Python Interpreter Configuration Guide: Selecting and Configuring the Best Interpreter in PyCharm
发布时间: 2024-09-14 21:39:15 阅读量: 42 订阅数: 28 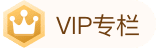
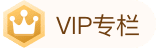
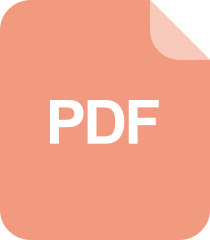
解决pycharm运行时interpreter为空的问题
# 1. An Overview of the Python Interpreter
The Python interpreter is the core component of the Python programming language, responsible for executing Python code. It translates source code into bytecode, which is then run by the virtual machine. The interpreter provides the fundamental features of the Python language, including variables, data types, control flow, and functions.
The interpreter can also be extended through modules and packages, allowing users to access a variety of libraries and tools. These libraries offer a wealth of functionality, ranging from data processing and machine learning to networking and GUI development. Understanding the capabilities of the Python interpreter is crucial for effectively utilizing the Python language.
# 2. Configuring the Python Interpreter in PyCharm
### 2.1 Selecting a Python Interpreter
In PyCharm, you can choose to use a local interpreter or an interpreter within a virtual environment.
**2.1.1 Local Interpreter**
A local interpreter is a Python interpreter installed on your computer. PyCharm automatically detects interpreters installed on your system.
**2.1.2 Virtual Environment**
A virtual environment is an isolated Python environment, separate from other interpreters on your system. This helps manage different project dependencies and prevents conflicts.
### 2.2 Configuring Interpreter Settings
**2.2.1 Interpreter Path**
Ensure that the correct interpreter path is configured in PyCharm. You can do this by following these steps:
1. Open PyCharm.
2. Go to "File" > "Settings" > "Project" > "Python Interpreter".
3. Select the interpreter you wish to use.
**2.2.2 Environment Variables**
Environment variables are used to define the interpreter path and dependencies. You can configure environment variables by following these steps:
1. Open PyCharm.
2. Go to "File" > "Settings" > "Project" > "Python Interpreter".
3. Click on the "Environment Variables" tab.
4. Add or modify environment variables.
**2.2.3 Project Interpreter**
You can specify a particular interpreter for each project. This allows you to use different Python versions or virtual environments in different projects.
1. Open PyCharm.
2. Open the project you wish to configure.
3. Go to "File" > "Settings" > "Project" > "Python Interpreter".
4. Select the interpreter you wish to use.
### Code Example: Configuring a Local Interpreter
```python
import sys
# Get the current interpreter path
interpreter_path = sys.executable
# Check if the interpreter path is correct
if interpreter_path != "/usr/bin/python3":
# Update the interpreter path
sys.executable = "/usr/bin/python3"
```
**Code Logic Analysis:**
* Import the `sys` module to access system information.
* Retrieve the current interpreter path and store it in the `interpreter_path` variable.
* Check if `interpreter_path` matches the expected interpreter path.
* If the path is incorrect, use `sys.executable` to update the interpreter path.
### Code Example: Configuring a Virtual Environment
```python
import venv
# Create a virtual environment
venv.create("my_virtual_env")
# Activate the virtual environment
venv.activate("my_virtual_env")
```
**Code Logic Analysis:**
* Import the `venv` module to create and activate virtual environments.
* Use `venv.create()` to create a virtual environment named "my_virtual_env".
* Use `venv.activate()` to activate the virtual environment.
# 3.1 Virtual Environment Management
**3.1.1 Creating and Activating Virtual Environments**
Virtual environments are Python environments independent of the system environment, allowing users to use different Python versions and packages in various projects without affecting others. To create a virtual environment, use the following command:
```python
python -m venv venv_name
```
Where `venv_name` is the name of the virtual environment you wish to create. After creating the virtual environment, activate it using the following command:
```python
source venv_name/bin/activate
```
To exit a virtual environment, use the following command:
```python
deactivate
```
**3.1.2 Managing Virtual Environment Packages**
When installing packages in a virtual environment, they are only installed in that environment and do not affect the system environment. To install a package, use the following command:
```python
pip install package_name
```
To uninstall a package, use the following command:
```python
pip uninstall package_name
```
To view the list of packages installed in a virtual environment, use the following command:
```python
pip list
```
### 3.2 Enhancing Interpreter Performance
**3.2.1 Enabling Multiprocessing**
Multiprocessing allows the Python interpreter to execute tasks using multiple CPU cores simultaneously, which can significantly improve the performance of certain types of applications. To enable multiprocessing, use the following code in your Python script:
```python
import multiprocessing
def worker(num):
print(f"Worker {num} is running")
if __name__ == "__main__":
jobs = []
for i in range(5):
p = multiprocessing.Process(target=worker, args=(i,))
jobs.append(p)
p.start()
```
In the example above, the `worker()` function will run simultaneously in 5 different processes.
**3.2.2 Using a JIT Compiler**
A JIT (Just-In-Time) compiler translates Python bytecode into machine code, thereby increasing the execution speed of the interpreter. To use a JIT compiler, use the following code in your Python script:
```python
import sys
sys.settrace(sys.gettrace() or lambda *args, **kwargs: None)
```
The JIT compiler will automatically enable and compile bytecode when needed.
# 4. Advanced Configuration of the Python Interpreter
### 4.1 Debugging the Interpreter
Debugging the interpreter is essential for finding and fixing errors in your code. PyCharm provides powerful debugging features that allow developers to step through code, inspect variable values, and identify exceptions.
