create a test *** "tests" directory, for example, "test_my_module.py."
2. **Import unittest:** In the test file, import the unittest library with `import unittest`.
3. **Create a test class:** Create a test class that inherits from unittest.TestCase, for example:
```python
import unittest
class TestMyModule(unittest.TestCase):
def test_my_function(self):
# Write test code
```
**Running Unit Tests:**
1. **Right-click on the test *** "Run 'test_my_module'."
2. **Use shortcuts:** Use the shortcut "Ctrl + Shift + F10" or "Cmd + Shift + F10" to run all tests.
3. **View test results:** Test results will be displayed in the "Run" tool window of PyCharm.
### 2.3 Analyzing Unit Test Results
**Analyzing Unit Test Results:**
1. **Passed:** The test passed with no failures or errors.
2. **Failed:** The test failed, indicating an assertion failure.
3. **Error:** An error occurred during test execution.
4. **Skipped:** The test was skipped and not executed.
5. **Expected failure:** The test was expected to fail but actually passed.
**Analyzing Failures and Errors:**
1. **View error messages:** Error messages will be shown in the "Run" tool window.
2. **Check assertions:** Failed tests typically contain failed assertions. Check the assertions to understand why the test failed.
3. **Use the debugger:** Use PyCharm's debugger to step through the test execution and identify issues.
**Code Block:**
```python
import unittest
class TestMyModule(unittest.TestCase):
def test_my_function(self):
expected_result = 10
actual_result = my_function()
self.assertEqual(expected_result, actual_result)
```
**Logical Analysis:**
This code block tests whether the `my_function()` function returns th
0
0
相关推荐
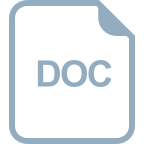
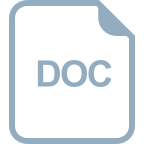
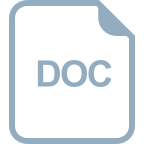
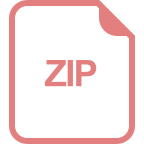
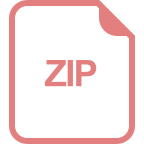
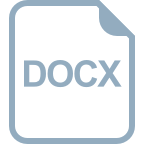
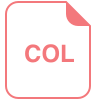
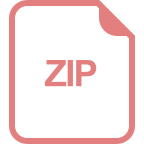