PyCharm Python Virtual Environment Guide: Isolate Development, Enhance Efficiency
发布时间: 2024-09-14 21:38:13 阅读量: 34 订阅数: 33 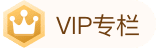
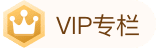
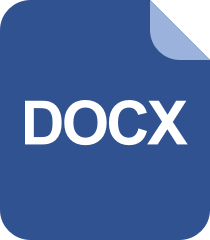
Python与PyCharm的入门到精通:安装配置全流程指南
# An Introduction to Python Virtual Environments with PyCharm: Isolation for Efficiency
## 1. Python Virtual Environment Primer
A Python virtual environment is a self-contained Python execution environment that allows developers to use different Python versions and packages across various projects without impacting the system-wide installation. Virtual environments achieve this isolation by creating separate directories that contain copies of the Python interpreter, packages, and libraries.
Virtual environments offer developers several advantages:
- **Isolated Development Environments**: Virtual environments allow developers to use different Python versions and packages for different projects without worrying about conflicts or impacting other projects.
- **Avoiding Package Conflicts**: Packages within a virtual environment are isolated from system-wide packages, preventing package conflicts and version discrepancies.
- **Enhancing Development Efficiency**: Virtual environments streamline package management by isolating development environments, thus increasing development efficiency.
## 2. Creating and Managing Virtual Environments with PyCharm
### 2.1 Creating a Virtual Environment
Creating a virtual environment in PyCharm is straightforward. Follow these steps:
1. Open PyCharm, create a new project or open an existing one.
2. Click on the "File" menu, then select "Settings".
3. In the "Settings" dialog, choose "Project" > "Python Interpreter".
4. Click the "Add" button, then choose "Virtualenv Environment".
5. In the "Create Virtual Environment" dialog, enter a name and location for the virtual environment.
6. Select the Python interpreter version to use.
7. Click "OK" to create the virtual environment.
### 2.2 Activating and Deactivating Virtual Environments
After creating a virtual environment, it needs to be activated before use. Follow these steps to activate a virtual environment:
1. In PyCharm, open the "Project" view.
2. Right-click on the project name, then select "Activate Virtualenv".
3. Choose the virtual environment you wish to activate from the list.
To deactivate a virtual environment, right-click on the project name and select "Deactivate Virtualenv".
### 2.3 Managing Packages in a Virtual Environment
Packages within a virtual environment are separate from those installed system-wide. This means packages can be installed and managed within the virtual environment without affecting system-wide installations.
To install a package, open the PyCharm "Terminal" window and use the following command:
```bash
pip install <package_name>
```
To uninstall a package, use this command:
```bash
pip uninstall <package_name>
```
PyCharm also provides a graphical user interface for package management. In the "Project" view, right-click on the project name and select "Show All Packages". This will open the "Packages" window, listing all packages installed in the virtual environment.
**Code Block: Installing the NumPy Package**
```python
# Open the PyCharm "Terminal" window
# Use the pip command to install NumPy
pip install numpy
```
**Logical Analysis:**
This code uses the pip command to install the NumPy package within the currently activated virtual environment. Pip is the package management tool for Python, used for installing, uninstalling, and managing Python packages.
**Parameter Explanation:**
***package_name:** The name of the package to be installed.
## 3.1 Isolated Development Environments
The primary and most significant advantage of virtual environments is their ability to isolate development environments. This is particularly useful in the following scenarios:
- **Working on Different Projects**: Each project can have its own virtual environment with packages and dependencies specific to the project. This prevents package conflicts and ensures each project uses the correct software versions.
- **Testing Different Package Versions**: Virtual environments allow testing of different package versions without impacting the main development environment. This is useful for evaluating new features or fixing bugs.
- **Collaborative Development**: Team members can work in their own virtual environments without worrying about package conflicts or mismatched dependency versions.