#### 4.1.1 Using the pdb Debugger
pdb (Python Debugger) is a built-in module for interactive debugging. It allows developers to set breakpoints in the code and pause the program during execution.
```python
import pdb
def my_function():
pdb.set_trace() # Set a breakpoint
print("Hello, world!")
my_function()
```
When this code is executed, the program will pause at the `pdb.set_trace()` line. Developers can use pdb commands (such as `n` (next), `s` (step), `l` (list)) to inspect variable values and debug the code.
#### 4.1.2 Remote Debugging
Remote debugging allows developers to debug code on a remote machine. PyCharm supports remote debugging and can connect to a remote interpreter via SSH or a Remote Python Debugger (RPD).
**Using SSH for Remote Debugging**
1. Start an SSH server on the remote machine.
2. In PyCharm, go to "Run" > "Debug Configurations".
3. Create a new Python remote debugging configuration.
4. Enter the IP address of the remote machine in the "Host" field.
5. Enter the SSH port (usually 22) in the "Port" field.
6. Select the remote interpreter in the "Interpreter" field.
**Using RPD for Remote Debugging**
1. Install RPD on the remote machine.
2. In PyCharm, go to "Run" > "Debug Configurations".
3. Create a new Python remote debugging configuration.
4. Enter the IP address of the remote machine in the "Host" field.
5. Enter the RPD port (usually 5678) in the "Port" field.
6. Select the remote interpreter in the "Interpreter" field.
### 4.2 Extending Interpreter Capabilities
Beyond its core capabilities, the Python interpreter can be extended by installing third-party libraries and creating custom interpreters.
#### 4.2.1 Installing Third-Party Libraries
Third-party libraries offer a wide range of functionality, from data analysis to machine learning. Third-party libraries can be installed using the `pip` command.
```
pip install numpy
```
After installation, you can import the library and use its features.
```python
import numpy as np
arr = np.array([1, 2, 3])
print(arr.mean())
```
#### 4.2.2 Creating Custom Interpreters
Developers can create custom interpreters to extend the core functionality of the interpreter. This can be achieved by subclassing the `PathFinder` class within `sys.meta_path`.
```python
class MyPathFinder(sys.meta_path):
def find_module(self, fullname, path=None):
# Custom module lookup logic
# Add the custom path finder to sys.meta_path
sys.meta_path.append(MyPathFinder())
```
By creating a custom interpreter, developers can add new features, such as custom module loading or code optimization.
# 5. Best Practices for the Python Interpreter
### 5.1 Maintaining Interpreter Versions
Keeping the Python interpreter version up-to-date is crucial for ensuring security and functionality.
#### 5.1.1 Updating Python Versions
Regularly check the Python official website for the latest Python version. It is recommended to use package managers like pip or conda to update Python versions.
```
pip install --upgrade pip
pip install --upgrade python
```
#### 5.1.2 Managing Multiple Python Versions
If you need to use multiple Python versions, you can use virtual environments or containers to isolate different environments. Virtual environments allow you to install and manage multiple Python versions on the same system, while containers provide an isolated environment with all necessary dependencies.
### 5.2 Ensuring Interpreter Security
Protecting the Python interpreter from malware and security vulnerabilities is critical.
#### 5.2.1 Avoid Using Unsecure Interpreters
Do not download or install Python interpreters from unofficial sources. Always obtain interpreters from the official website or trusted repositories.
#### 5.2.2 Limiting Interpreter Permissions
Limit the permissions of the Python interpreter to prevent the execution of malicious code. Use tools like setuptools or wheel to create executables with limited permissions.
```
python setup.py build
python setup.py install --user
```
# 6. Frequently Asked Questions About the Python Interpreter
### 6.1 Interpreter Not Found
**Problem Description:**
An "interpreter not found" error occurs when running a Python script in PyCharm.
**Solution:**
**6.1.1 Check Interpreter Path**
* Open the "Settings" menu in PyCharm.
* Under "Project" > "Python Interpreter", check if the path of the selected interpreter is correct.
* If the path is incorrect, click the "Add" button and select the correct interpreter.
**6.1.2 Ensure Environment Variables Are Correct**
* Check if the PATH environment variable in the operating system includes the path to the Python interpreter.
* If not, add the interpreter path, for example:
```
Windows: PATH=%PATH%;C:\Python39
macOS/Linux: export PATH=$PATH:/usr/local/bin/python3.9
```
### 6.2 Incorrect Interpreter Version
**Problem Description:**
The interpreter version displayed in PyCharm does not match the actual installed version.
**Solution:**
**6.2.1 Update PyCharm**
* Check for any available updates for PyCharm.
* If there are any, update PyCharm and restart.
**6.2.2 Reinstall Python**
* Uninstall the currently installed Python version.
* Download and reinstall the desired Python version from the official website.
* Restart PyCharm and reconfigure the interpreter.
0
0
相关推荐
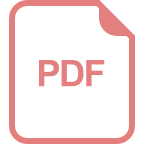
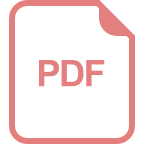
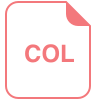
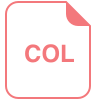
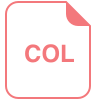
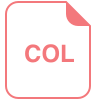
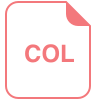
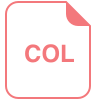
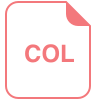