#### Isolating Packages and Dependencies
Virtual environments achieve isolation by segregating packages and dependencies into their own directories. This means each virtual environment has its own package installations, separate from other virtual environments or system-installed packages.
#### Avoiding Package Conflicts
Package conflicts occur when two or more packages require the same resources (e.g., modules or functions). In a virtual environment, each environment has its own package installations, thus avoiding conflicts.
#### Ensuring Correct Dependency Versions
Virtual environments also ensure that each project uses the correct dependency versions. This is crucial for ensuring projects run smoothly and to prevent errors caused by mismatched dependency versions.
## 4. Practical Applications of Virtual Environments
### 4.1 Creating and Managing Multiple Virtual Environments
Creating and managing multiple virtual environments in PyCharm is convenient. Follow these steps to create a new virtual environment:
1. Open PyCharm and create a new project.
2. Click on the "Python Interpreter" dropdown in the project toolbar.
3. Select the "Add" option, then choose "Virtual Environment".
4. In the "Create Virtual Environment" dialog, specify a name and location for the virtual environment.
5. Choose the desired Python interpreter version.
6. Click the "Create" button.
After creating a virtual environment, you can manage it by following these steps:
1. Select the virtual environment to manage from the "Python Interpreter" dropdown.
2. Click the "gear" icon to open the "Virtual Environment Settings" dialog.
3. In this dialog, you can view details of the virtual environment, install and uninstall packages, and perform other management tasks.
### 4.2 Installing and Managing Packages in a Virtual Environment
Installing and managing packages in a virtual environment is similar to doing so globally. Follow these steps to install a package in a virtual environment:
1. Open the project in PyCharm.
2. Ensure the virtual environment you wish to install the package in is activated.
3. Click on the "Terminal" icon in the "Project" toolbar.
4. In the terminal, use the `pip` command to install the package. For example:
```
pip install package-name
```
To uninstall a package, use this command:
```
pip uninstall package-name
```
### 4.3 Debugging and Troubleshooting in Virtual Environments
Debugging and troubleshooting in virtual environments are similar to doing so in a global environment. Follow these steps to debug code in a virtual environment:
1. Open the project in PyCharm.
2. Ensure the virtual environment for debugging is activated.
3. Set breakpoints and run the code.
4. Use debugging tools (such as step execution, variable inspection, etc.) in the "Debug" toolbar to debug the code.
If you encounter issues in a virtual environment, try the following troubleshooting steps:
1. Ensure the correct virtual environment is activated.
2. Check if the packages in the virtual environment are up-to-date.
3. Try recreating the virtual environment.
4. Check PyCharm's error logs for more information.
## 5.1 Managing Virtual Environments with pipenv
pipenv is a Python package management tool that helps you manage packages within virtual environments. Unlike pip, pipenv automatically creates and manages virtual environments and tracks the packages and dependencies you install.
### Installing pipenv
To install pipenv, run the following command:
```bash
pip install pipenv
```
### Creating a Virtual Environment
Creating a virtual environment with pipenv is simple. Just run this command:
```bash
pipenv install
```
This will create a `Pipfile` containing the configuration information for the virtual environment and a `Pipfile.lock` file containing the locked information of the installed packages.
### Installing Packages
To install packages, use this command:
```bash
pipenv install <package_name>
```
pipenv will automatically install the package and its dependencies, adding them to the `Pipfile` and `Pipfile.lock` files.
### Freezing Dependencies
To freeze the dependencies in a virtual environment, run the following command:
```bash
pipenv lock
```
This will update the `Pipfile.lock` to include the latest versions of all installed packages in the virtual environment.
### Managing Multiple Virtual Environments with pipenv
pipenv allows you to manage multiple virtual environments. To create a new virtual environment, use the following command:
```bash
pipenv --venv <virtualenv_name> install
```
To activate a virtual environment, use:
```bash
pipenv --venv <virtualenv_name> shell
```
To deactivate a virtual environment, simply use:
```bash
deactivate
```
0
0
相关推荐






